Dart If-Else-If
Dart If-Else-If statement is and extension to if-else statement. If-Else-If contains more than one condition and appears to be a ladder of if-else statements.
Syntax
The syntax of if-else-if statement in Dart is as shown in the following.
if (boolean_expression_1) {
//statement(s)
} else if (boolean_expression_2) {
//statement(s)
} else {
//else block statement(s)
}
If-Else-If ladder can contain multiple else-if blocks, but only one mandatory if block at the start and an optional else block at the end of ladder.
The execution checks the boolean expressions sequentially one by one. While evaluation if the boolean expression evaluates to true, the corresponding block of statements are executed, else, the program control goes with the evaluation of next boolean expression in the ladder. If none of the boolean expressions evaluate to true, else block is executed.
Example
In this example, we will try to check if the given number is negative, zero or positive using if-else-if block.
main.dart
import 'dart:io';
void main(){
//read number from user
print('Enter a number');
var a = double.parse(stdin.readLineSync()!);
if(a<0){
print('$a is negative number.');
} else if(a==0) {
print('$a is zero. Neither negative nor positive');
} else {
print('$a is positive number.');
}
}
Output
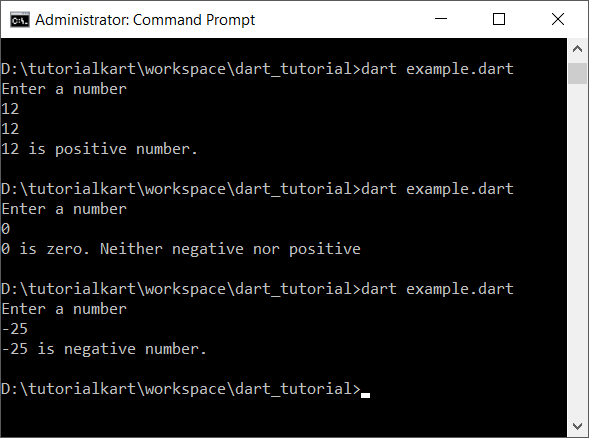
Conclusion
In this Dart Tutorial, we learned the syntax and usage of Dart Conditional Statement: If-Else-If.