What is Constructor in Apex Programming?
Constructor in Apex Programming is a code and is a special method that is invoked when an object is created from the class. Constructor has the following properties.
- Method name will be same as Class.
- Access specifier will be public.
- This method will invoked only one that is at the time of creating an object.
- This is used to instantiate the data members of the class.
Example :
public class TestObject { // the no argument Constructor public TestObject() { //code } }
Types of Constructor in Apex programming.
- Default Constructor.
- Non-parameterized Constructor.
- Parameterized Constructor.
Default Constructor
If an Apex Class doesn’t contain any constructor then Apex Compiler by default creates a dummy constructor on the name of class when we create an object for the class.
public class Example { } Example e = new Example();
As shown in above example, the Apex class doesn’t contain any Constructor. So when we create object for example class the Apex compiler creates a default constructor.
Non-parameterized Constructor & parameterized Constructor.
It is a constructor that doesn’t have any parameters or constructor that has parameters.
Apex Class
public class Example { Integer Rollno; String Name; public Example(Integer X, String myname) { Rollno = X; Name = myname; } Public Example() { Rollno = 1015; Name = 'Prasanth'; } }
Constructor in Apex programming with example
To create an Constructor in Apex programming, first we need to write an Apex class. To write an Apex class login to Salesforce and navigate to developer console and click on file.
- Select new Apex class and name the class.
Apex Class
public class Employee { string Employeename; Integer EmployeeNo; Public Employee() { EmployeeName = 'Prasant kumar'; EmployeeNo = 1015; } public void Show() { System.debug('Emplyeename is' +EmployeeName); System.debug('EmplyeeNo is' +EmployeeNo); } }
- Now open anonymous block.
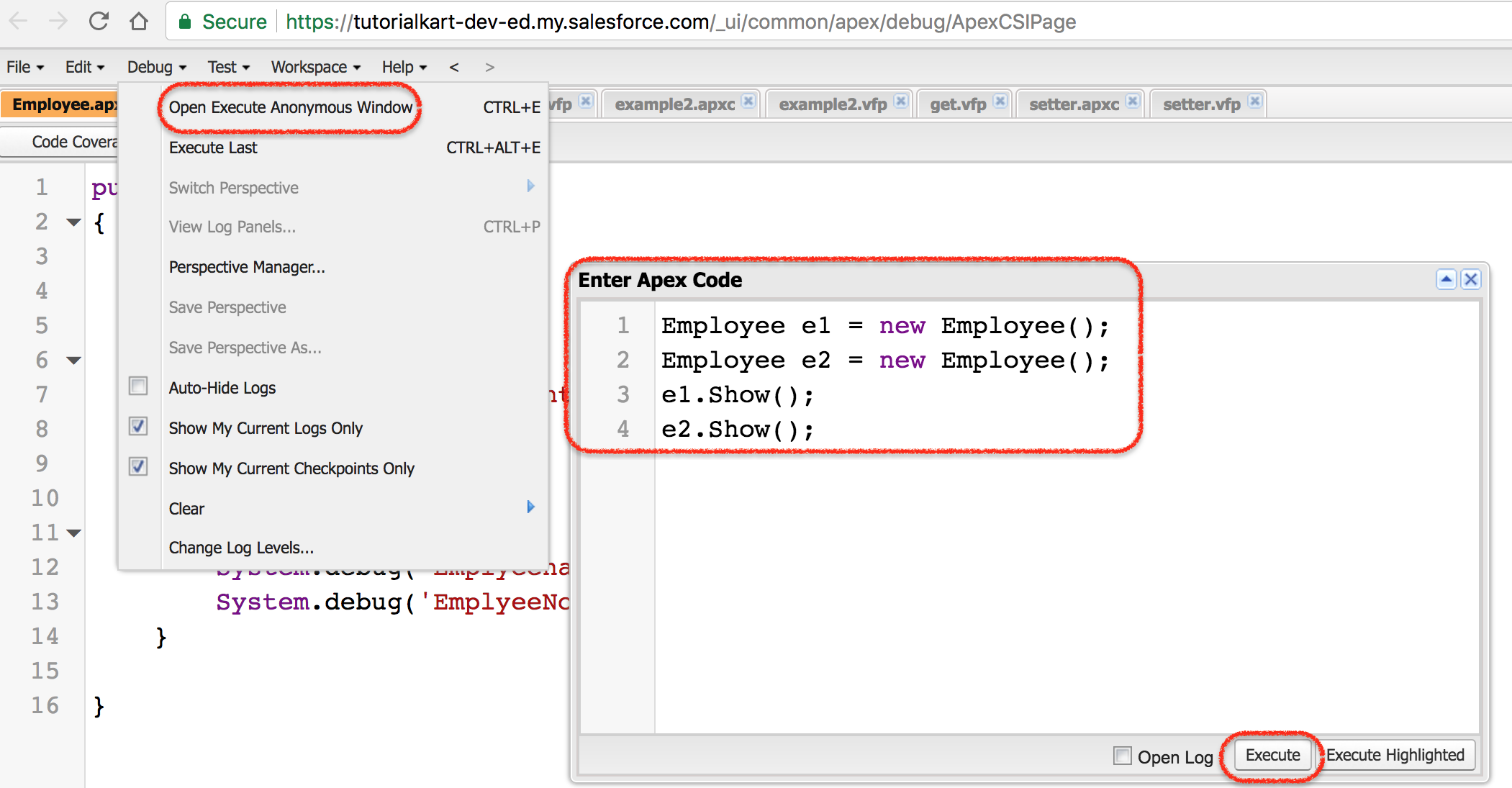
Click on Execute button.
- If we want to write a constructor with argument (parameterised constructor), then we ca nuse that constructor to create an object using those argument.
- If a class contain parameterised constructor, the default constructor won’t create automatically. We need to create no-argument constructor explicitly.
- Constructor in Apex programming can be overloaded.
- Constructor in Apex programming can call another constructor using (…) syntax. This is also called as Constructor Chaining.