Apex Trigger with before insert event
Apex Trigger with before insert event on a sObject is executed before an insert operation takes place in the sObject. sObject, for example, could be Contact, Account, etc.
First, create an Apex Trigger for a specific sObject with before insert. When an Apex Trigger is created, by default the before insert event is present. We can write code in the Trigger class to perform any validations on the fields or do a specific task before an insert operation is performed on the sObject.
In this tutorial, we create an Apex Trigger for Contact sObject. We write code in the Trigger to perform email validation. In particular, we try to respond with an error when a Contact is created with an existing Email in the database.
Syntax of Apex Trigger with before insert event
trigger Trigger_Name on sObject_Name (before insert) {
// code block section
}
Trigger_Name
and sObject_Name
are provided when you create a new Apex Trigger. After sObject_Name
, specify the event: before insert in paranthesis. You can write N number of statements in the code block section.
Before Insert Event
Following is the default code when an Apex Trigger is created with name:ValidateEmailTrigger on sObject:Contact for before insert event.
trigger ValidateEmailTrigger on Contact (before insert) {
}
Copy the following code to perform Email validation that we mentioned earlier.
trigger ValidateEmailTrigger on Contact (before insert) {
// set to store emails present in the contacts that initiated this trigger
set<String> emailSet = new set<String>();
// set to store existing emails that matched emailSet
set<String> existingEmails = new set<String>();
// add all the emails of contacts in trigger to emailSet
for(Contact con : Trigger.new) {
emailSet.add(con.email);
}
// get the existing emails which match the emails in emailSet
for(Contact con : [select Email from contact where email in : emailSet]) {
existingEmails.add(con.Email);
}
// for each contact in trigger.new
for(contact a:trigger.new) {
if(existingEmails.contains(a.Email)) {
a.Email.adderror('This email already exists. Msg from trigger.');
}
}
}
Following is an existing contact.
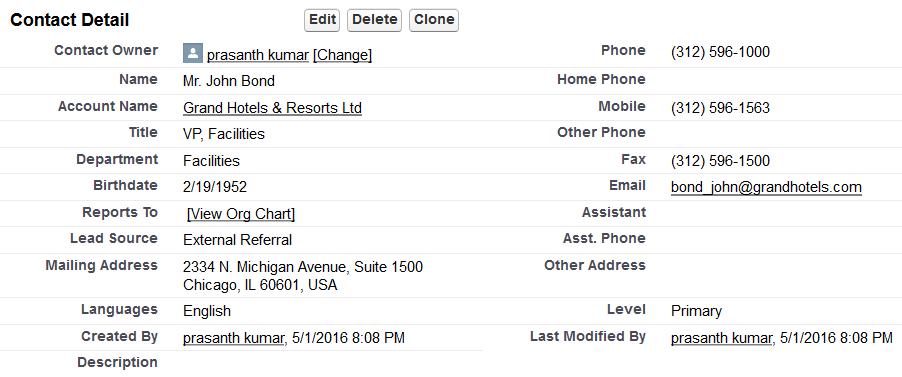
Now we shall try to create a new contact with the same Email: bond_john@grandhotels.com.
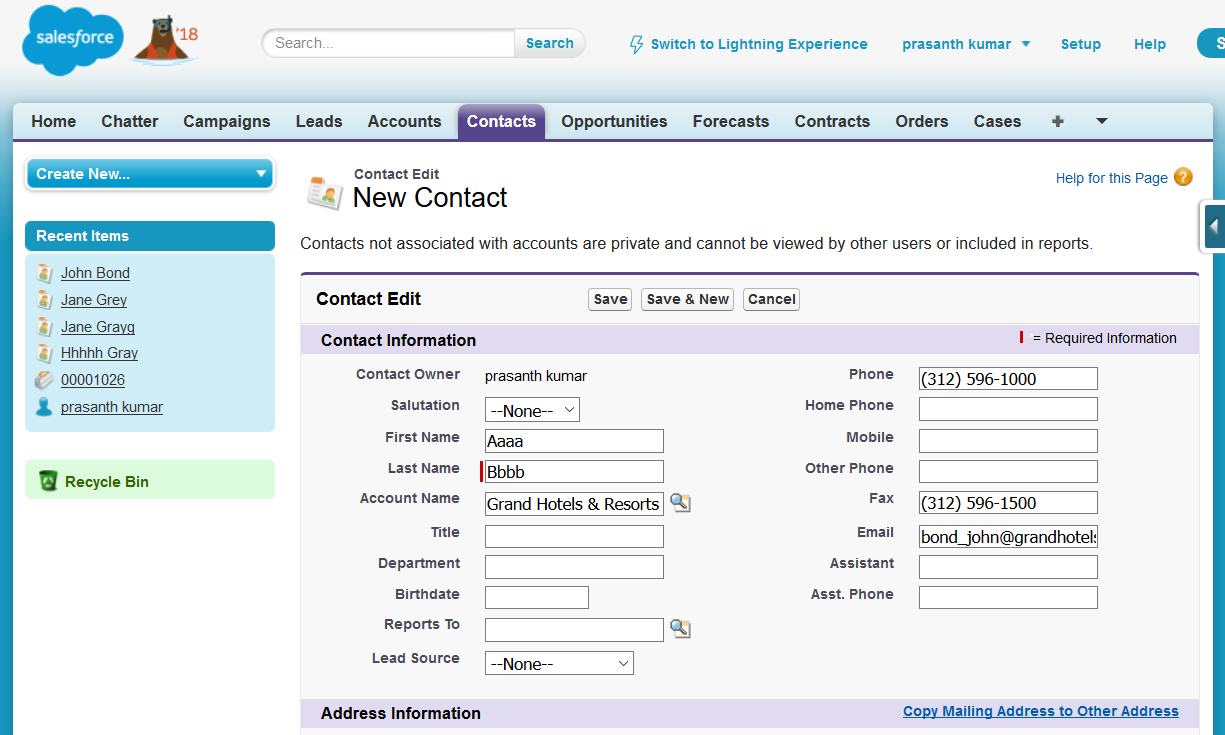
When you click on Save button, an insert happens. As we have written an Apex Trigger with before insert event, before the actual insertion of the record into the database, the ValidationEmailTrigger is executed.
The code in ValidationEmailTrigger gets executed. As the email matches one of the Email already present in the database, the statement which adds an error to Email field is executed.
a.Email.adderror('This email already exists. Msg from trigger.');
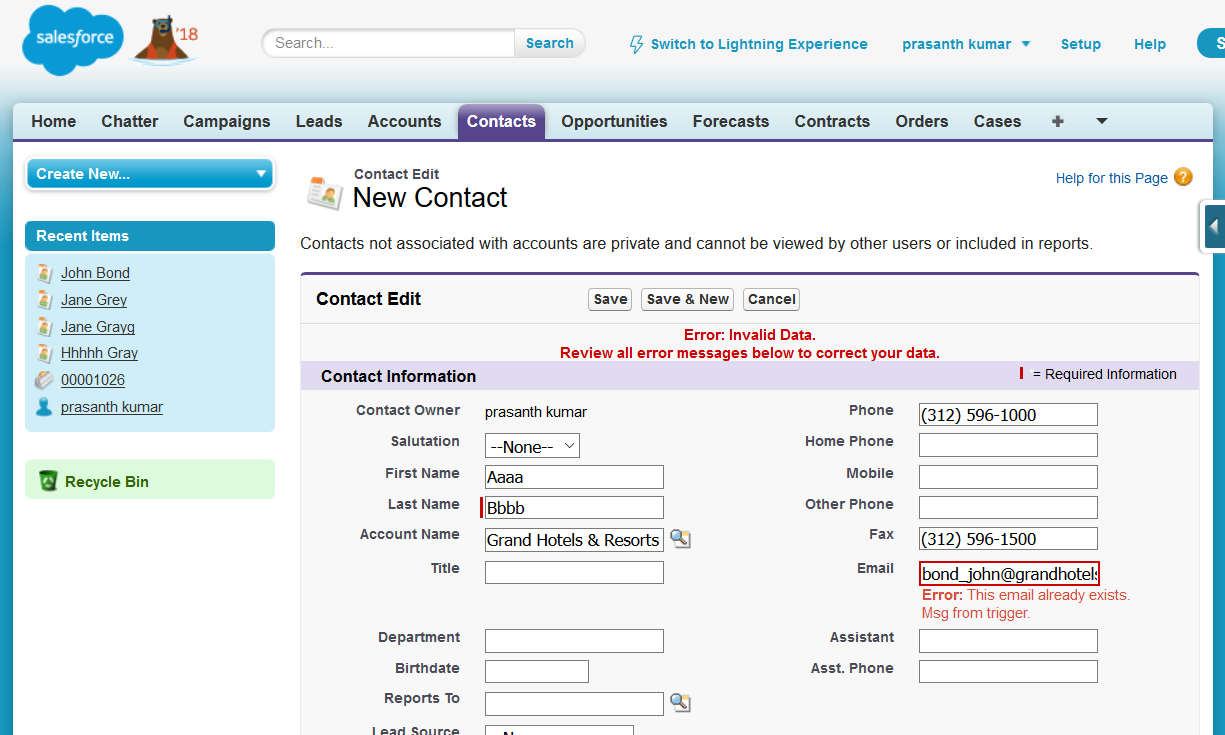
We have learned how to code an Apex Trigger with before insert event and write code to perform a specific task with the help an example. In our next tutorial, we shall learn about Apex Trigger with after insert event.