In this tutorial, you will learn how to setup Java Project with OpenNLP in Eclipse. The process should be same, to other IDEs as well.
Setup OpenNLP Java Project
Follow these steps.
1. Create project in Eclipse.
Create a Java Project in the Eclipse. (Open Eclipse -> File(in Menu) -> New -> Project -> Java -> Java Project)
Provide a project name (Ex : OpenNLPJavaTutorial) and click on “Finish”.
2. Jar files
Download jar files of openNLP from http://redrockdigimark.com/apachemirror/opennlp/.
At the time of writing this tutorial, opennlp-1.7.1 is the latest, and the list looks like in the below picture
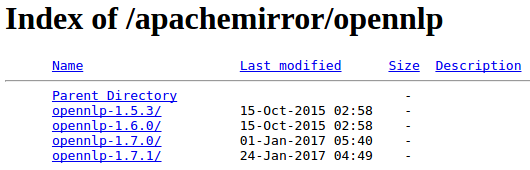
Step 4: Click on opennlp-1.7.1/ . We need bin package, because that could have the library (.jar) files.
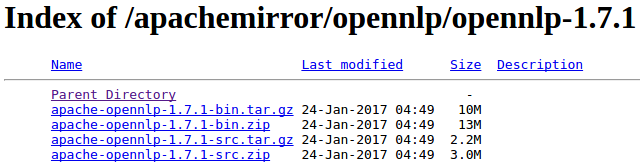
Click on apache-opennlp-1.7.1-bin.zip to download.
Once the zip file is downloaded, extract the contents, copy the lib folder and paste in the project as shown in the below picture.
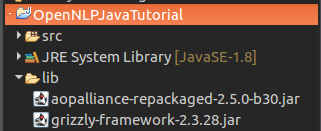
Lib folder should contain the list of below jar files:
aopalliance-repackaged-2.5.0-b30.jar
grizzly-framework-2.3.28.jar
grizzly-http-2.3.28.jar
grizzly-http-server-2.3.28.jar
hk2-api-2.5.0-b30.jar
hk2-locator-2.5.0-b30.jar
hk2-utils-2.5.0-b30.jar
hppc-0.7.1.jar
jackson-annotations-2.8.4.jar
jackson-core-2.8.4.jar
jackson-databind-2.8.4.jar
jackson-jaxrs-base-2.8.4.jar
jackson-jaxrs-json-provider-2.8.4.jar
jackson-module-jaxb-annotations-2.8.4.jar
javassist-3.20.0-GA.jar
javax.annotation-api-1.2.jar
javax.inject-2.5.0-b30.jar
javax.ws.rs-api-2.0.1.jar
jcommander-1.48.jar
jersey-client-2.25.jar
jersey-common-2.25.jar
jersey-container-grizzly2-http-2.25.jar
jersey-entity-filtering-2.25.jar
jersey-guava-2.25.jar
jersey-media-jaxb-2.25.jar
jersey-media-json-jackson-2.25.jar
jersey-server-2.25.jar
morfologik-fsa-2.1.0.jar
morfologik-fsa-builders-2.1.0.jar
morfologik-stemming-2.1.0.jar
morfologik-tools-2.1.0.jar
opennlp-brat-annotator-1.7.1.jar
opennlp-morfologik-addon-1.7.1.jar
opennlp-tools-1.7.1.jar
opennlp-uima-1.7.1.jar
osgi-resource-locator-1.0.1.jar
validation-api-1.1.0.Final.jar
3. Add jar files to build path
Add these jars to the build path (Project -> Properties -> Java Build Path -> Libraries -> Add Jars -> Select all the jars in lib folder -> Click “Apply” -> Click “OK”)
Apache has already trained some models for different problems in Natural Language Processing, with training data, and these models are available at http://opennlp.sourceforge.net/models-1.5/ . In the subsequent tutorials, we would refer to model files, which are available at this location. Do bookmark the link for a quick access.
We are ready with the openNLP Java Project Setup. Lets try Sentence detection using SentenceDetectExample.java.
4. Model file
Download “en-sent.bin” model file and place in the project. The final project structure should match with the structure shown in the below picture .
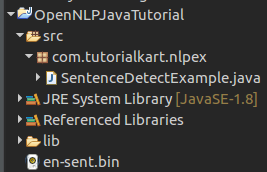
Example Java Project with OpenCV
We shall try out the example, SentenceDetectExample.java to check if the setup is good.
SentenceDetectExample.java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import com.fasterxml.jackson.databind.exc.InvalidFormatException;
import opennlp.tools.sentdetect.SentenceDetectorME;
import opennlp.tools.sentdetect.SentenceModel;
/**
* @author tutorialkart
*/
public class SentenceDetectExample {
public static void main(String[] args) {
try {
new SentenceDetectExample().sentenceDetect();
} catch (IOException e) {
e.printStackTrace();
}
}
public void sentenceDetect() throws InvalidFormatException, IOException {
String paragraph = "Apache openNLP supports the most common NLP tasks, such as tokenization, sentence segmentation, part-of-speech tagging, named entity extraction, chunking, parsing, and coreference resolution. These tasks are usually required to build more advanced text processing services. OpenNLP also includes maximum entropy and perceptron based machine learning.";
// refer to model file "en-sent,bin", available at link http://opennlp.sourceforge.net/models-1.5/
InputStream is = new FileInputStream("en-sent.bin");
SentenceModel model = new SentenceModel(is);
// load the model
SentenceDetectorME sdetector = new SentenceDetectorME(model);
// detect sentences in the paragraph
String sentences[] = sdetector.sentDetect(paragraph);
// print the sentences detected, to console
for(int i=0;i<sentences.length;i++){
System.out.println(sentences[i]);
}
is.close();
}
}
When SentenceDetectExample.java is run, the console output is:
Apache openNLP supports the most common NLP tasks, such as tokenization, sentence segmentation, part-of-speech tagging, named entity extraction, chunking, parsing, and coreference resolution.
These tasks are usually required to build more advanced text processing services.
OpenNLP also includes maximum entropy and perceptron based machine learning.
We are successfully done with the setup of openNLP Java Project in Eclipse.
Conclusion
In this OpenNLP Tutorial, we have seen the setup of openNLP Java Project in Eclipse. In our next OpenNLP tutorials, we shall look into following topics.