In this OpenCV tutorial using Python, you will learn how to read and display an image.
OpenCV Python – Read and Display Image
In Computer Vision applications, images are an integral part of the development process. Often there would be a need to read images and display them if required.
To read and display image using OpenCV Python, you could use cv2.imread() for reading image to a variable and cv2.imshow() to display the image in a separate window.
Syntax of cv2.imread()
The syntax of imread() function is
cv2.imread(/complete/path/to/image,flag)
First argument is complete path to the image along with the extension.
Second argument is an optional flag which could be any one of the following.
- cv2.IMREAD_COLOR : Loads a color image. Any transparency of image will be neglected. It is the default flag.
- cv2.IMREAD_GRAYSCALE : Loads image in grayscale mode
- cv2.IMREAD_UNCHANGED : Loads image as such including alpha channel
Returns numpy array, containing the pixel values. For colored images, each pixel is represented as an array containing Red, Green and Blue channels.
Note that the default flag is cv2.IMREAD_COLOR. Hence even if read a png image with transparency, the transparency channel is neglected.
Syntax of cv2.imshow()
cv2.imshow(window_name, image)
First argument is name of the window that is displayed when image is shown in.
Second argument is the image to be shown in the window.
Example
In the following Python program, we read an image at the location '/home/img/python.png'
, and then display the image in a separate window.
Python Program
import cv2
img = cv2.imread('/home/img/python.png')
cv2.imshow('sample image',img)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
When above program is run, an image is shown in another window named ‘sample image’, as specified in the arguments of imshow().
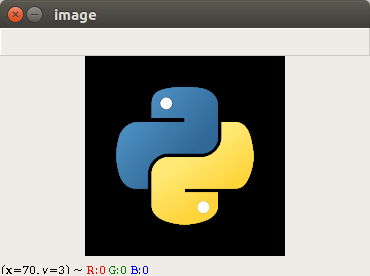
Right click on image in the window shows options for various operations out of which some are zoom-in, zoom-out etc.
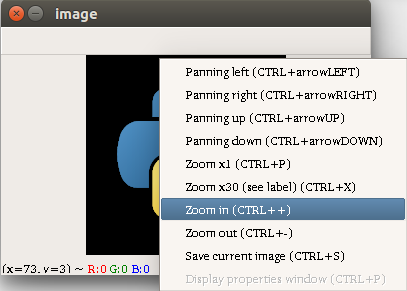
Conclusion
In this OpenCV Python Tutorial, we have learnt how to read and display an image using OpenCV library.