In this OpenCV tutorial, we will learn how to rotate an image to 90, 180 and 270 degrees using OpenCV Python using cv2.getRotationMatrix2D() and cv2.warpAffine() functions, with an example.
OpenCV Python – Rotate Image
We can rotate an image using OpenCV to any degree.
To rotate an image using OpenCV Python, first calculate the affine matrix that does the affine transformation (linear mapping of pixels), then warp the input image with the affine matrix.
Syntax of cv2: rotate image
M = cv2.getRotationMatrix2D(center, angle, scale)
rotated = cv2.warpAffine(img, M, (w, h))
where
- center: center of the image (the point about which rotation has to happen)
- angle: angle by which image has to be rotated in the anti-clockwise direction.
- rotated: ndarray that holds the rotated image data
- scale: 1.0 mean, the shape is preserved. Other value scales the image by the value provided.
Note: Please observe that the dimensions of the resulting image are provided same as that of the original image. When you are rotating by 90 or 270 and would to affect the height and width as well. swap height with width and width with height.
Examples
1. Rotate image 90 degrees, 180 degrees, and 270 degrees.
In this example, we will read an image, and then rotate it different angles liek 90 degrees, 180 degrees and 270 degrees. For each rotation with given degrees, we will save the image.
rotate-image.py
import cv2
# read image as grey scale
img = cv2.imread('/home/arjun/Desktop/logos/python.png')
# get image height, width
(h, w) = img.shape[:2
]
# calculate the center of the image
center = (w / 2, h / 2)
angle90 = 90
angle180 = 180
angle270 = 270
scale = 1.0
# Perform the counter clockwise rotation holding at the center
# 90 degrees
M = cv2.getRotationMatrix2D(center, angle90, scale)
rotated90 = cv2.warpAffine(img, M, (h, w))
# 180 degrees
M = cv2.getRotationMatrix2D(center, angle180, scale)
rotated180 = cv2.warpAffine(img, M, (w, h))
# 270 degrees
M = cv2.getRotationMatrix2D(center, angle270, scale)
rotated270 = cv2.warpAffine(img, M, (h, w))
cv2.imshow('Original Image',img)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
cv2.imshow('Image rotated by 90 degrees',rotated90)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
cv2.imshow('Image rotated by 180 degrees',rotated180)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
cv2.imshow('Image rotated by 270 degrees',rotated270)
cv2.waitKey(0) # waits until a key is pressed
cv2.destroyAllWindows() # destroys the window showing image
Original Image
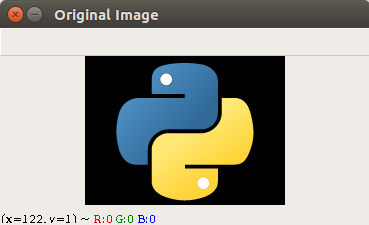
90-degree rotation anti-clockwise
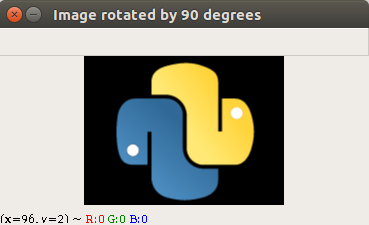
180-degree rotation anti-clockwise
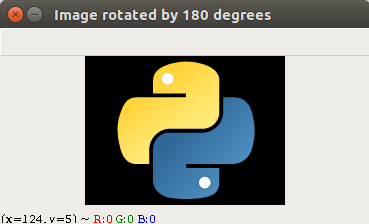
270-degree rotation anti-clockwise
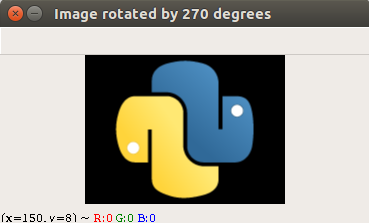
Conclusion
In this OpenCV Tutorial, we have learned how to rotate an image by 90, 180 and 270 degrees.