Python Tkinter Canvas Widget
Tkinter Canvas Widget is a rectangular area that allows for drawing shapes, pictures or complex layouts. You can draw shapes like arc, line, oval, rectangle, polygon; objects like bitmap, image, text, and windows.
In this tutorial, we will learn how to create Canvas widget and how to use it in your GUI application to draw some shapes on to it.
Create Canvas Widget
To create a Canvas widget in your Python GUI application, use the following syntax,
widget = tk.Canvas(parent, option, ...)
where parent is the root window or a frame in which we would like to pack this Canvas widget. You can provide one or more options supported for Canvas widget to change its appearance and behavior.
Example 1 – Tkinter Canvas – Basic Example
In the following example, we shall create a simple Canvas widget with a specific width and height, and also a background color. These width, height and background color are some of the options supported by Canvas widget.
example.py – Python Program
import tkinter
window_main = tkinter.Tk(className='Tkinter - TutorialKart', )
window_main.geometry("450x250")
canvas = tkinter.Canvas(window_main, bg="#a0ebbb", height=200, width=300)
canvas.pack()
window_main.mainloop()
Output
The light green color area in the following screenshot is the Tkinter Canvas widget, that we have packed into the main window.
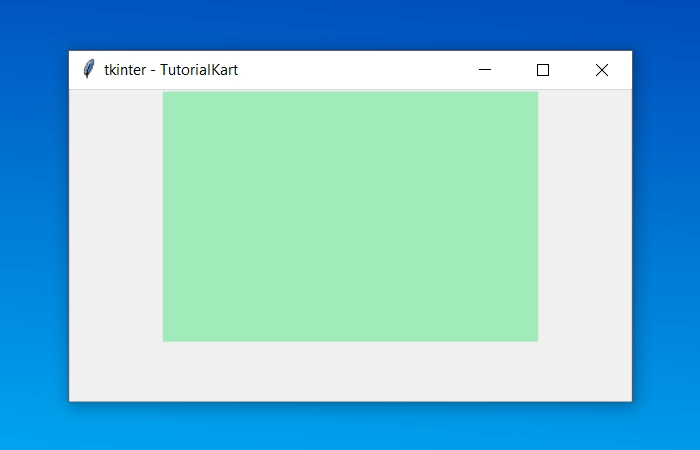
Example 2 – Tkinter Canvas – Draw Shapes
In the following program, we create a Canvas widget and draw shapes like arc and oval onto the canvas.
example.py – Python Program
import tkinter
window_main = tkinter.Tk(className='Tkinter - TutorialKart', )
window_main.geometry("450x250")
canvas = tkinter.Canvas(window_main, bg="#a0ebbb", height=200, width=300)
coordinates_arc = 50, 50, 150, 150 #x1, y1, x2, y2
arc = canvas.create_arc(coordinates_arc, start=40, extent=300, fill="#395c45")
coordinates_oval = 200, 50, 250, 150 #x1, y1, x2, y2
arc = canvas.create_oval(coordinates_oval, fill="#395c45")
canvas.pack()
window_main.mainloop()
Output
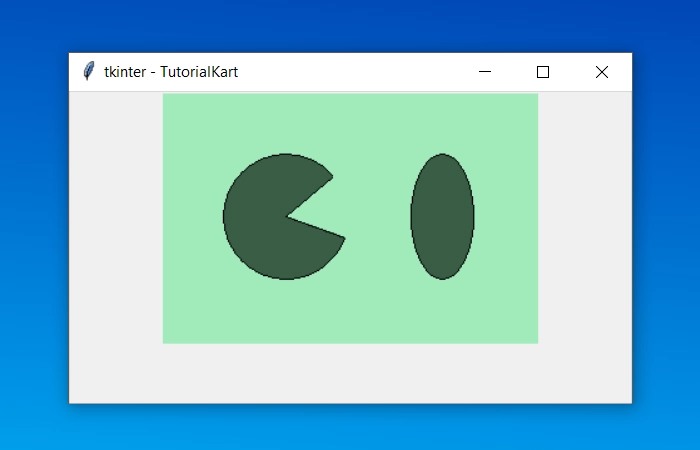
Conclusion
In this Python Tutorial, we learned how to create a Canvas widget in our GUI application, and how to draw some of the basic shapes on to it, with the help of example programs.