Python Tkinter Label Widget
Tkinter Label widget displays one or more lines of text, image or bitmap.
In this tutorial, we will learn how to create Label widget and how to use it in your GUI application to display some text or an image.
Create Tkinter Label Widget
To create a Label widget in your Python GUI application, use the following syntax,
widget = tk.Label(parent, option, ...)
where parent
is the root window or a frame in which we would like to pack this Label widget. You can provide one or more options supported for Label widget to change its appearance and behavior.
Example 1 – Tkinter Label
In the following program, we will create a Label widget and display it in the root window.
example.py – Python Program
import tkinter as tk
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry("400x200")
label_1 = tk.Label(window_main, text='Hello World')
label_1.pack()
window_main.mainloop()
Output
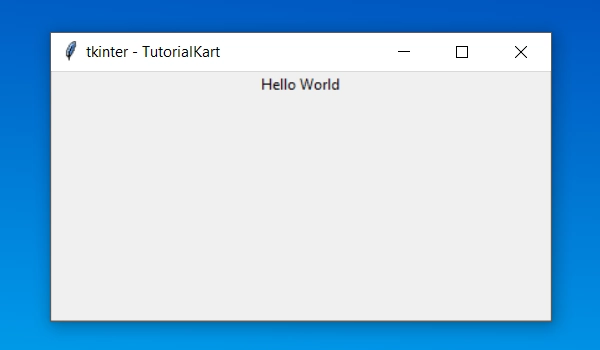
Example 2 – Tkinter Label – With Font Style
In the following example, we will apply font style to the text using font option of Label widget.
example.py – Python Program
import tkinter as tk
import tkinter.font as font
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry("400x200")
labelFont = font.Font(family='Helvitica', size=20)
label_1 = tk.Label(window_main, text='Hello World', fg='red', font=labelFont)
label_1.pack()
window_main.mainloop()
Output
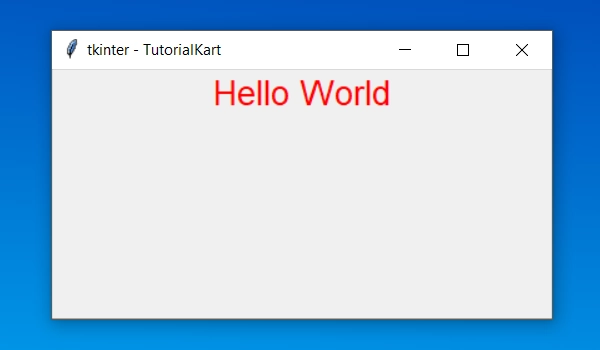
Conclusion
In this Python Tutorial, we learned what a Label widget is, how to create one, and how to use some of its options to change its appearance.