Python Tkinter Message
Tkinter Message widget can display text in multiple lines.
Unlike Label widget, Tkinter Message widget cannot display an image.
In this tutorial, we will learn how to create Message widget and how to use it in your GUI application to display some text in multiple lines.
Create Tkinter Message Widget
To create a Message widget in your Python GUI application, use the following syntax,
widget = tk.Message(parent, option, ...)
where parent
is the root window or a frame in which we would like to pack this Message widget. You can provide one or more options supported for Message widget to change its appearance and behavior.
Example 1 – Tkinter Message
In the following program, we will create a Message widget and add it to a Tkinter root window.
Python Program
import tkinter as tk
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry("400x200")
message_1 = tk.Message(window_main, text='Welcome to TutorialKart!')
message_1.pack()
window_main.mainloop()
Output
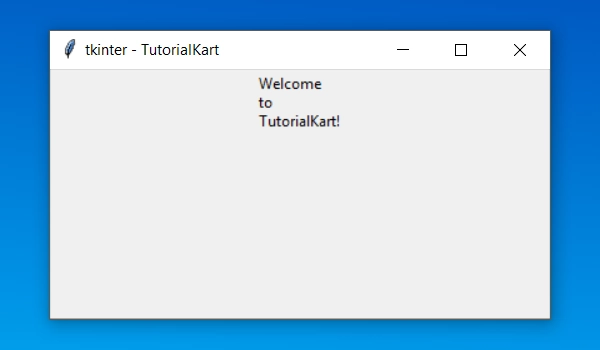
Example 2 – Tkinter Message – With Some Styles
In the following program, we will create a Message widget with some modified appearance by changing font, justification or alignment, padding, width, and background color. These are some of the options supported for Message widget.
example.py – Python Program
import tkinter as tk
import tkinter.font as font
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry("400x200")
message_font = font.Font(size=20)
message_1 = tk.Message(window_main,
text='Welcome to TutorialKart!',
bg='#ecffd9',
font=message_font,
justify=tk.CENTER,
width=150,
padx=20
)
message_1.pack()
window_main.mainloop()
Output
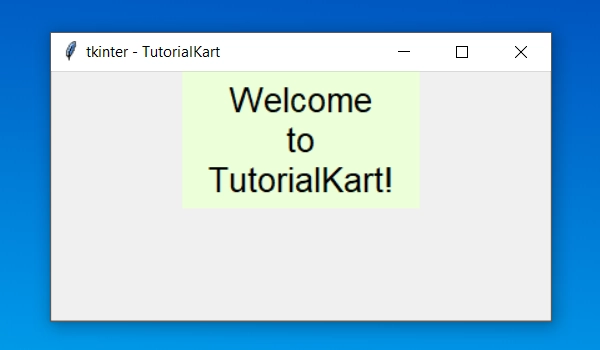
Conclusion
In this Python Tutorial, we learned what a Message widget is, how to create one, and how to use some of its options to change its appearance.