Python Tkinter Entry
The Entry
widget in Tkinter is used to accept single-line text input from the user. It is commonly used for login forms, data entry fields, and text input in GUI applications.
The Entry
widget supports various options for customizing appearance, retrieving user input, and handling events.
In this tutorial, we will explore how to use the Tkinter Entry widget with multiple examples.
Syntax
tk.Entry(master, **options)
Parameters
Parameter | Type | Description |
---|---|---|
master | Widget | The parent widget, usually a frame or root window. |
textvariable | StringVar | Used to store and retrieve text from the Entry widget. |
width | Integer | Defines the width of the Entry field (in characters). |
show | String | Masks input text (e.g., show="*" for passwords). |
state | String | Defines the state of the Entry field (normal , disabled , readonly ). |
Examples
1. Basic Entry Widget
In this example, we create a basic Entry widget where users can type text.
import tkinter as tk
root = tk.Tk()
root.title("Tkinter Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=10)
root.mainloop()
Output in Windows:
A simple input field appears where users can type text.
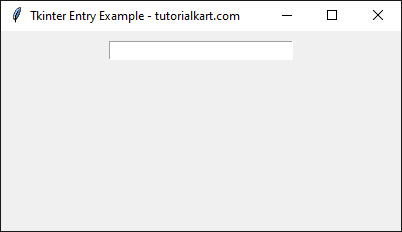
Click on the entry widget, and a cursor appear. You may start typing some text into the entry field.
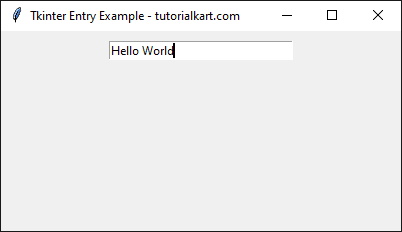
2. Retrieving User Input
This example demonstrates how to retrieve text from an Entry widget using a button click.
import tkinter as tk
root = tk.Tk()
root.title("Retrieve Entry Text - tutorialkart.com")
root.geometry("400x200")
def show_text():
print("Entered Text:", entry.get())
# Create an Entry widget
entry = tk.Entry(root, width=30)
entry.pack(pady=10)
# Create a button to retrieve text
btn = tk.Button(root, text="Get Text", command=show_text)
btn.pack()
root.mainloop()
Output in Windows:
A text field appears with a button.
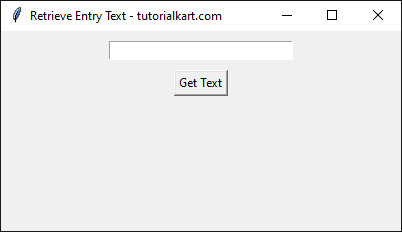
Enter some text into the text filed.
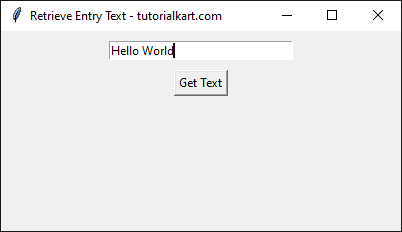
Clicking the button prints the entered text in the console.
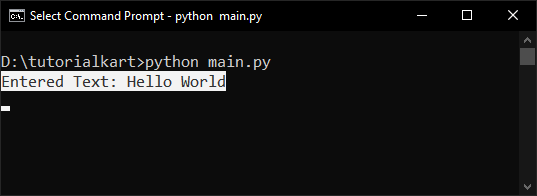
3. Password Entry Field
In this example, we will learn how to use the show
option to mask user input (useful for passwords).
import tkinter as tk
root = tk.Tk()
root.title("Password Entry - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with masked input
entry = tk.Entry(root, width=30, show="*")
entry.pack(pady=10)
root.mainloop()
Output in Windows:
A password input field appears where typed characters are hidden.
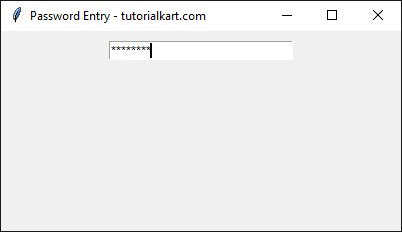
4. Read-Only Entry Field
In this example, we will learn how to use the state
option to make an Entry field read-only.
import tkinter as tk
root = tk.Tk()
root.title("Read-Only Entry - tutorialkart.com")
root.geometry("400x200")
# Create a read-only Entry widget
entry = tk.Entry(root, width=30, state="readonly")
entry.pack(pady=10)
root.mainloop()
Output in Windows:
An entry field is displayed but users cannot type in it.
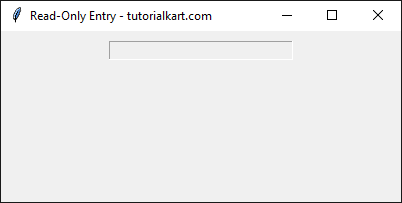
Conclusion
In this tutorial, we explored how to use the Entry
widget in Tkinter. We covered:
- Creating a basic Entry widget
- Retrieving user input
- Using a password-style input field
- Making an Entry field read-only
The Entry
widget is an essential component in Tkinter for accepting user input in GUI applications.