Python Tkinter Frame Width Height Not Working
By default, Tkinter Frame fits to its children and thus its width and height depends on its children. You can override this behavior and force a specific width and height to the frame.
To force the width and height of frame widget call pack_propagate(0) on the frame.
</>
Copy
frame_1.pack_propagate(0)
Now the width and height options specified in the frame will take affect.
Example 1 – Set Specific Width & Height for Tkinter Frame
In the following Python program, we will create two Tkinter Frames and force it to render based on the dimensions: width and height provided in the options.
example.py – Python Program
</>
Copy
import tkinter as tk
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry("400x200")
frame_1 = tk.Frame(window_main, bg='#c4ffd2', width=200, height=50)
frame_1.pack()
frame_1.pack_propagate(0)
frame_2 = tk.Frame(window_main, bg='#ffffff', width=350, height=70)
frame_2.pack()
frame_2.pack_propagate(0)
#in frame_1
label_1 = tk.Label(frame_1, text='Name')
label_1.pack(side=tk.LEFT)
submit = tk.Entry(frame_1)
submit.pack(side=tk.RIGHT)
#in frame_2
button_reset = tk.Button(frame_2, text='Reset')
button_reset.pack(side=tk.LEFT)
button_submit = tk.Button(frame_2, text='Submit')
button_submit.pack(side=tk.RIGHT)
window_main.mainloop()
Output
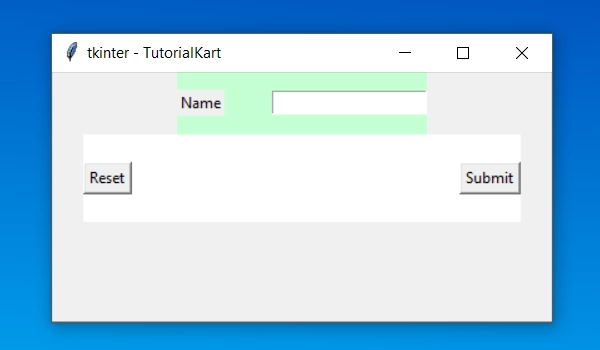
Conclusion
In this Python Tutorial, we learned how to force a specific width and height to the Tkinter Frame widget.