SwiftUI Text View
SwiftUI Text is a view that is used to display one or more lines of text in Application UI. The text displayed is read only.
Example Text View
The following code snippet is a simple example to create a SwiftUI Text view.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
}
}
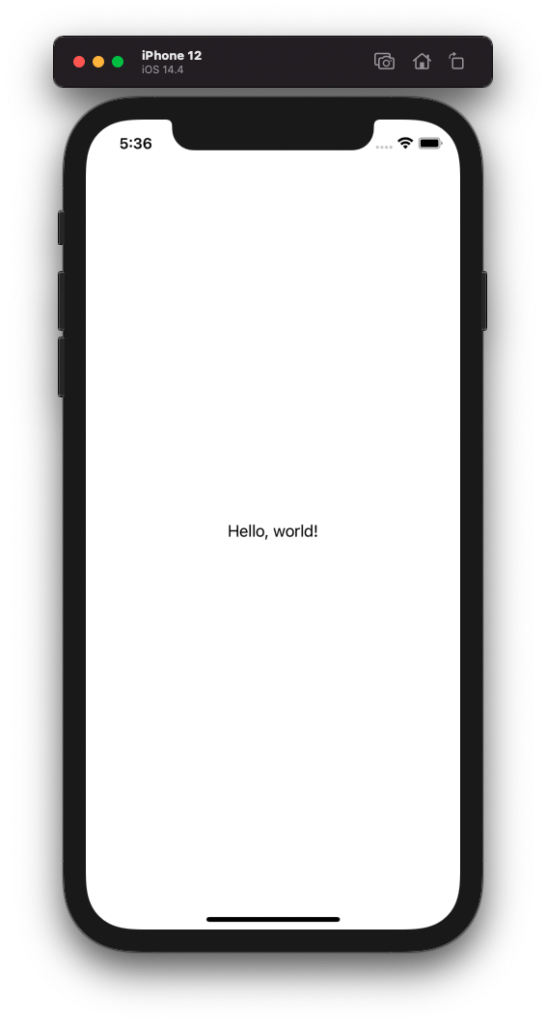
SwiftUI Text View Modifiers
We can use view modifiers to change the styling of the text in Text view.
Bold Text
bold() modifier makes the text in Text view to bold.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.bold()
}
}
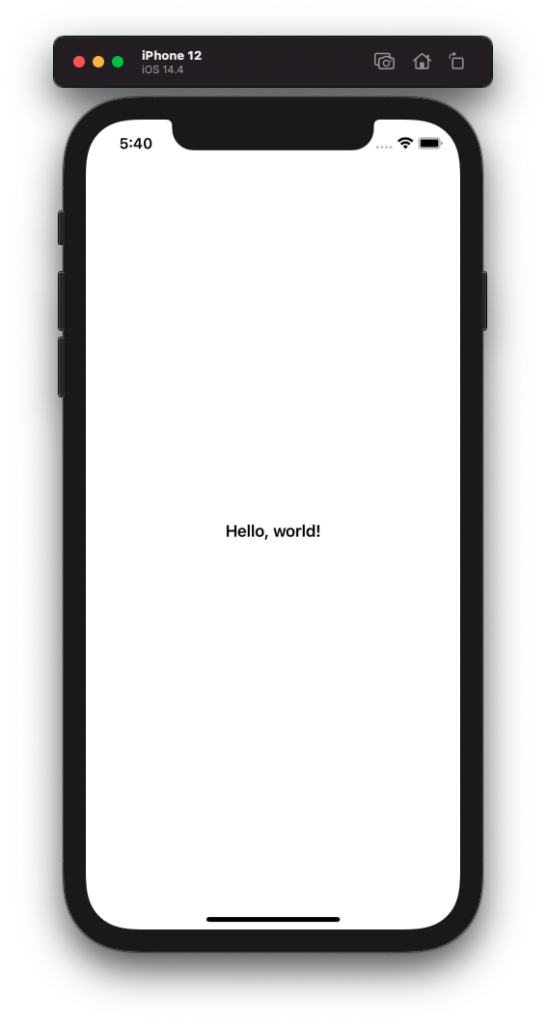
Italic Text
italic() modifier makes the text in Text view to italic.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.italic()
}
}
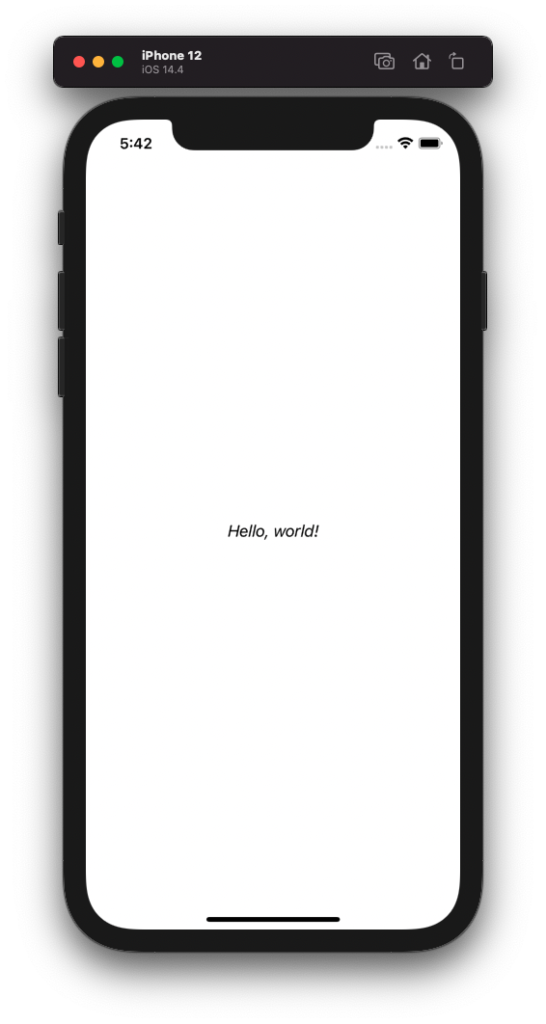
Text as Title
To display the text as standard title in Mac/iOS, use font(_:) modifier.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.font(.title)
}
}
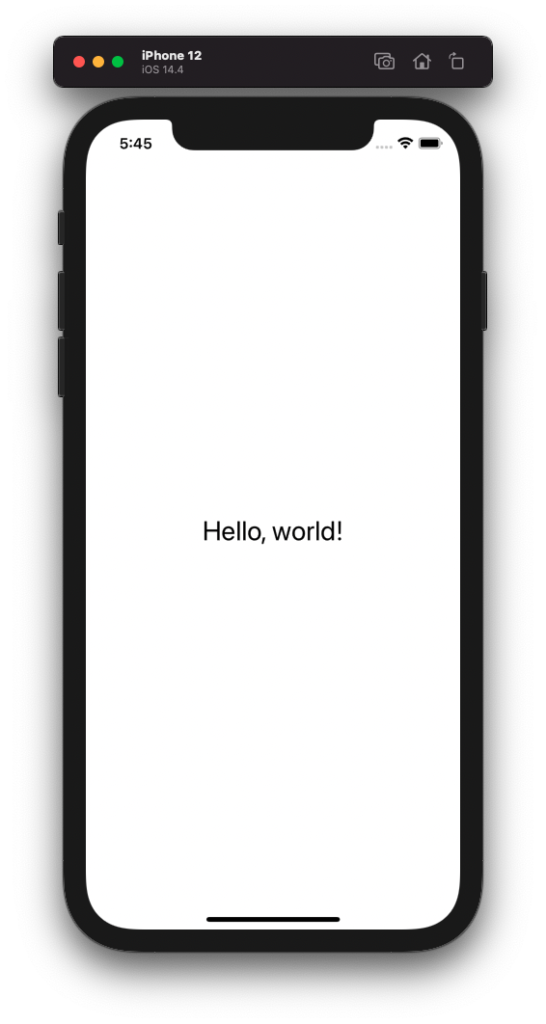
Underline Text
To underline the text in Text view, use underline() modifier.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.underline()
}
}
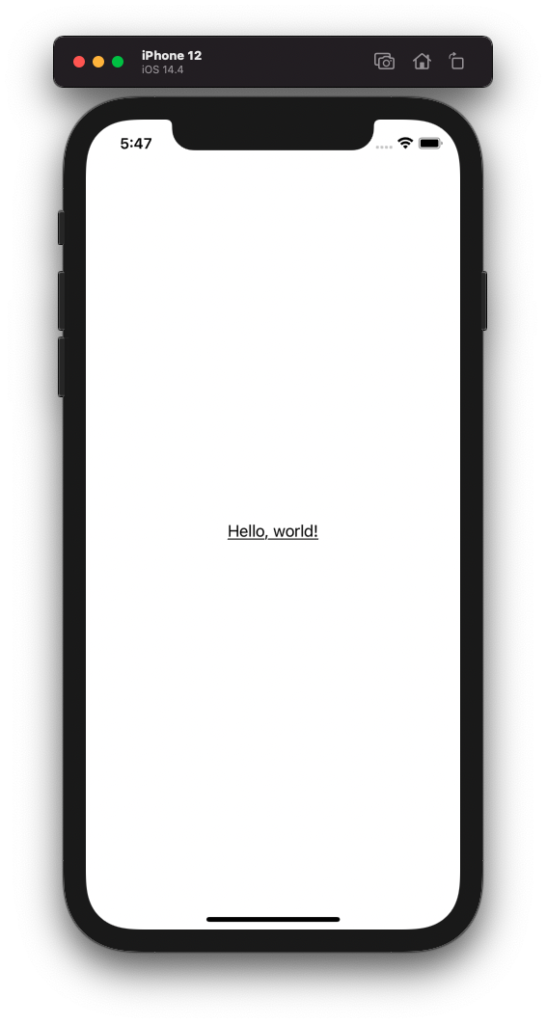
Text Color
To change the color of text in Text view, use foregroundColor() modifier.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.foregroundColor(.red)
}
}
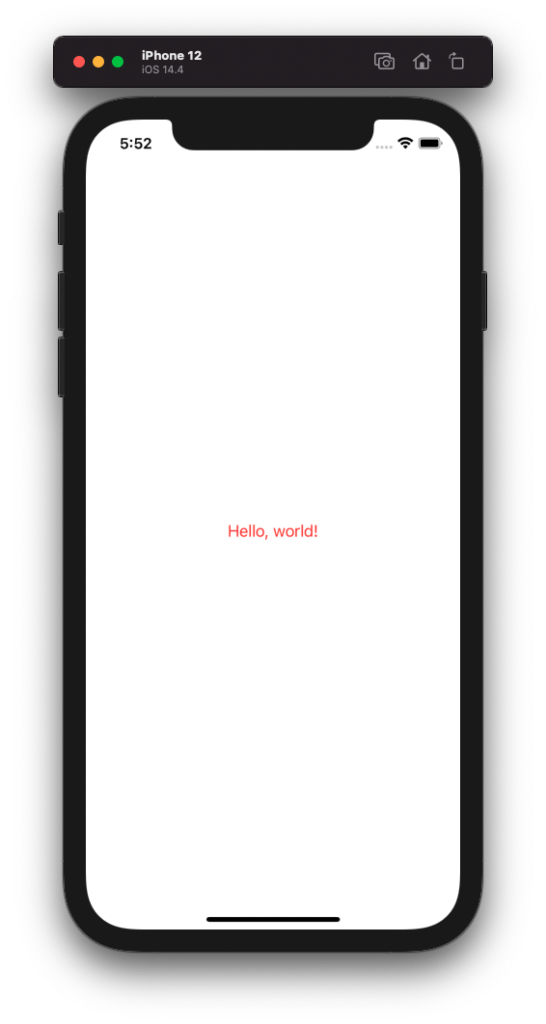
Text Size
To change the font size of text in Text view, use font() modifier.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.font(.system(size: 100))
}
}
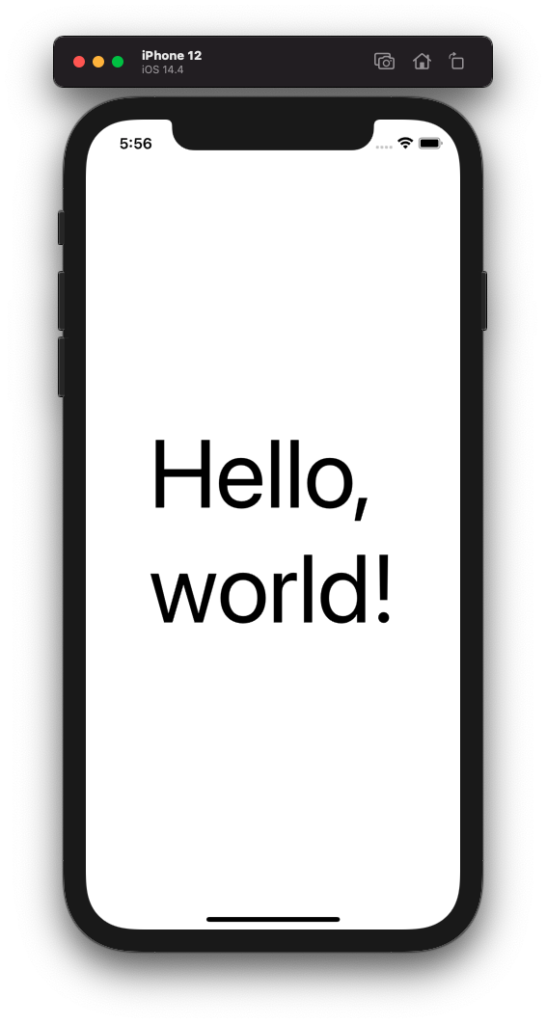
Text Uppercase
To change the casing of text in Text view to uppercase, use textCase() modifier.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.textCase(.uppercase)
}
}
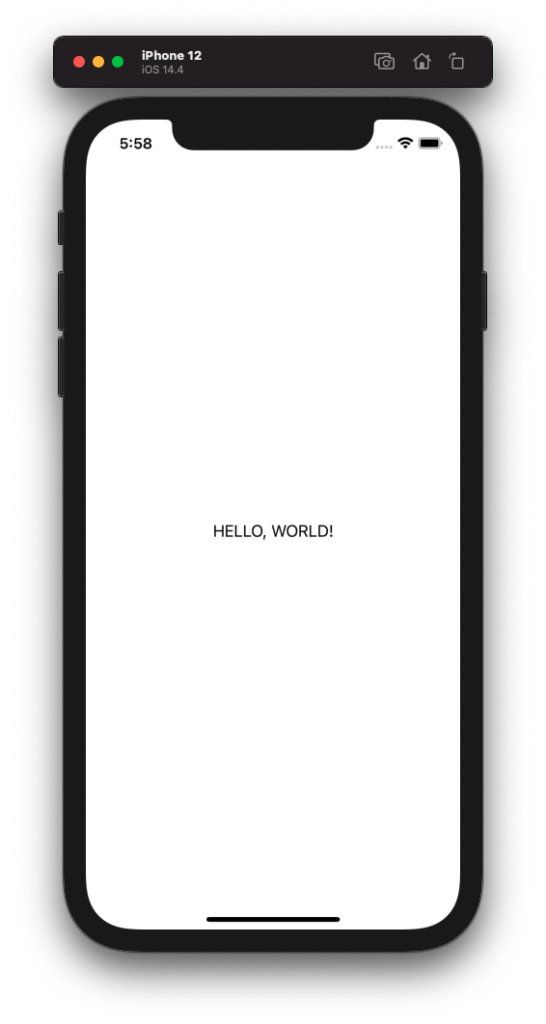
Text Lowercase
To change the casing of text in Text view to lowercase, use textCase() modifier.
ContentView.swift
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.textCase(.uppercase)
}
}
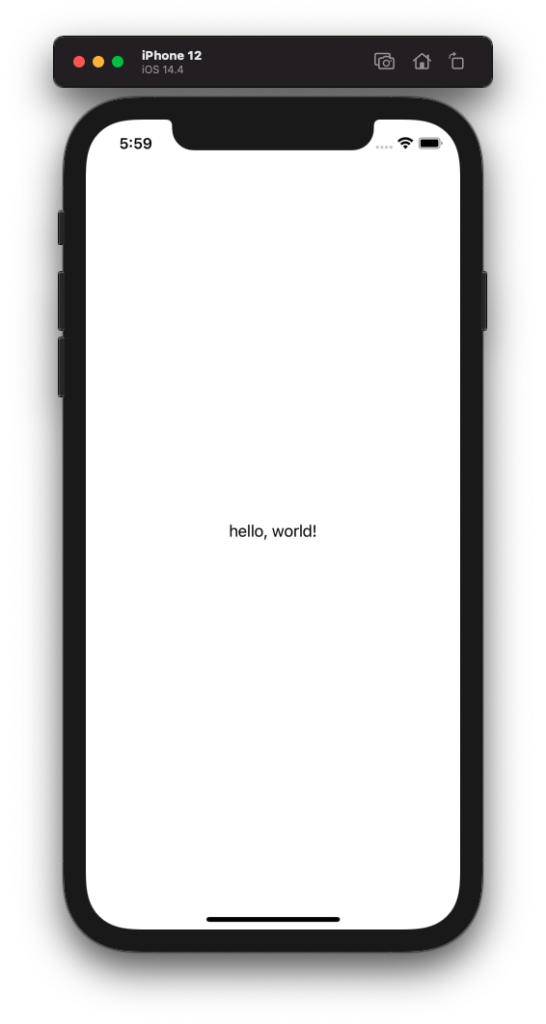
Conclusion
Concluding this SwiftUI Tutorial for Text view, we have learned how to create a Text view in SwiftUI, and how to modify the styling of the text in Text view using different modifiers. We have covered only some of the modifiers for Text view. For other examples on modifiers for Text view, visit following tutorials.