In this tutorial, we will write a Python program to check if the given year is a leap year or not.
Python Leap Year Program
An year is said to be leap year if it divisible by 4 and not divisible by 100, with an exception that it is divisible by 400.
Algorithm
Following is the algorithm that we shall use to check if given input year is leap year or not.
- Read an integer from user, to year variable.
- Check the condition if year is exactly divisible by 4 and 100, or the year is exactly divisible by 400.
- If the above condition returns true, given year is leap year else it is not leap year.
ADVERTISEMENT
Leap Year Program
Python Program
year = int(input('Enter year : ')) if (year%4 == 0 and year%100 != 0) or (year%400 == 0) : print(year, "is a leap year.") else : print(year, "is not a leap year.")
Output
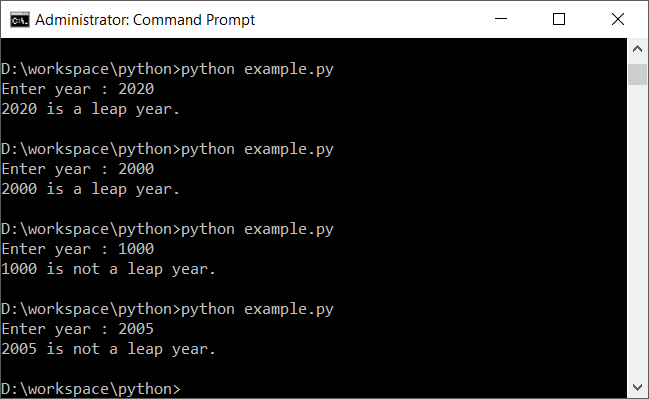
Conclusion
In this Python Tutorial, we learned how to check if given year is leap year or not.