In this Python tutorial, we will learn about For Loop statement, its syntax, and cover examples with For Loop where it is used to iterate over different types of sequences and collections.
Python – For Loop
Python For loop is used to execute a set of statements iteratively over a sequence of elements.
We shall also discuss Nested For Loop in this tutorial.
Syntax
The syntax of For Loop in Python is
for <variable> in <iterable>: statement(s)
where an iterable could be a sequence or collection or any custom Python class object that implements __iter__() method.
Flowchart
The flow of program execution in a Python For Loop is shown in the following picture.
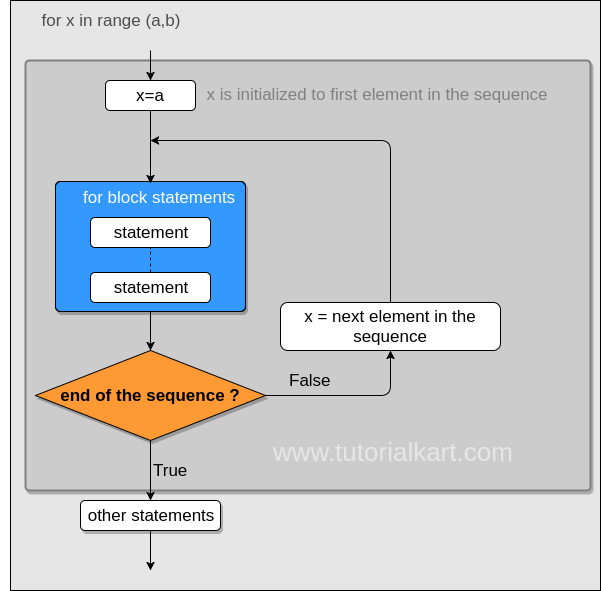
For Loop with List
In this example, we will take a list of numbers and use for loop to iterate over each of the element in this list.
Example.py
myList = [1, 2, 3, 5, 6, 10, 11, 12] for item in myList: print(item)Try Online
Output
1 2 3 5 6 10 11 12
For Loop with Range
In this example, we will take a range object, and use for loop to iterate over each number in this range.
Example.py
myRange = range(1, 5) for number in myRange: print(number)Try Online
Output
1 2 3 4
For loop with String
In this example, we will take a String and use for loop to iterate over each character of the string.
Example.py
myString = 'tutorialkart' for ch in myString: print(ch)Try Online
Output
t u t o r i a l k a r t
For loop with Tuple
In this example, we will take a tuple and use for loop to iterate over each item in the tuple.
Example.py
myTuple = ('abc', 'bcd', 'xyz') for item in myTuple: print(item)Try Online
Output
abc bcd xyz
Nested For Loop
Any statement inside the for loop could be another for loop, if block, if-else block or any such.
An example program for nested for loop with embedded if-else blocks is given below.
Example.py
for greeting in ['hello', 'hi', 'hola']: for ch in greeting: print(ch) if greeting=='hello': print("hello buddy!") else: if greeting=='hi': print("hi friend!") else: print("hola gola!")Try Online
Output
h e l l o hello buddy! h i hi friend! h o l a hola gola!
Conclusion
In this Python Tutorial, we have learn about for loop execution flow, for loop syntax in python, nested for loop, for loops that iterate over list, range, string and tuple. As an exercise, try out the for loop that iterates over buffer.