Render Array of Objects as Table in React
Rendering an array of objects as a table is required when displaying data such as user lists, product details, or any other structured information. React makes it easy to dynamically create table rows and columns by iterating over the array and rendering each object’s properties.
In this tutorial, we will walk you through the process of rendering an array of objects as a table in React with detailed examples and explanations.
By the end of this tutorial, you will learn how to:
- Use the
map
method to render table rows and cells dynamically - Assign unique keys to rows for better performance
- Handle nested object properties when rendering a table
Steps to Render Array of Objects as a Table
Step 1: Prepare the Data
Start by preparing an array of objects containing the data you want to display in the table. Each object represents a row, and its properties represent the columns.
Step 2: Create the Table Structure
Create the basic structure of the table using the <table>
, <thead>
, <tbody>
, and <tr>
elements. Use the map
method to iterate over the array and render rows and cells dynamically.
Step 3: Assign Unique Keys
For each row, assign a unique key
prop to help React efficiently update and manage the DOM.
Examples
Example 1: Rendering a Simple Array of Objects as a Table
In this example, we render an array of objects with basic properties as a table.
Filename: App.js
import React from 'react';
const App = () => {
const data = [
{ id: 1, name: 'Alice', age: 25, profession: 'Engineer' },
{ id: 2, name: 'Bob', age: 30, profession: 'Designer' },
{ id: 3, name: 'Charlie', age: 35, profession: 'Teacher' }
];
return (
<div>
<h1>User Table</h1>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
<th>Profession</th>
</tr>
</thead>
<tbody>
{data.map((user) => (
<tr key={user.id}>
<td>{user.id}</td>
<td>{user.name}</td>
<td>{user.age}</td>
<td>{user.profession}</td>
</tr>
))}
</tbody>
</table>
</div>
);
};
export default App;
Explanation:
- The
data
array contains objects withid
,name
,age
, andprofession
properties. - The
map
method is used to iterate over the array and create a table row (<tr>
) for each object. - The
key
prop is set touser.id
to uniquely identify each row. - Each property of the object is displayed in a separate table cell (
<td>
).
Output
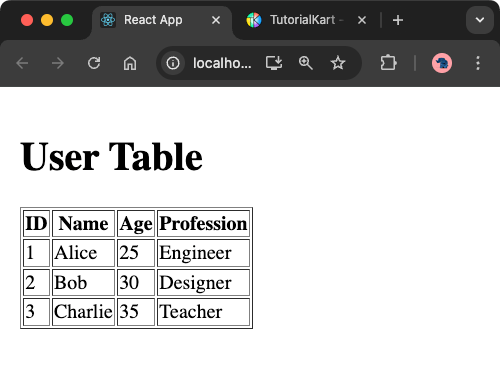
Example 2: Handling Nested Objects in Table Data
This example demonstrates how to handle nested properties when rendering a table.
Filename: App.js
import React from 'react';
const App = () => {
const data = [
{ id: 1, name: 'Alice', contact: { email: 'alice@example.com', phone: '123-456-7890' } },
{ id: 2, name: 'Bob', contact: { email: 'bob@example.com', phone: '987-654-3210' } },
{ id: 3, name: 'Charlie', contact: { email: 'charlie@example.com', phone: '555-555-5555' } }
];
return (
<div>
<h1>Contact Table</h1>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
</tr>
</thead>
<tbody>
{data.map((user) => (
<tr key={user.id}>
<td>{user.id}</td>
<td>{user.name}</td>
<td>{user.contact.email}</td>
<td>{user.contact.phone}</td>
</tr>
))}
</tbody>
</table>
</div>
);
};
export default App;
Explanation:
- The
data
array contains objects with nestedcontact
properties. - We access nested properties (e.g.,
user.contact.email
) inside themap
method to display them in the table. - Each row is uniquely identified by the
key
prop set touser.id
.
Output
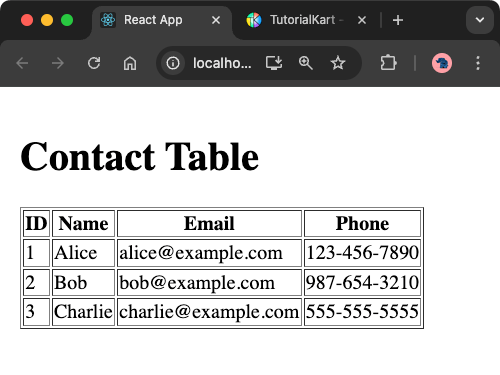
Conclusion
In this tutorial, you learned how to render an array of objects as a table in React. You used the map
method to dynamically generate table rows and cells, assigned unique keys to rows, and handled nested object properties. By following these techniques, you can efficiently display structured data in your React applications.