C Do-While Loop
C Do-While Loop statement is same as C While Loop, but differs in the order of execution of condition and while block.
In while loop, the program first checks the condition and then executes the while block based on the boolean value the condition returns. Whereas in do-while loop, the while block is executed first, and then the condition is checked whether to continue the loop or not.
In this tutorial, we will learn the syntax of do-while loop, its execution flow using flow diagram, and its usage using example programs.
Syntax of C Do-While Loop
The syntax of do-while loop in C programming language is given below.
do { //while block statement(s) } while (condition);
where
do is C keyword.
while block statement(s) are the set of statements that execute during each iteration. These set of statements are enclosed in flower braces {}.
while is C keyword.
condition is an expression that evaluates to boolean value.
Flow Diagram of C Do-While Loop
The execution flow diagram of do-while loop in C is given below.
The step by step process of do-while loop execution is:
- Start do-while loop.
- Execute while block statement(s).
- Check the condition. If true, go to step 2.
- End do-while loop.
Example for C Do-While Loop
In the following program, we use do-while loop to print numbers from 0 to 5.
C Program
#include <stdio.h> void main() { int i = 0; do { printf("%d\n", i); i++; } while (i <= 5); }
Output
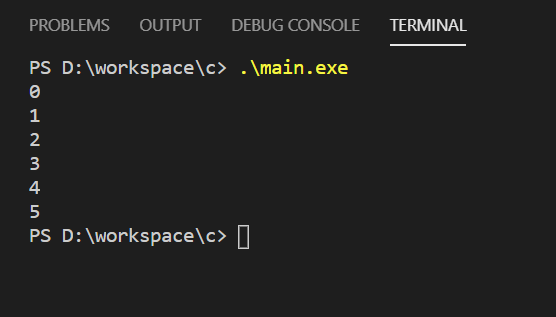
Conclusion
In this C Tutorial, we learned about C Do-While Loop statement.