C For Loop
C For loop statement executes a block of statements repeatedly in a loop based on a condition.
C For loop differs from While Loop in syntax. Initialization and Update are part of the syntax in for loop. Otherwise, in most of the cases, you can do the same task that a for loop does, using a while loop.
In this tutorial, we will learn the syntax of for loop, its execution flow using flow diagram, and its usage using example programs.
Syntax of C For Statement
The syntax of For loop statement in C language is given below.
for (initialization; condition; update) { //for block statement(s) }
where
for is C builtin keyword.
initialization part can contain variable initialization. The variables declared here have a scope of this for loop and therefore are visible only in this for loop. You can declare and initialize one or more variables of same type in initialization.
condition is a boolean value or an expression that evaluates to boolean value. The condition is generally formed using comparison operators and logical operators.
update statement can contain comma separated assignment statements or arithmetic operations like increment, decrement.
() parenthesis surround the initialization, condition and update.
for block statement(s) is a set of statements. This block of statement(s) is also called for block, in short. You can have none, one or multiple statements inside for block.
{} flower braces wrap around for block statement(s). These are optional only in case that there is only one statement inside for block. Otherwise, these braces are mandatory.
Flow Diagram of C For Loop Statement
The flow of execution for a C For Loop statement is given in the following flow diagram.
The for statement execution starts with evaluating the condition. If the condition evaluates to true, the program executes the statement(s) inside for block, and goes to the condition again. But, if the condition evaluates to false, during any iteration, the for loop execution is completed, and the program continues with the execution of subsequent statements present after for statement.
Example for C For Statement
We already know that C For is a looping statement. It contains initialization, condition, and update parts based on which a decision is made whether to execute statement(s) inside for block or not.
In the following program, we have a for loop with initial section initializing a variable i to zero, condition that checks whether value store in variable i is less than 10. If the number is less than 10, then we shall print print the number, increment i it in update section, and continue with next iteration.
C Program
#include <stdio.h> void main() { for (int i = 0; i < 10; i++) { printf("%d\n", i); } }
Here the condition is i < 10
. It is a boolean expression and it evaluates to either true or false. During each iteration i is updating, and when i becomes 10
, the for loop breaks.
Output
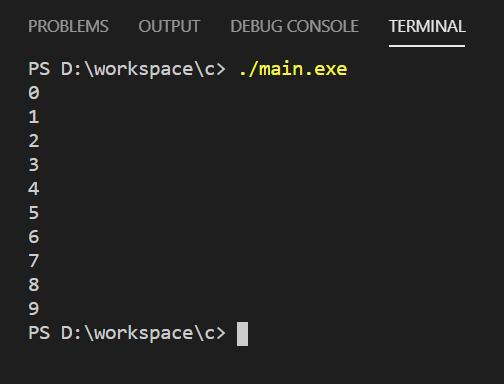
Break C For Loop
You can break a for loop in C, using break statement. When break statement executes, the surrounding loop is deemed completed and the control comes out of the for loop.
In the following example, we try to print the numbers from 0 to 9, as in the previous example. But when the number is 5, we break the for loop.
C Program
#include <stdio.h> void main() { for (int i = 0; i < 10; i++) { if (i == 5) { break; } printf("%d\n", i); } }
Output
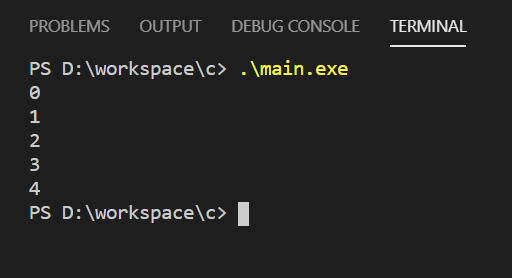
Continue C For Loop
You may skip the execution of steps inside for block and continue with next iterations using continue statement. continue statement takes the execution to update section of for loop, which is the next logical step after for block.
C Program
#include <stdio.h> void main() { for (int i = 0; i < 10; i++) { if (i == 5) { continue; } printf("%d\n", i); } }
Output
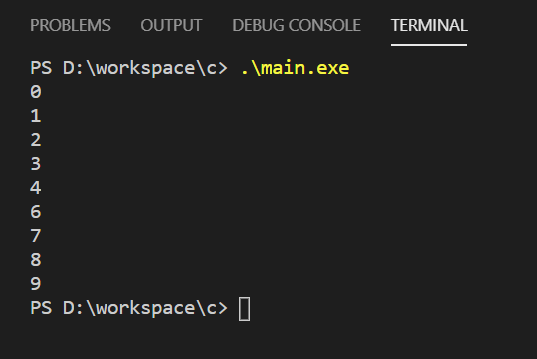
Nested C For Loop
For Loop is just like another C statement. So, in the for block, you can have a for loop statement. This process of writing a for loop inside a for loop is called For Loop Nesting.
C Program
#include <stdio.h> void main() { for (int i = 0; i <= 5; i++) { for (int j = 0; j <= i; j++) { printf("%d ", j); } printf("\n"); } }
Output
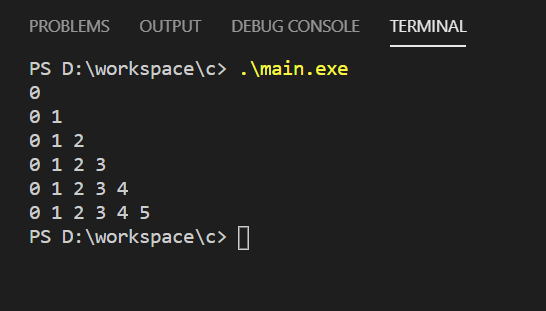
Conclusion
In this C Tutorial, we learned what a For Loop statement is in C programming language, how its execution happens, and how it works with example programs.