C If statement
C If statement lets programmers to execute a block of statements based on a condition. Condition is a boolean expression which evaluates to either true or false.
Syntax of C If Statement
The syntax of If statement in C language is given below.
if (condition) { //statement(s) }
where
if is C builtin keyword.
condition is a boolean value or an expression that evaluates to boolean value. The condition is generally formed using comparison operators and logical operators.
() parenthesis around the condition is mandatory.
statement(s) is a set of statements. This block of statement(s) is also called if block. You can have none, one or multiple statements inside if block.
{} flower braces wrap around if block statement(s). These are optional only in case that there is only one statement inside if block. Otherwise, these braces are mandatory.
Flow Diagram of C IF Statement
Following diagram represents the flow of execution for a C if statement.
The if-statement execution starts with evaluating the condition. If the condition evaluates to true, the program executes the statement(s) inside if block. But, if the condition evaluates to false, the if-statement execution is completed, and the program continues with the execution of subsequent statements present after if-statement.
Example for C If Statement
We already know that C If statement is a decision making statement. It contains a condition based on which a decision is made whether to execute statement(s) inside if block or not.
In the following program, we have a condition that checks whether the number is even. If the number is even, then we shall print a message to the console that the number is even.
C Program
#include <stdio.h> void main() { int a; printf("Enter Number : "); scanf("%d", &a); if (a%2 == 0) { printf("You entered an even number"); } }
Here the condition is a%2 == 0
. It is a boolean expression and it evaluates to either true or false. If it is true, then the print statement inside if block is executed.
Output
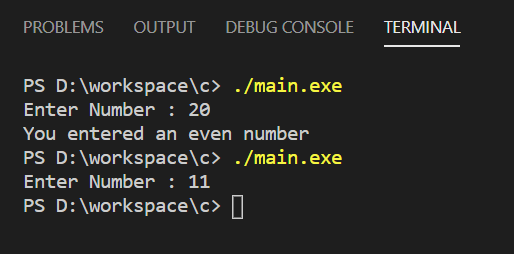
Conclusion
In this C Tutorial, we learned what an If statement is in C programming language, how its execution happens, and how it works with an example program.