C While Loop
C While loop statement lets programmers to execute a block of statements repeatedly in a loop based on a condition. Condition is a boolean expression which evaluates to either true or false.
In this tutorial, we will learn the syntax of while loop, its execution flow using flow diagram, and its usage using example programs.
Syntax of C While Statement
The syntax of While loop statement in C language is given below.
while (condition) { //statement(s) }
where
while is C builtin keyword.
condition is a boolean value or an expression that evaluates to boolean value. The condition is generally formed using comparison operators and logical operators.
() parenthesis around the condition is mandatory.
statement(s) is a set of statements. This block of statement(s) is also called while block. You can have none, one or multiple statements inside while block.
{} flower braces wrap around while block statement(s). These are optional only in case that there is only one statement inside while block. Otherwise, these braces are mandatory.
Flow Diagram of C While Statement
The flow of execution for a C for statement is given in the following flow diagram.
The while statement execution starts with evaluating the condition. If the condition evaluates to true, the program executes the statement(s) inside while block, and goes to the condition again. But, if the condition evaluates to false, during any iteration, the while loop execution is completed, and the program continues with the execution of subsequent statements present after while statement.
Example for C While Statement
We already know that C While statement is a looping statement. It contains a condition based on which a decision is made whether to execute statement(s) inside while block or not.
In the following program, we have a condition that checks whether the number is less than some value stored in a. If the number is less than a, then we shall print print the number, increment it, and continue with next iteration.
C Program
#include <stdio.h> void main() { int a = 10; int number = 0; while (number < a) { printf("%d\n", number); number++; } }
Here the condition is number < a
. It is a boolean expression and it evaluates to either true or false. During each iteration number is changing, and when number becomes 10
, the while loop breaks.
Output
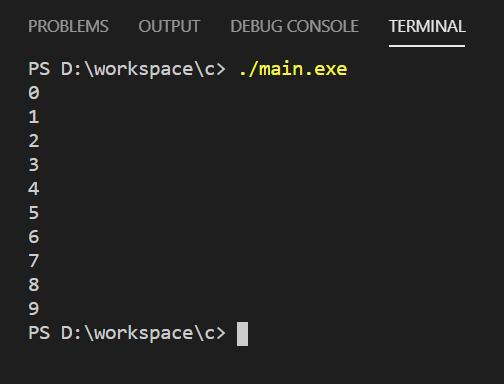
Break C While Loop
You can break a while loop in C, using break statement. When break statement executes, the surrounding loop is deemed completed and the control comes out of the while loop.
In the following example, we try to print the numbers from 0 to 9, as in the previous example. But when the number is 5, we break the for loop.
C Program
#include <stdio.h> void main() { int a = 10; int number = 0; while (number < a) { if (number == 5) { break; } printf("%d\n", number); number++; } }
Output
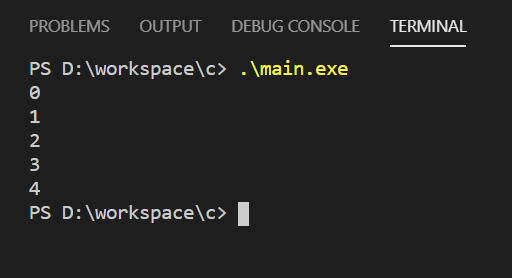
Continue C While Loop
You may skip the execution of steps inside while block and continue with next iterations using continue statement. continue statement takes the execution to evaluate while condition.
C Program
#include <stdio.h> void main() { int a = 10; int number = 0; while (number < a) { if (number == 5) { number++; continue; } printf("%d\n", number); number++; } }
Output
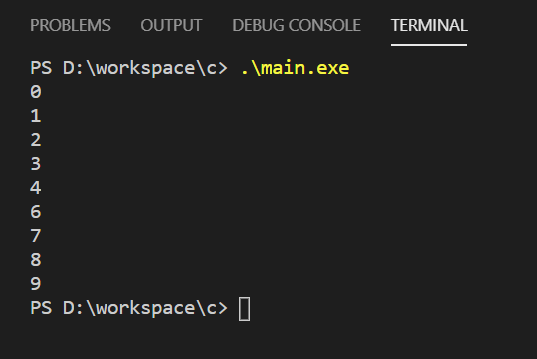
Nested C While Loop
While Loop is just like another C statement. So, in the while loop body, you can have a while loop statement. This process of writing a while loop inside a while loop is called While Loop Nesting.
C Program
#include <stdio.h> void main() { int i = 0; while (i <= 5) { int j = 0; while (j <= i) { printf("%d ", j); j++; } printf("\n"); i++; } }
Output
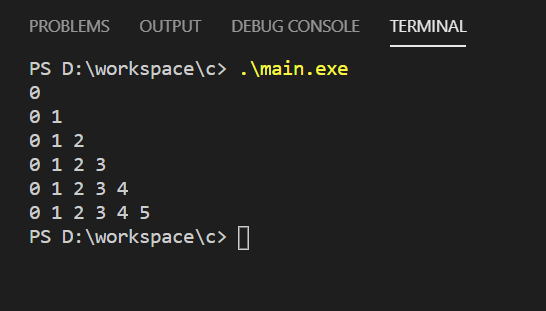
Conclusion
In this C Tutorial, we learned what a While Loop statement is in C programming language, how its execution happens, and how it works with an example program.