Rename File in C Programming
To rename a file in C, use rename() function of stdio.h. rename() function takes the existing file name and new file names as arguments and renames the file. rename() returns 0 if the file is renamed successfully, else it returns a non-zero value.
In this tutorial, we will use stdio.h’s rename() function and rename a file.
The syntax of rename() function is
int rename(const char * oldname, const char * newname);
Example 1 – Rename File
In the following example, we rename a file named “welcome.txt” to “readme.txt” in the current working directory. But if the file is not present in the current directory, you may have to provide relative path or absolute path to rename() function. Also, note that the file name should include the extension as well.
Following screenshot shows the location of the file we are renaming. It is right next to our main.exe file.
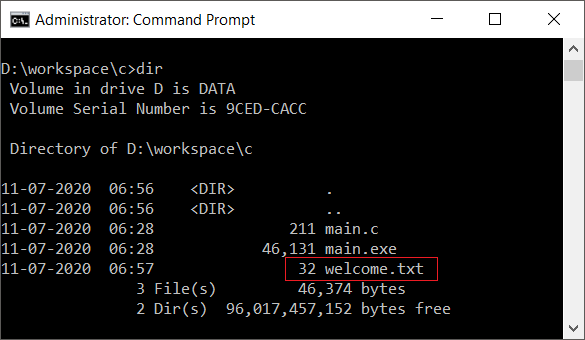
C Program
#include <stdio.h> int main() { int result = rename("welcome.txt", "readme.txt"); if (result == 0) { printf("The file is renamed successfully."); } else { printf("The file could not be renamed."); } return 0; }
Output
PS D:\workspace\c> .\main.exe The file is renamed successfully.
Following screenshot shows that the file has been renamed.
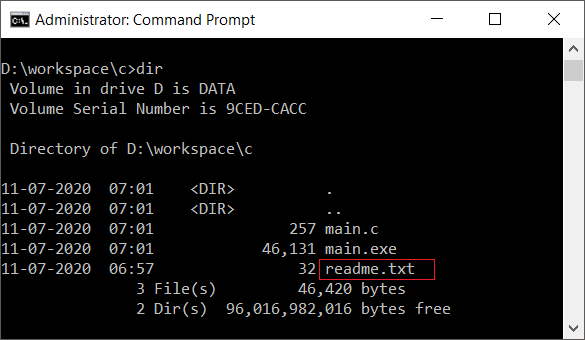
Example 2 – Rename File in C [Negative Scenario]
In this example, we shall try to rename a file that is not present. If we try to rename a file that is not present using rename() function, the function should return a non-zero value.
In the previous example, we renamed “welcome.txt”. Let us try again renaming the same file. As the file is already renamed, the file with the name “welcome.txt” should not be present in the location, unless you create one again.
C Program
#include <stdio.h> int main() { int result = rename("welcome.txt", "readme.txt"); if (result == 0) { printf("The file is renamed successfully."); } else { printf("The file could not be renamed."); } return 0; }
Output
PS D:\workspace\c> .\main.exe The file could not be renamed.
Usually, there could be many reasons when a file is not renamed. You may have to check the possible scenarios.
Conclusion
In this C Tutorial, we have learnt to rename a file in C.