Delete File in C Programming
To delete a file using C language, use remove() function of stdio.h. remove() function takes file name (or path if not located in the same location) as argument and deletes the file. remove() returns 0 if the file is deleted successfully, else it returns a non-zero value.
In this tutorial, we will use stdio.h’s remove() function and delete a file.
Please not that when file is deleted using remove() function, the file will not be moved to Recycle Bin or Trash, but the file is deleted permanently.
The syntax of remove() function is
int remove(const char * filename);
Example – Delete File
In the following example, we delete a file named “welcome.txt” from the current directory. But if the file is not present in the current directory, you may have to provide relative path or absolute path to remove() function. Also, note that the file name should include the extension as well.
Following screenshot shows the location of the file we are deleting. It is right next to our main.exe file.
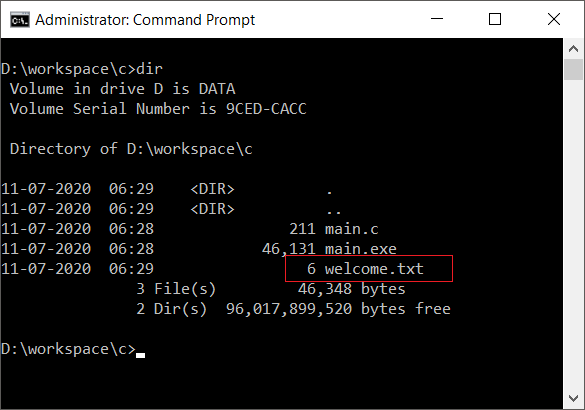
C Program
#include <stdio.h> int main() { if (remove("welcome.txt") == 0) { printf("The file is deleted successfully."); } else { printf("The file is not deleted."); } return 0; }
Output
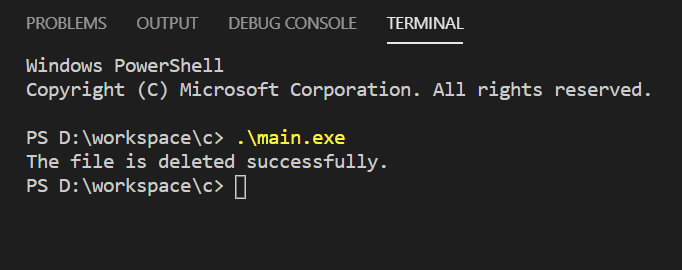
Example – C delete file [Negative Scenario]
In this example, we shall try to delete a file that is not present. If we try to delete a file that is not present using remove() function, we should get a non-zero value as return value.
In the previous example, we deleted welcome.txt. Let us try again deleting the same file. As the file is already deleted, it should not be present in the location, unless you create one again.
C Program
#include <stdio.h> int main() { if (remove("welcome.txt") == 0) { printf("The file is deleted successfully."); } else { printf("The file is not deleted."); } return 0; }
Output
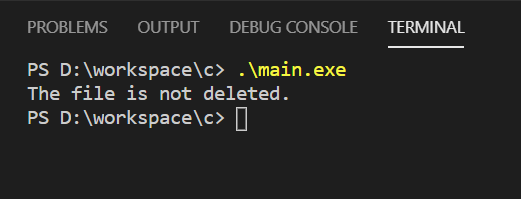
There could be many reasons when a file is not deleted. You may have to check the possible scenarios.
Conclusion
In this C Tutorial, we have learnt to delete a file using C programs.