JavaFX DatePicker
JavaFX DataPicker in GUI allows user to enter a value for the date or click on the calendar icon and choose a date from the popup.
In this tutorial, we will learn how to initialize a DatePicker control and show it in GUI, then add an action listener to get the value picked by user using DatePicker.
Example 1 – Basic JavaFX DatePicker
Following is a basic example to demonstrate how to initialize date picker in JavaFX using DatePicker class and show it on a scene.
JavaFxDatePickerTutorial.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxDatePickerTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("JavaFX Date Picker - tutorialkart.com");
//javafx date picker
DatePicker datePicker = new DatePicker();
// tile pane
TilePane tilePane = new TilePane();
// add date picker to the tile pane
tilePane.getChildren().add(datePicker);
//set up scene
Scene scene = new Scene(tilePane, 400, 400);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this Java application and you should see a DatePicker as shown below, of course without the popup. When you click on the calendar icon, you will get the popup. You can change the month or year by clicking on the left or right arrows and then choose a date by clicking on the day of month.
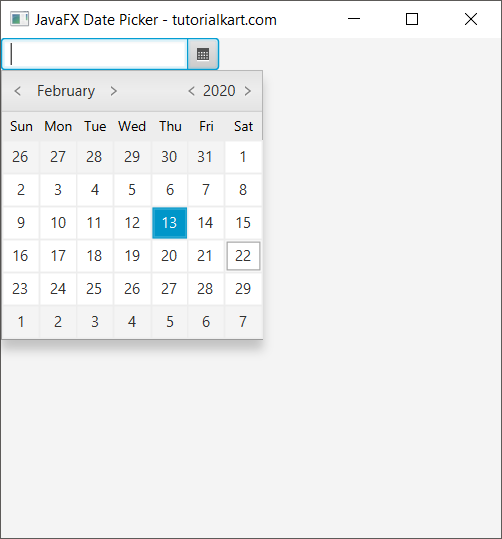
When you click on a date, the popup disappears and the chosen date appears in the date picker field.
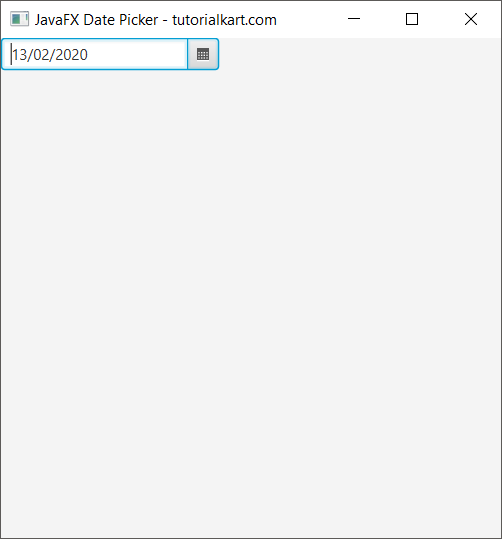
Example 2 – JavaFX DatePicker – Get Value
In this example, we will set up an action listener to the DatePicker. The callback function is called when there is a change in the value of DatePicker object.
JavaFxDatePickerTutorial.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.DatePicker;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxDatePickerTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("JavaFX Date Picker - tutorialkart.com");
//javafx date picker
DatePicker datePicker = new DatePicker();
//add action listener to the date picker
datePicker.setOnAction(action -> {
System.out.println("Date Picked: "+datePicker.getValue());
});
// tile pane
TilePane tilePane = new TilePane();
// add date picker to the tile pane
tilePane.getChildren().add(datePicker);
//set up scene
Scene scene = new Scene(tilePane, 400, 400);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
When you select a date from the pop, you should see a date filled up in the date picker field.
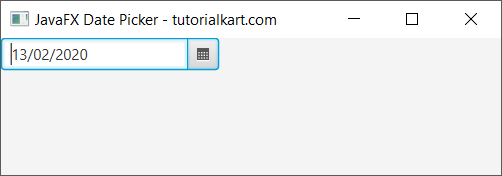
In the console, you will see the date printed as shown below.
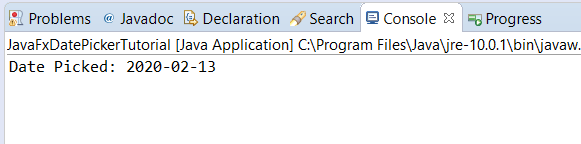
If you click the calendar icon and click on the same date as that of previously selected, the callback function of action listener is not called. The callback function is called only when you select a different date.
Conclusion
In this JavaFX Tutorial, we learned how to define a JavaFX DatePicker in GUI, and how to set action listener for DatePicker.