JavaFX Tutorial – We shall learn to Create new Button and Set Action Listener in JavaFX Application.
Create new Button and Set Action Listener in JavaFX
Following is a quick code snippet to create a Button and set action listener to this Button.
Quick Code Snippet
Button btn = new Button(); btn.setText("Display Message"); btn.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { System.out.println("Hi there! You clicked me!"); } });
Following is a step by step guide to create a new Button in JavaFX and Set Action Listener.
1. Create a JavaFX Button
Import javafx.scene.control.Button class and create a new object of this class type.
Button btn = new Button();
2. Button Text
Set text for the JavaFX Button using Button.setText() method.
btn.setText("Display Message");
3. Set Action Listener
If you have set an Action Listener and the button is clicked, EventHandler would execute the handle method. You should implement the handle method to perform an action after an event happens for button.
btn.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { System.out.println("Hi there! You clicked me!"); } });
4. Run the JavaFX Application.
Following is the complete program to set action listener to JavaFX Button.
NewButtonExample.java
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class NewButtonExample extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { try { // set title primaryStage.setTitle("New Button and its Action Listener"); // create a new Button shape Button btn = new Button(); // set text inside button btn.setText("Display Message"); // set Action Listener btn.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { // instructions executed when the button is clicked System.out.println("Hi there! You clicked me!"); } }); // stack pane StackPane root = new StackPane(); // add button to Stack Pane root.getChildren().add(btn); Scene scene = new Scene(root,400,400); scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm()); primaryStage.setScene(scene); primaryStage.show(); } catch(Exception e) { e.printStackTrace(); } } }
GUI Output
Following would be the output on running the JavaFX Application.
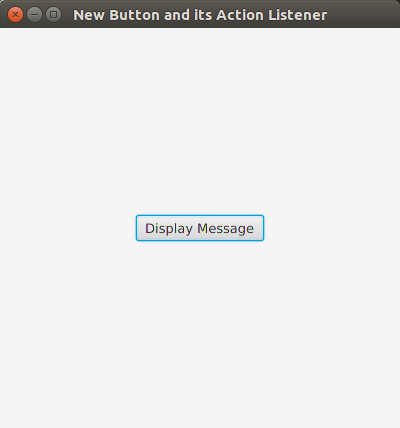
If you click on the button ‘Display message’, ‘Hi there! You clicked me!’ would be printed to console output. You may replace the code in handle() method to perform your own defined action.
Console Output
Hi there! You clicked me!
Conclusion
In this JavaFX Tutorial : Create new Button and Set Action Listener in JavaFX , we have learnt to create a new button with desired text and trigger an action when the button is clicked.