JavaFX Slider
JavaFX Slider is a control that can be used to select a value within a interval using a head on a sliding bar. The position of the head on the slider represents the value of the Slider.
Following is an example of how slider looks in a window.
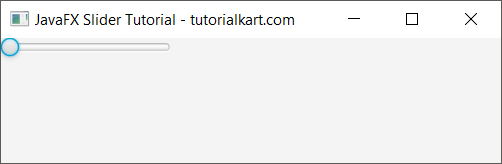
In this tutorial, we will learn the following things.
- Display Slider in window
- Add Listener to Slider and Get Slider value when the slider value changes
Example 1 – JavaFX Slider Basic Example
Following a basic JavaFX Slider example, where we shall display the slider in a window.
JavaFxSliderTutorial.java
import javafx.application.Application; import javafx.event.Event; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Slider; import javafx.scene.control.TextField; import javafx.scene.layout.TilePane; import javafx.stage.Stage; public class JavaFxSliderTutorial extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { try { // set title primaryStage.setTitle("JavaFX Slider Tutorial - tutorialkart.com"); //javafx text field Slider slider = new Slider(0, 100, 0); // tile pane TilePane tilePane = new TilePane(); // add slide to the tile pane tilePane.getChildren().add(slider); //set up scene Scene scene = new Scene(tilePane, 400, 100); primaryStage.setScene(scene); primaryStage.show(); } catch(Exception e) { e.printStackTrace(); } } }
Run this JavaFX application and you shall get the following UI window displayed.
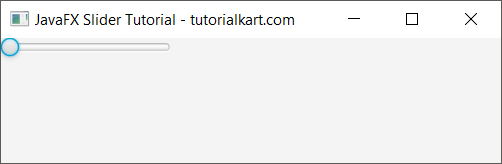
Example 2 – Get JavaFX Slider value on change
JavaFxSliderTutorial.java
import javafx.beans.value.ChangeListener; import javafx.application.Application; import javafx.beans.value.ObservableValue; import javafx.scene.Scene; import javafx.scene.control.Slider; import javafx.scene.layout.TilePane; import javafx.stage.Stage; public class JavaFxSliderTutorial extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { try { // set title primaryStage.setTitle("JavaFX Slider Tutorial - tutorialkart.com"); //javafx text field Slider slider = new Slider(0, 100, 1); slider.valueProperty().addListener(new ChangeListener<Number>() { public void changed( ObservableValue<? extends Number> observableValue, Number oldValue, Number newValue) { System.out.println(slider.getValue()); } }); // tile pane TilePane tilePane = new TilePane(); // add slide to the tile pane tilePane.getChildren().add(slider); //set up scene Scene scene = new Scene(tilePane, 400, 100); primaryStage.setScene(scene); primaryStage.show(); } catch(Exception e) { e.printStackTrace(); } } }
Run this application, and you will get a UI window as in the previous example. If you start sliding the head on the slider, you will see that the slider value is printed to the console. You can use this value as per your application requirement.
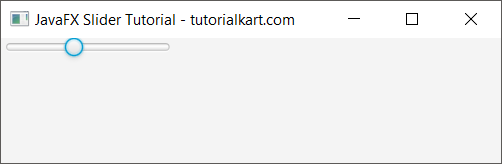
Following is the sample of console, with slider value printed out.
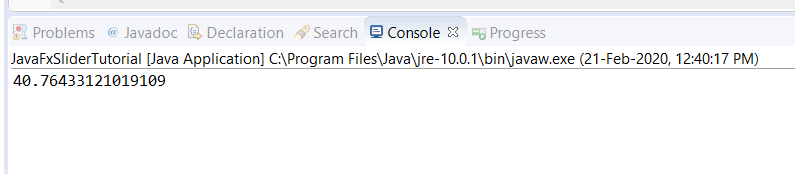
Conclusion
In this JavaFX Tutorial, we learned how to show slider in our GUI, add a listener to it and get the value of it when you change the slider position.