JavaFX RadioButton
Radio Buttons are generally used to select one among many options. In JavaFX, RadioButton is a type of ToggleButton. We can realize that only one among many radio buttons can be selected by using ToggleGroup.
In this tutorial, we will learn how to display RadioButton in our GUI application, then to define many Radio buttons and finally how to group them together using ToggleGroup with examples.
Example 1 – JavaFX RadioButton Basic Example
JavaFxRadioButtonTutorial2.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.RadioButton;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxRadioButtonTutorial2 extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("Radio Button Tutorial - tutorialkart.com");
//javafx radio button
RadioButton radioButton1 = new RadioButton("Option 1");
// tile pane
TilePane tilePane = new TilePane();
// add radio button to the tile pane
tilePane.getChildren().add(radioButton1);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this application and RadioButton should be displayed as shown in the following screenshot. A round radio button appears with the label provided to RadioButton() constructor.
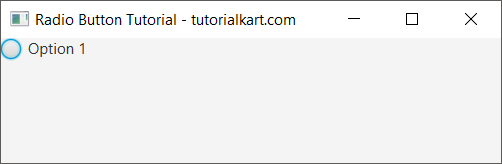
Example 2 – More Radio Buttons
In this example, we shall try to display multiple radio buttons.
JavaFxRadioButtonTutorial3.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxRadioButtonTutorial3 extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("Radio Button Tutorial - tutorialkart.com");
//javafx radio buttons
RadioButton radioButton1 = new RadioButton("Option 1");
RadioButton radioButton2 = new RadioButton("Option 2");
RadioButton radioButton3 = new RadioButton("Option 3");
// tile pane
TilePane tilePane = new TilePane();
// add radio buttons to the tile pane
tilePane.getChildren().add(radioButton1);
tilePane.getChildren().add(radioButton2);
tilePane.getChildren().add(radioButton3);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this Java application, and try to click on multiple radio buttons.
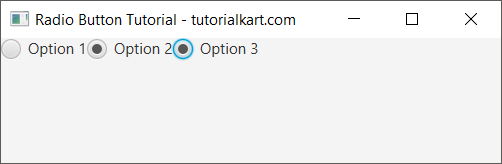
This is not what we expect, when we group some radio buttons. User should be able to select only one of these radio buttons. For that to happen, we have to group them. This grouping is demonstrated in the next example.
Example 3 – Group of JavaFX RadioButtons
Adding RadioButtons to ToggleGroup makes it possible to select only one of the option belonging to that toggle group.
JavaFxRadioButtonTutorial.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxRadioButtonTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("Radio Button Tutorial - tutorialkart.com");
//javafx radio buttons
RadioButton radioButton1 = new RadioButton("Option 1");
RadioButton radioButton2 = new RadioButton("Option 2");
RadioButton radioButton3 = new RadioButton("Option 3");
//a group for radio buttons
ToggleGroup radioGroup = new ToggleGroup();
//to group radio buttons
radioButton1.setToggleGroup(radioGroup);
radioButton2.setToggleGroup(radioGroup);
radioButton3.setToggleGroup(radioGroup);
// tile pane
TilePane tilePane = new TilePane();
// add radio buttons to the tile pane
tilePane.getChildren().add(radioButton1);
tilePane.getChildren().add(radioButton2);
tilePane.getChildren().add(radioButton3);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
When you run this Java program, the radio buttons are displayed as shown below.
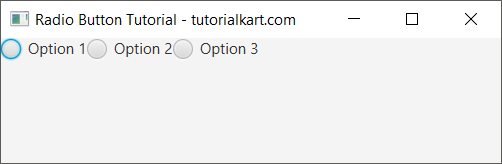
Try clicking on different options. The previous selection is cleared and the new chosen option is selected. The option you clicked on appears with a black disc inside the circle before label.
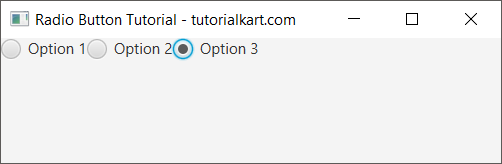
Example 4 – Check which JavaFX Radio Button is Clicked
You can check which radio button is chosen.
JavaFxRadioButtonTutorial4.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxRadioButtonTutorial4 extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("Radio Button Tutorial - tutorialkart.com");
//javafx radio buttons
RadioButton radioButton1 = new RadioButton("Option 1");
RadioButton radioButton2 = new RadioButton("Option 2");
RadioButton radioButton3 = new RadioButton("Option 3");
//to group radio buttons
ToggleGroup radioGroup = new ToggleGroup();
//add buttons to the group
radioButton1.setToggleGroup(radioGroup);
radioButton2.setToggleGroup(radioGroup);
radioButton3.setToggleGroup(radioGroup);
Button button = new Button("Submit");
button.setOnAction(action -> {
System.out.println("Is option 1 selected: "+radioButton1.isSelected());
System.out.println("Is option 2 selected: "+radioButton2.isSelected());
System.out.println("Is option 3 selected: "+radioButton3.isSelected());
});
// tile pane
TilePane tilePane = new TilePane();
// add all controls to the tile pane
tilePane.getChildren().add(radioButton1);
tilePane.getChildren().add(radioButton2);
tilePane.getChildren().add(radioButton3);
tilePane.getChildren().add(button);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run the Java application, click on a Radio Button, and then click on Submit button.
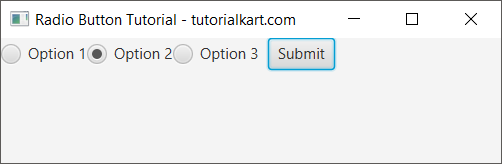
When you click on the Submit button, we shall check if each of the radio button is selected using isSelected(). We will get true returned only for the radio button the user has selected as shown in the sample console output below.

Example 5 – Action listener for a JavaFX RadioButton
You can add an Action listener for a RadioButton. And this listener callback is called when you click and release on the RadioButton,
In this example, we shall define a RadioButton and add an action listener to it. Whenever user clicks on the radio button, whether selects it or unselects it, we shall print if the radio button is selected.
JavaFxRadioButtonTutorial5.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.RadioButton;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxRadioButtonTutorial5 extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("Radio Button Tutorial - tutorialkart.com");
//javafx radio button
RadioButton radioButton1 = new RadioButton("Option 1");
radioButton1.setOnAction(action -> {
System.out.println("Option 1 selected: "+radioButton1.isSelected());
});
// tile pane
TilePane tilePane = new TilePane();
// add radio button to the tile pane
tilePane.getChildren().add(radioButton1);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this Java application and you will get a radio button as displayed in the first example of this tutorial. Click on the radio button and you should something like following in the console.
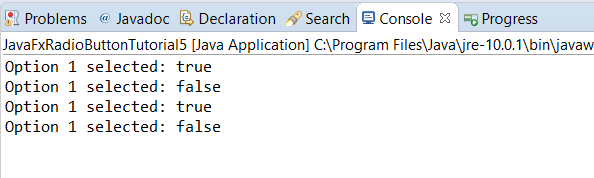
Conclusion
In this JavaFX Tutorial, we learned how to display a RadioButton control on GUI, group some radio buttons together, and get the selection.