JavaFX TextField
JavaFX TextField class can be used to provide a provision for the user to enter some text, and then the program can read the value entered by the user programmatically.
To create a new TextField, use TextField class with new keyword as shown below.
TextField textField = new TextField();
Now you can add this TextField to a pane. For example, to add TextField to TilePane, following is the code.
tilePane.getChildren().add(textField);
You can read the text entered in the TextField programmatically using getText() method. Usually, you use this statement when an event occurs, like when user clicks a button after entering all the text fields, something like that.
String text = textField.getText();
Example 1 – JavaFX TextField
In the following example, we shall initialize a TextField, along with a button. When the button is clicked, we shall read the value entered in TextField.
JavaFxTextFieldTutorial.java
import javafx.application.Application;
import javafx.event.Event;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxTextFieldTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("TextField Tutorial");
//javafx text field
TextField textField = new TextField();
Button btn = new Button();
btn.setText("Submit");
btn.setOnAction(new EventHandler() {
@Override
public void handle(Event arg0) {
System.out.println(textField.getText());
}
});
// stack pane
TilePane tilePane = new TilePane();
// add TextField and Button to the tilepage
tilePane.getChildren().add(textField);
tilePane.getChildren().add(btn);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run the application and you should see the following UI window generated.
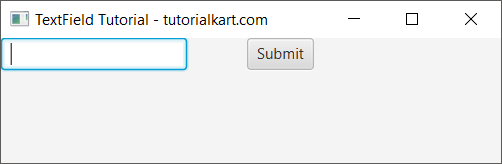
Enter some text in the text field and click on Submit button as shown below.
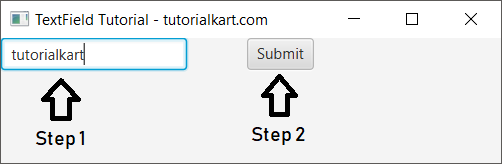
In the event handler of the button, we have written print statement to print the value entered in the TextField to console. So, after you click on the Submit button, you should see the value printed to the console as shown in the below screenshot.
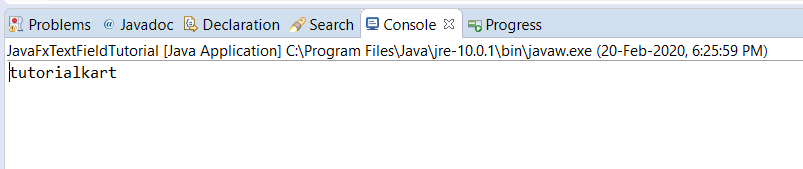
Example 2 – Set value to JavaFX TextField
You can also set value to JavaFX TextField using the constructor as shown below.
JavaFxTextFieldTutorial.java
import javafx.application.Application;
import javafx.event.Event;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxTextFieldTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("TextField Tutorial - tutorialkart.com");
//javafx text field
TextField textField = new TextField("tutorialkart");
// stack pane
TilePane tilePane = new TilePane();
// add TextField to the tilepage
tilePane.getChildren().add(textField);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
When you run this application, you should see the TextField with the value provided in the constructor TextField(), being set in the UI window, as shown below.
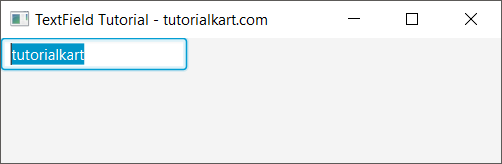
You can also set a string for the text of TextField using TextField.setText() as shown in the below program.
JavaFxTextFieldTutorial.java
import javafx.application.Application;
import javafx.event.Event;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxTextFieldTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("TextField Tutorial - tutorialkart.com");
//javafx text field
TextField textField = new TextField();
//set text
textField.setText("tutorialkart");
// stack pane
TilePane tilePane = new TilePane();
// add TextField to the tilepage
tilePane.getChildren().add(textField);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Conclusion
In this JavaFX Tutorial, we learned how to initialize a JavaFX TextField, read the value entered in TextField, and set a value to TextField.