JavaFX Label
JavaFX Label class can be used to display a text label or image label inside a JavaFX Scene.
In this tutorial, we will learn how to display a JavaFX Label in your GUI application.
Following is a quick code snippet of how to create a JavaFX Label.
Label label = new Label("TutorialKart");
You have to import javafx.scene.control.Label
to use JavaFX Label.
Example 1 – JavaFX Label with Text
In the following example JavaFX application, we have created a JavaFX Label and added it to a scene to display it on the GUI window.
JavaFxLabelTutorial.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxLabelTutorial extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("JavaFX Label - tutorialkart.com");
//javafx label
Label label = new Label("TutorialKart");
// tile pane
TilePane tilePane = new TilePane();
// add label to the tile pane
tilePane.getChildren().add(label);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this application and you should see a Label with the string “TutorialKart” in the window, as shown below.
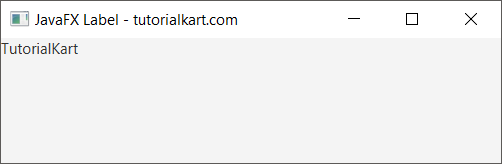
Example 2 – JavaFX Label with Text and Image
JavaFX Label constructor can accept ImageView as a second argument and display image and label in GUI.
In the following example JavaFX application, we have created a JavaFX ImageView and passed it to JavaFX Label. We shall see how does Label displays text and image in GUI.
JavaFxLabelTutorial2.java
import java.io.FileInputStream;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavaFxLabelTutorial2 extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
try {
// set title
primaryStage.setTitle("JavaFX Label - tutorialkart.com");
//image
FileInputStream input = new FileInputStream("images/camera2.png");
Image image = new Image(input);
ImageView imageView = new ImageView(image);
//javafx label
Label label = new Label("TutorialKart", imageView);
// tile pane
TilePane tilePane = new TilePane();
// add label to the tile pane
tilePane.getChildren().add(label);
//set up scene
Scene scene = new Scene(tilePane, 400, 100);
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
}
Run this application and you should see a Label with the text “TutorialKart” and the image before the text in the window, as shown below.
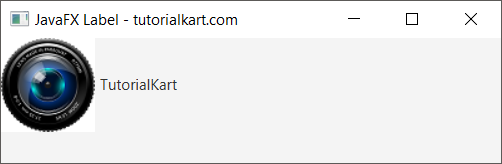
Conclusion
In this JavaFX Tutorial, we learned how to display text in GUI window. Also, we have seen an example, where we can provide an image to display before the text.