C# Substring
To get Substring of a string in C#, use Substring function on the string.
string.Substring(int startIndex, int length)
where
startIndex
is the index position in the string from which substring starts.
length
is the length of the substring.
length
is optional. So, if you provide only startIndex
, the substring starting from startIndex
till the end of given string is returned by the Substring.
Example 1 – C# Sustring – Using startIndex
In the following example, we will find the substring with startIndex.
Program.cs
using System; namespace CSharpExamples { class Program { static void Main(string[] args) { string str = "HelloWorld"; int startIndex = 4; string substring = str.Substring(startIndex); Console.WriteLine("Substring is : "+substring); } } }
Output
PS D:\workspace\csharp\HelloWorld> dotnet run Substring is : oWorld
ADVERTISEMENT
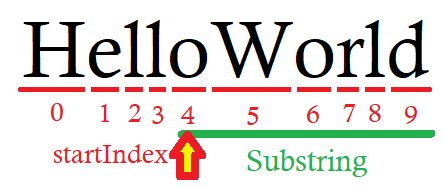
Example 2 – C# Sustring – Using startIndex and length
In the following example, we will find the substring with startIndex.
Program.cs
using System; namespace CSharpExamples { class Program { static void Main(string[] args) { string str = "HelloWorld"; int startIndex = 4; int length = 3; string substring = str.Substring(startIndex, length); Console.WriteLine("Substring is : "+substring); } } }
Output
PS D:\workspace\csharp\HelloWorld> dotnet run Substring is : oWo
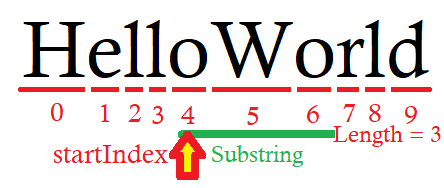
Example 3 – C# Sustring
In the following example, we will read the string, startIndex and length from the user and then find the substring.
Program.cs
using System; namespace CSharpExamples { class Program { static void Main(string[] args) { Console.Write("Enter a string : "); string str = Console.ReadLine(); Console.Write("Enter startIndex : "); int startIndex = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter length : "); int length = Convert.ToInt32(Console.ReadLine()); string substring = str.Substring(startIndex, length); Console.WriteLine("Substring is : "+substring); } } }
Output
PS D:\workspace\csharp\HelloWorld> dotnet run Enter a string : Welcome to TutorialKart for C# Tutorial. Enter startIndex : 5 Enter length : 12 Substring is : me to Tutori PS D:\workspace\csharp\HelloWorld> dotnet run Enter a string : TutorialKart Enter startIndex : 5 Enter length : 3 Substring is : ial
Summary
In this C# Tutorial, we learned to find substring of a string with the help of examples.