Business Application Programming Interface (BAPI) provides a standardized programming interface that enables external applications to interact with SAP systems. Utilizing BAPIs for creating customer inquiries streamlines the process, ensuring consistency and efficiency without the need for extensive ABAP coding. This guide outlines the professional approach to creating a customer inquiry using BAPI in SAP.
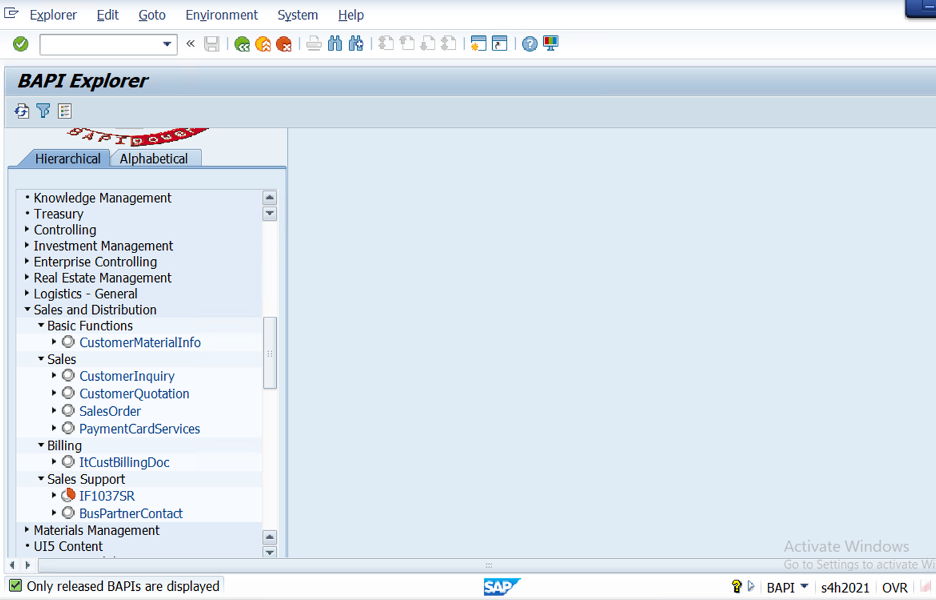
Prerequisites
Before proceeding, ensure that you have:
- SAP Access: Appropriate permissions to access transaction codes and execute BAPIs.
- Development Environment: Access to SAP’s ABAP development environment (SE37, SE84, SE38).
- Knowledge of SAP SD Module: Understanding of Sales and Distribution processes within SAP.
Step 1: Identify the Appropriate BAPI
The first step involves selecting the correct BAPI to create a customer inquiry. For creating customer inquiries, the recommended BAPI is:
- BAPI_INQUIRY_CREATEFROMDATA2
Procedure:
- Navigate to Transaction Code BAPI:
- Enter
BAPI
in the SAP transaction code field to open the BAPI Explorer.
- Search for the BAPI:
- Use the search functionality to locate
BAPI_INQUIRY_CREATEFROMDATA2
. - Ensure you select the latest version of the BAPI by choosing the method with the highest numerical suffix (e.g.,
BAPI_INQUIRY_CREATEFROMDATA2
is preferred over older versions).
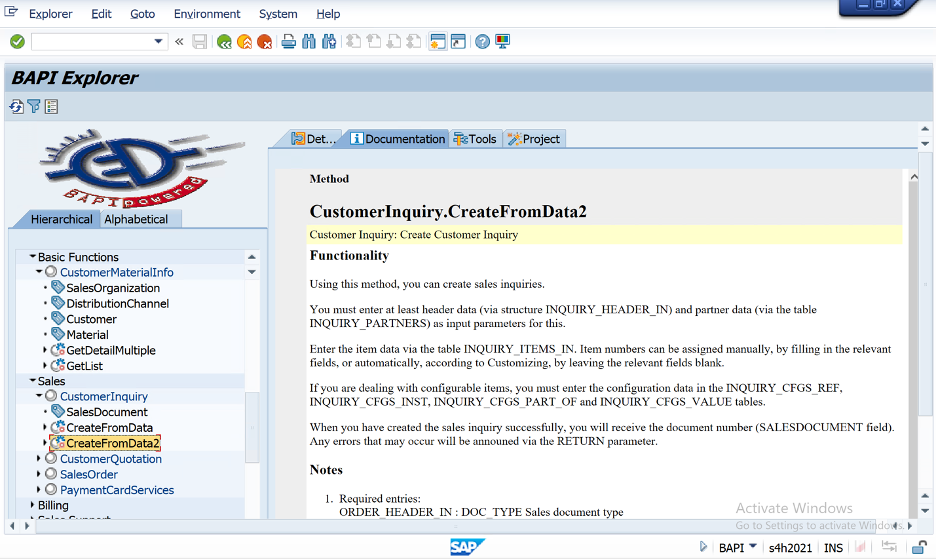
Step 2: Access and Understand BAPI Documentation
Understanding the BAPI’s functionality and requirements is crucial before implementation.
Procedure:
- Open BAPI Documentation:
- Go to transaction code
SE37
. - Enter the BAPI name
BAPI_INQUIRY_CREATEFROMDATA2
and press Enter.
- Review Documentation:
- Thoroughly read the BAPI’s documentation to comprehend its functionality, input parameters, and expected outputs.
- Pay special attention to the required input structures such as
INQUIRY_HEADER_IN
,INQUIRY_PARTNERS
, andINQUIRY_ITEMS_IN
.
- Understand Input Parameters:
- Header Data: Contains overarching information about the inquiry.
- Item Data: Details about each item included in the inquiry.
- Partner Data: Information about the business partners involved (e.g., sold-to party, ship-to party).
Step 3: Define the Required Parameters
Properly defining and structuring the input parameters is essential for the successful creation of a customer inquiry.
Required Entries:
- Header Data (
INQUIRY_HEADER_IN
):
- DOC_TYPE: Sales document type (e.g., ‘IN’ for inquiry).
- SALES_ORG: Sales organization identifier (e.g., ‘1000’).
- DISTR_CHAN: Distribution channel (e.g., ’10’).
- DIVISION: Division code (e.g., ’00’).
- Partner Data (
INQUIRY_PARTNERS
):
- PARTN_ROLE: Partner role (e.g., ‘SP’ for sold-to party).
- PARTN_NUMB: Customer number.
- Item Data (
INQUIRY_ITEMS_IN
):
- MATERIAL: Material number.
Example Parameter Definitions in ABAP:
DATA:
ls_inquiry_header_data TYPE BAPISDHD1,
lt_inquiry_item_data TYPE TABLE OF BAPISDITM,
lt_partner_data TYPE TABLE OF BAPIPARNR.
ls_inquiry_header_data-doc_type = 'IN'.
ls_inquiry_header_data-sales_org = '1000'.
ls_inquiry_header_data-distr_chan = '10'.
ls_inquiry_header_data-division = '00'.
" Define Partner Data
APPEND VALUE #( partn_role = 'SP' partn_numb = 'CUST001' ) TO lt_partner_data.
" Define Item Data
APPEND VALUE #( material = 'MAT123' ) TO lt_inquiry_item_data.
Step 4: Implement the BAPI in ABAP
With the parameters defined, the next step is to implement the BAPI within an ABAP program.
Procedure:
- Open ABAP Editor:
- Use transaction code
SE38
orSE80
to open the ABAP editor.
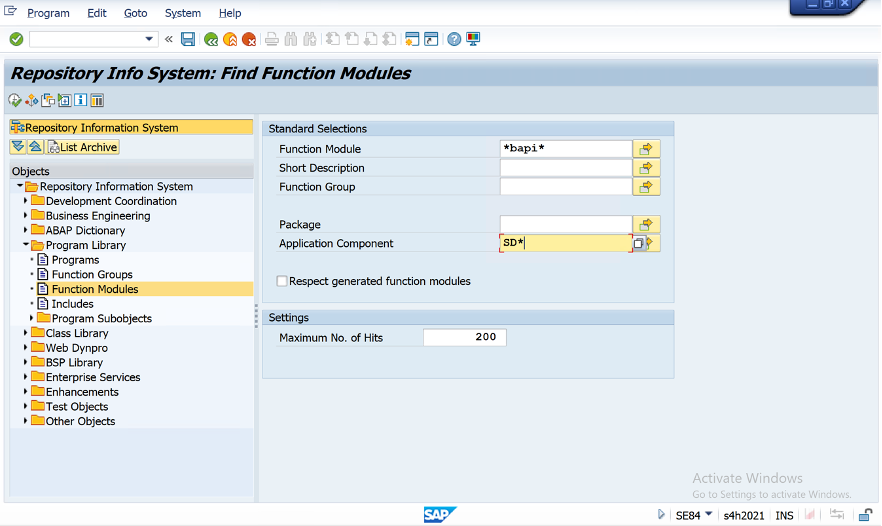
- Create a New Program or Use an Existing One:
- Create a new program or choose an existing one where the BAPI will be implemented.
- Call the BAPI:
- Use the
CALL FUNCTION
statement to execute the BAPI with the defined parameters.
Example ABAP Code:
CALL FUNCTION 'BAPI_INQUIRY_CREATEFROMDATA2'
EXPORTING
inquiry_header_in = ls_inquiry_header_data
TABLES
inquiry_items_in = lt_inquiry_item_data
inquiry_partners = lt_partner_data
return = lt_return.
" Check for errors
LOOP AT lt_return INTO ls_return.
IF ls_return-type = 'E'.
WRITE: / 'Error:', ls_return-message.
EXIT.
ENDIF.
ENDLOOP.
Step 5: Handle Errors and Exceptions
Proper error handling ensures that any issues during the BAPI execution are appropriately managed.
Procedure:
- Examine the RETURN Parameter:
- After calling the BAPI, the
RETURN
table contains messages indicating the success or failure of the operation.
- Implement Error Checking:
- Loop through the
RETURN
table to identify any error messages. - Handle errors as necessary, such as logging them or displaying user-friendly messages.
Example Error Handling:
IF sy-subrc = 0.
LOOP AT lt_return INTO ls_return.
IF ls_return-type = 'E'.
WRITE: / 'Error creating inquiry:', ls_return-message.
EXIT.
ENDIF.
ENDLOOP.
ELSE.
WRITE: / 'Unexpected error occurred.'.
ENDIF.
Step 6: Commit the Transaction
BAPIs do not automatically commit transactions to the database. It is essential to explicitly commit the transaction to ensure that changes are saved.
Procedure:
- Call the Commit BAPI:
- Use
BAPI_TRANSACTION_COMMIT
to finalize the transaction.
Example Commit Statement:
CALL FUNCTION 'BAPI_TRANSACTION_COMMIT'
EXPORTING
wait = 'X'.
Conclusion
Creating a customer inquiry using BAPI in SAP involves a systematic approach that ensures data integrity and process efficiency. By following the steps outlined above—identifying the correct BAPI, understanding its documentation, defining parameters, implementing the BAPI in ABAP, handling errors, and committing the transaction—you can effectively automate the creation of customer inquiries within the SAP system.
Best Practices:
- Consistent Naming Conventions: Use clear and consistent naming for variables and structures to enhance code readability.
- Thorough Testing: Rigorously test the BAPI implementation in a development environment before deploying it to production.
- Documentation: Maintain comprehensive documentation of the BAPI usage and any custom logic implemented.
- Error Handling: Implement robust error handling to manage exceptions gracefully and provide meaningful feedback to users.
Leveraging BAPIs like BAPI_INQUIRY_CREATEFROMDATA2
not only streamlines the inquiry creation process but also ensures that integrations with external systems are seamless and maintainable.