Apex pageblocksectionItem component
Apex pageblocksectionItem component can include up to two child components where an <apex: pageblocksection> creates one column in one row. If there is no content included in apex pageblocksectionItem component, then the content spans both cells of the column. If two child components are specified , the content of the first is rendered in left, “label” cells of the column, while the content of the second is rendered in the right , “data’’ cell of the column.
Apex pageblocksectionItem component attributes
dataStyle | This attribute is used to style the content using CSS. |
dataTitle | This is used to display the pageblocksection column. |
dir | This is used to display the direction of the text (RTL or LTR). |
labelStyle | Its a string type, these style used to display the content of the left “label” cell of the page block section column and uses all JavaScript enabled tags |
renderd | It is a Boolean types, that specifies whether the component is rendered on the page or not. |
In this Salesforce tutorial, we are going to create Visualforce page for addition of two number, subtraction and multiplication using apex pageblocksection and Apex pageblockSectionItem.
Visualforce page
<apex:page controller="subtraction" sidebar="false" >
<apex:form >
<apex:pageBlock title="Calculator">
<apex:pageBlockSection columns="2" title="Mathematical Operations"
collapsible="false">
<apex:pageBlockSectionItem >
<apex:outputLabel >Enter X value </apex:outputLabel>
<apex:inputText value="{!xvalue}"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem >
<apex:outputLabel >Enter Y value </apex:outputLabel>
<apex:inputText value="{!yvalue}"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem >
<apex:outputLabel >Result </apex:outputLabel>
<apex:inputText value="{!result}"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem >
<apex:outputLabel > You have performed {!operation} of {!xvalue} and {!yvalue}.
</apex:outputLabel>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem >
<apex:commandButton value="Addition" action="{!add}"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem >
<apex:commandButton value="Subtraction" action="{!sub}"/>
</apex:pageBlockSectionItem>
<apex:pageBlockSectionItem >
<apex:commandButton value="Multiplication" action="{!mul}"/>
</apex:pageBlockSectionItem>
</apex:pageBlockSection>
</apex:pageBlock>
</apex:form>
</apex:page>
In above Visualforce page, We have used controller “Subtraction” to perform addition, subtraction and multiplication operation. Apex : CommandButton and Apex:commandLink components must be defined inside Apex:form component.
- Add pageblock and enter Title as “Calculator”.
- Now add pageblocksection, Title and specify the number of columns that to be displayed.
- Now in Apex pageblockSectionItem component, enter <apex:outputLabel> and <apex:inputText> as shown above.
Controller
public class subtraction
{
public Integer xvalue {get;set;}
public Integer yvalue {get;set;}
public Integer result {get;set;}
public string operation {get;set;}
public pagereference sub()
{
result = xvalue-yvalue;
operation = 'Subtraction';
return null;
}
public pagereference add()
{
result = xvalue+yvalue;
operation = 'Addition';
return null;
}
public pagereference mul()
{
result = xvalue*yvalue;
operation = 'Multiplication';
return null;
}
}
Output
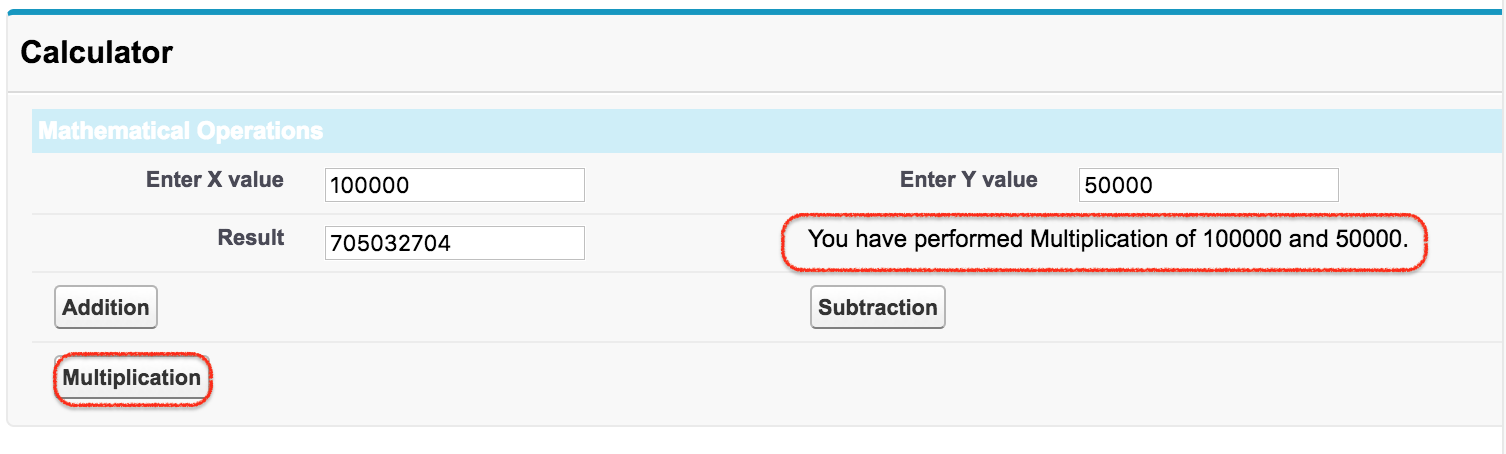
- Enter x value and Y value.
- Now click on the operation that you want to perform.
- The result will be displayed as shown above.