Custom controller is an Apex class which is used to implement all the logic of Visualforce without leveraging the standard functionality. In Salesforce.com, Standard controllers provides all the functionalities that are required for Visualforce pages. If we want to override the existing functionalities , then we can write our own Custom controller in Salesforce or a Controller extension using Apex class.
When Custom Controller in Salesforce is used?
- Custom Controller in Salesforce is used to override the built in functionalities of a standard controller.
- To add new actions.
- To build visualforce pages that respects user permissions.
- Custom controller and Controller extensions classes executes in system mode.
- User Permissions, field level security, Organization wide defaults, Role hierarchy and Sharing rules can be done using keyword called with sharing.
How to build Custom Controller in Salesforce?
Custom Controller in Salesforce is an Apex Class. To create Custom controller navigate to Setup | Build | develop | Apex Classes | New.
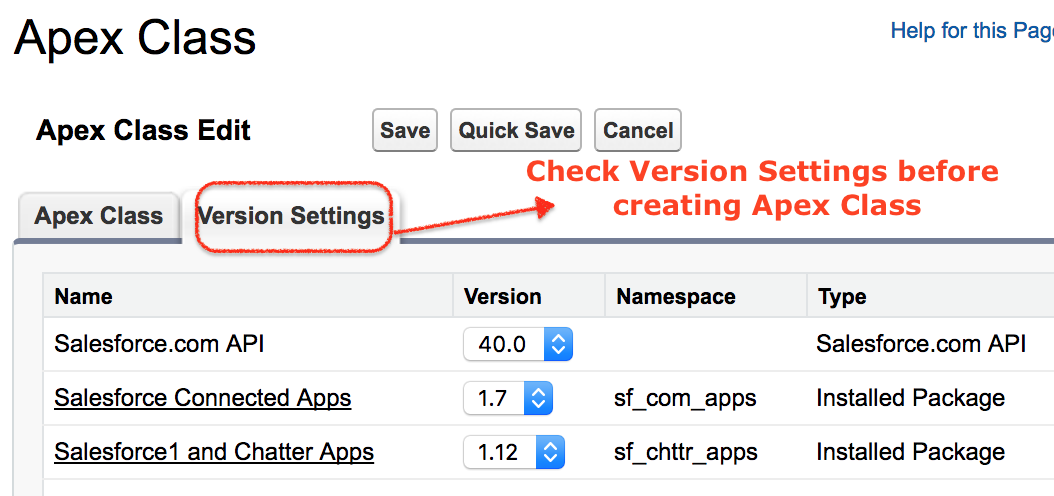
- Click on Version settings before creating Custom controller in Salesforce. This version settings specify version of Apex and the API used.
- Now click on Apex class editor to write Apex class for Custom controller in Salesforce.
- In general, In single apex class we can add upto 1 million characters in length.
public class CustomController {
private final Account account;
public CustomController() {
account = [SELECT Id, Name, Site FROM Account
WHERE Id = :ApexPages.currentPage().getParameters().get('id')];
}
public Account getAccount() {
return account;
}
public PageReference save() {
update account;
return null;
}
}
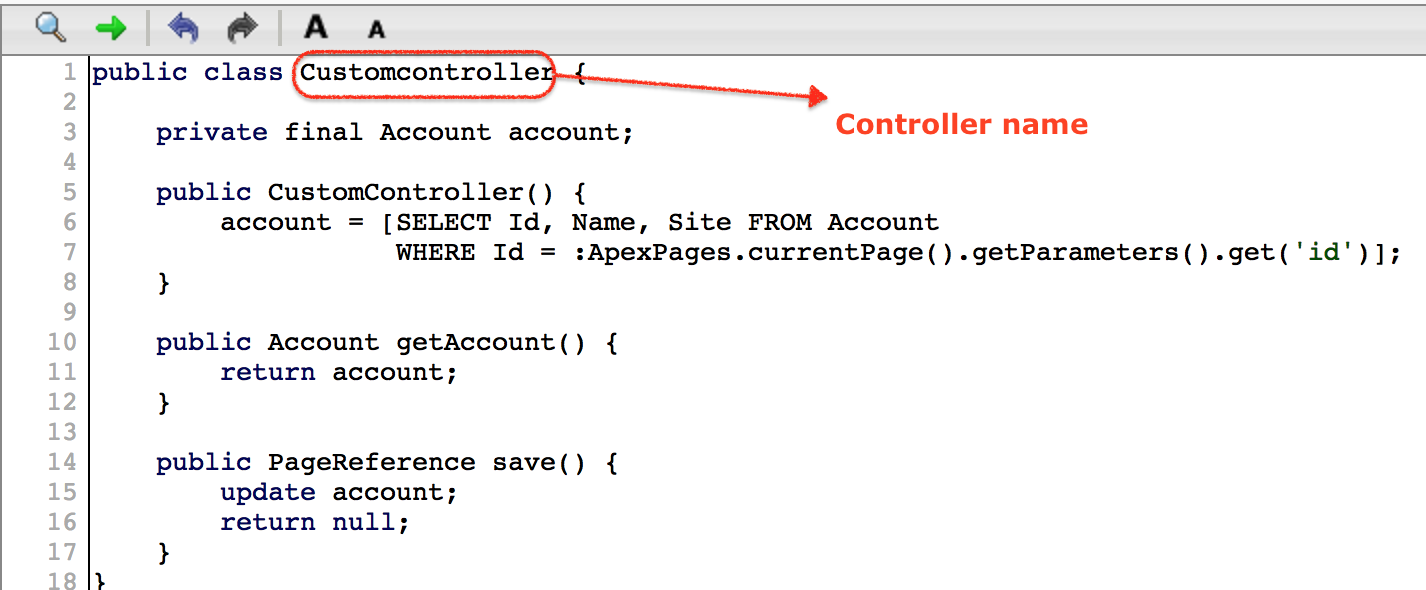
- Customcontroller is the name of the controller.
- Click on Save button.
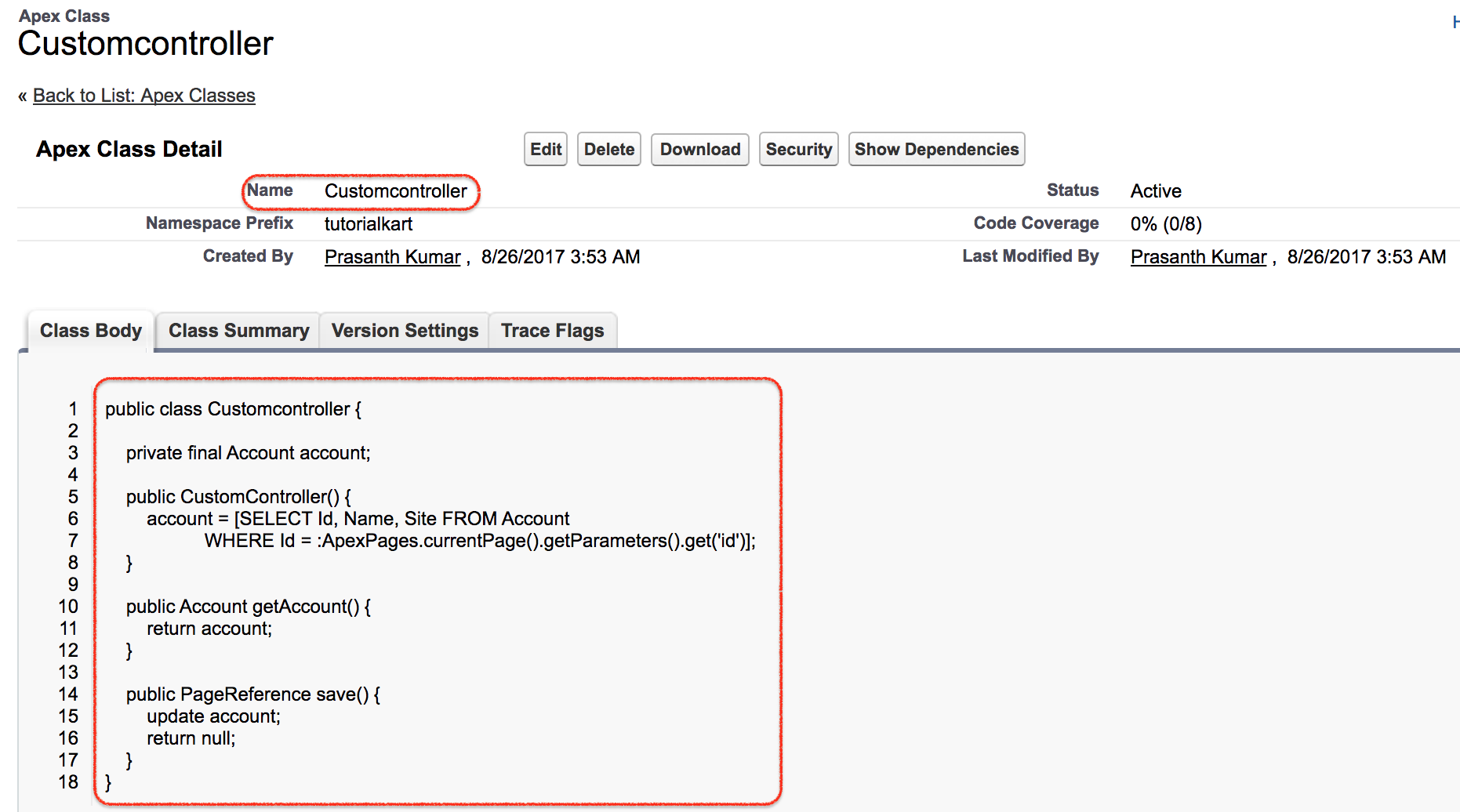
How to implement Custom controller in Visualforce pages.
To implement Custom Controller in Visualforce pages, first we have to create new visualforce page in Salesforce.Follow the steps given below to implement custom controller.
- Click on the link to create new Visualforce page in salesforce.
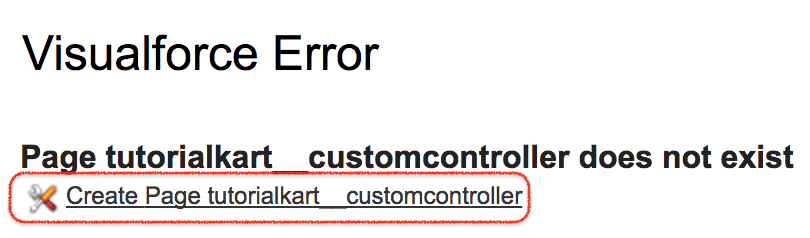
- In this visualforce page, we writing apex code to open Account in form. Account id must be added at the end of the URL
- https://tutorialkart-dev-ed–tutorialkart.ap4.visual.force.com/apex/customcontroller?id=0016F000026m5ZB
<apex:page controller="CustomController" tabStyle="Account">
<apex:form>
<apex:pageBlock title="Congratulations {!$User.FirstName}">
You belong to Account Name: <apex:inputField value="{!account.name}"/>
<apex:commandButton action="{!save}" value="save"/>
</apex:pageBlock>
</apex:form>
</apex:page>
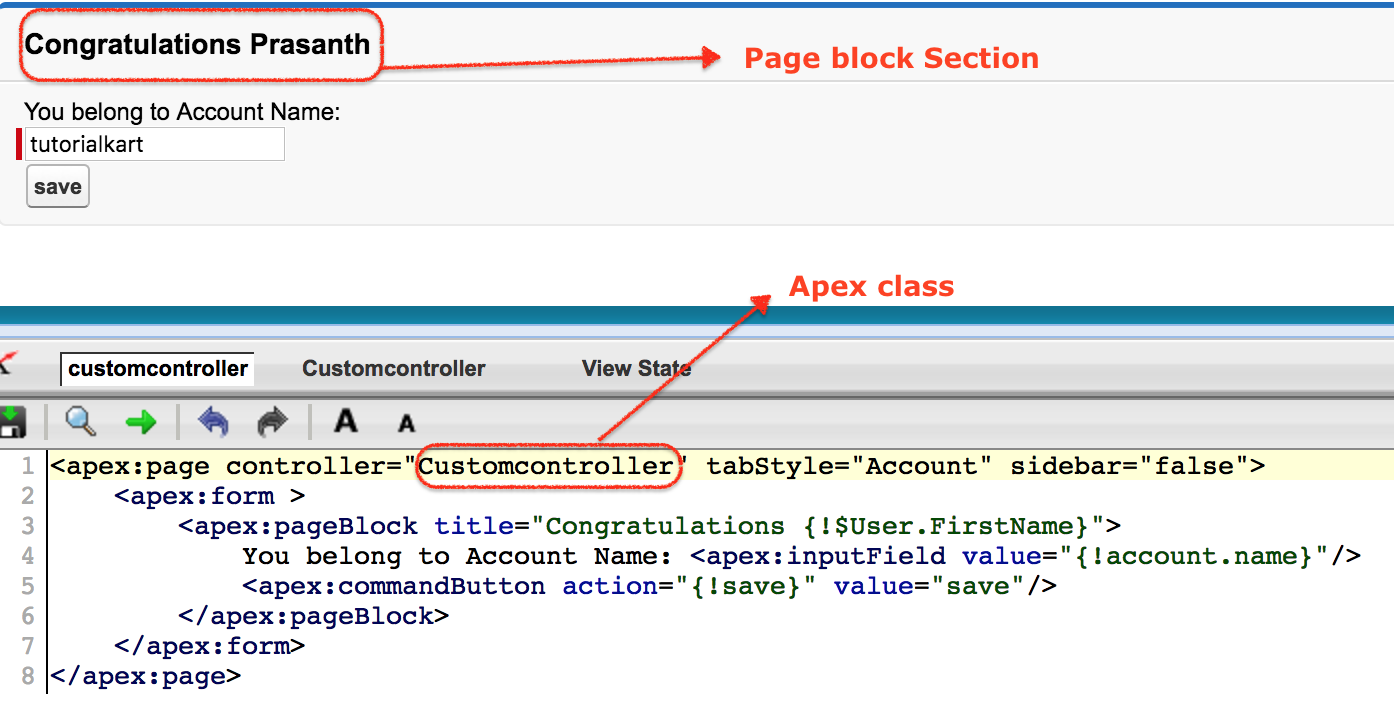
The Custom controller is associated with the page because of the Controller attribute of the <apex:page> component. The custom controller method in Salesforce must be referenced with {!}.
- {!User.Firstname} – This method retrieves the firstname of the User.
In above apex class, getAccount method is referenced by <apex:inputfield> tag in visualforce page and <apex:commandButton> tag references the save method with its action attribute.
- Finally click on Save.
In output section, If we rename the account name and Saved. The account name will be renamed with the new name.