In this C++ tutorial, you will learn how to check if a given string ends with a specific suffix value, with example programs.
Check if the string ends with a specific suffix in C++
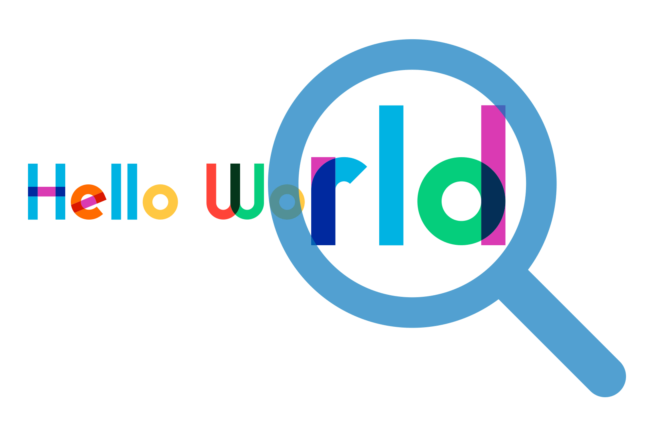
To check if the string starts with a specific suffix value in C++, get the substring of the given string that starts at index = string length – suffix length. Compare this substring to the specified prefix value.
If the substring equals the prefix value, then we can say that the given string ends with the specified suffix value. Otherwise the given string does not end with the specified value.
1. Checking if the string ends with a specific suffix using substring and string equality in C++
In the following program, we take a string in str
and a suffix string value in suffix
. We have to check if the string str
ends with the suffix string suffix
using string substr() method and string equality check.
Steps
- Given an input string in
str
, and a suffix string insuffix
. - Call the
substr()
method of the string class to extract a substring ofstr
starting from index = string length – suffix length. Store the extracted substring in variablesubstring
. - Then compare the substring with the
suffix
string using the==
operator. - If the substring equals the suffix value, then the string
str
ends with the specified suffix value. Else the stringstr
does not end with the specified suffix value. You can use C++ If Else statement to implement this step, withsubstring == prefix
as the if-condition.
Program
main.cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World";
string suffix = "rld";
string substring = str.substr(str.length() - suffix.length());
if (substring == suffix) {
cout << "The string ends with the suffix." << endl;
} else {
cout << "The string does not end with the suffix." << endl;
}
return 0;
}
Since the given string in str
ends with the specified suffix string, the condition substring == suffix
returns true, and the if-block runs.
Output
The string ends with the suffix.
Now, let us change the suffix value, and run the program again.
main.cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World";
string suffix = "Apple";
string substring = str.substr(str.length() - suffix.length());
if (substring == suffix) {
cout << "The string ends with the suffix." << endl;
} else {
cout << "The string does not end with the suffix." << endl;
}
return 0;
}
Since the given string in str
does not end with the specified suffix string, the condition substring == suffix
returns false, and the else-block runs.
Output
The string does not end with the suffix.
Conclusion
In this C++ Tutorial, we learned how to check if the string ends with the specified suffix value using string substr() method and string equality check, with examples.