R Tutorial – We shall learn about R Operators – Arithmetic, Relational, Logical, Assignment and some of the Miscellaneous Operators that R programming language provides.
R Operators
There are four main categories of Operators in R programming language. They are shown in the following picture :
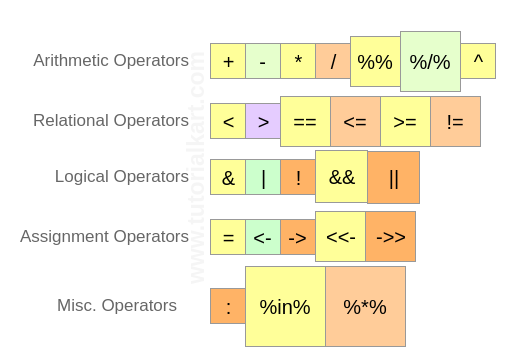
We shall learn about these operators in detail with Example R programs.
R Arithmetic Operators

Arithmetic Operators are used to accomplish arithmetic operations. They can be operated on the basic data types Numericals, Integers, Complex Numbers. Vectors with these basic data types can also participate in arithmetic operations, during which the operation is performed on one to one element basis.
Operator | Description | Usage |
+ | Addition of two operands | a + b |
– | Subtraction of second operand from first | a – b |
* | Multiplication of two operands | a * b |
/ | Division of first operand with second | a / b |
%% | Remainder from division of first operand with second | a %% b |
%/% | Quotient from division of first operand with second | a %/% b |
^ | First operand raised to the power of second operand | a^b |
An example for each of the arithmetic operator on Numerical values is provided below.
r_op_arithmetic.R
# R Arithmetic Operators Example for integers a <- 7.5 b <- 2 print ( a+b ) #addition print ( a-b ) #subtraction print ( a*b ) #multiplication print ( a/b ) #Division print ( a%%b ) #Reminder print ( a%/%b ) #Quotient print ( a^b ) #Power of
Output
$ Rscript r_op_arithmetic.R [1] 9.5 [1] 5.5 [1] 15 [1] 3.75 [1] 1.5 [1] 3 [1] 56.25
An example for each of the arithmetic operator on Vectors is provided below.
r_op_arithmetic_vector.R
# R Operators - R Arithmetic Operators Example for vectors a <- c(8, 9, 6) b <- c(2, 4, 5) print ( a+b ) #addition print ( a-b ) #subtraction print ( a*b ) #multiplication print ( a/b ) #Division print ( a%%b ) #Reminder print ( a%/%b ) #Quotient print ( a^b ) #Power of
Output
$ Rscript r_op_arithmetic_vector.R [1] 10 13 11 [1] 6 5 1 [1] 16 36 30 [1] 4.00 2.25 1.20 [1] 0 1 1 [1] 4 2 1 [1] 64 6561 7776
R Relational Operators

Relational Operators are those that find out relation between the two operands provided to them. Following are the six relational operations R programming language supports.The output is boolean (TRUE or FALSE) for all of the Relational Operators in R programming language.
Operator | Description | Usage |
< | Is first operand less than second operand | a < b |
> | Is first operand greater than second operand | a > b |
== | Is first operand equal to second operand | a == b |
<= | Is first operand less than or equal to second operand | a <= b |
>= | Is first operand greater than or equal to second operand | a > = b |
!= | Is first operand not equal to second operand | a!=b |
An example for each of the relational operator on Numberical values is provided below.
r_op_relational.R
# R Operators - R Relational Operators Example for Numbers a <- 7.5 b <- 2 print ( ab ) # greater than print ( a==b ) # equal to print ( a<=b ) # less than or equal to print ( a>=b ) # greater than or equal to print ( a!=b ) # not equal to
Output
$ Rscript r_op_relational.R [1] FALSE [1] TRUE [1] FALSE [1] FALSE [1] TRUE [1] TRUE
An example for each of the relational operator on Vectors is provided below.
r_op_relational_vector.R
# R Operators - R Relational Operators Example for Numbers a <- c(7.5, 3, 5) b <- c(2, 7, 0) print ( a<b ) # less than print ( a>b ) # greater than print ( a==b ) # equal to print ( a<=b ) # less than or equal to print ( a>=b ) # greater than or equal to print ( a!=b ) # not equal to
Output
$ Rscript r_op_relational_vector.R [1] FALSE TRUE FALSE [1] TRUE FALSE TRUE [1] FALSE FALSE FALSE [1] FALSE TRUE FALSE [1] TRUE FALSE TRUE [1] TRUE TRUE TRUE
R Logical Operators

Logical Operators in R programming language work only for the basic data types logical, numeric and complex and vectors of these basic data types.
Operator | Description | Usage |
& | Element wise logical AND operation. | a & b |
| | Element wise logical OR operation. | a | b |
! | Element wise logical NOT operation. | !a |
&& | Operand wise logical AND operation. | a && b |
|| | Operand wise logical OR operation. | a || b |
An example for each of the logical operators on Numerical values is provided below.
r_op_logical.R
# R Operators - R Logical Operators Example for basic logical elements a <- 0 # logical FALSE b <- 2 # logical TRUE print ( a & b ) # logical AND element wise print ( a | b ) # logical OR element wise print ( !a ) # logical NOT element wise print ( a && b ) # logical AND consolidated for all elements print ( a || b ) # logical OR consolidated for all elements
Output
$ Rscript r_op_logical.R [1] FALSE [1] TRUE [1] TRUE [1] FALSE [1] TRUE
An example for each of the logical operators on Vectors is provided below.
r_op_logical_vector.R
# R Operators - R Logical Operators Example for boolean vectors a <- c(TRUE, TRUE, FALSE, FALSE) b <- c(TRUE, FALSE, TRUE, FALSE) print ( a & b ) # logical AND element wise print ( a | b ) # logical OR element wise print ( !a ) # logical NOT element wise print ( a && b ) # logical AND consolidated for all elements print ( a || b ) # logical OR consolidated for all elements
Output
$ Rscript r_op_logical_vector.R [1] TRUE FALSE FALSE FALSE [1] TRUE TRUE TRUE FALSE [1] FALSE FALSE TRUE TRUE [1] TRUE [1] TRUE
R Assignment Operators

Assignment Operators are those that help in assigning a value to the variable.
Operator | Description | Usage |
= | Assigns right side value to left side operand | a = 3 |
<- | Assigns right side value to left side operand | a <- 5 |
-> | Assigns left side value to right side operand | 4 -> a |
<<- | Assigns right side value to left side operand | a <<- 3.4 |
->> | Assigns left side value to right side operand | c(1,2) ->> a |
An example for each of the assignment operators is provided below.
r_op_assignment.R
# R Operators - R Assignment Operators a = 2 print ( a ) a <- TRUE print ( a ) 454 -> a print ( a ) a <<- 2.9 print ( a ) c(6, 8, 9) -> a print ( a )
Output
$ Rscript r_op_assignment.R [1] 2 [1] TRUE [1] 454 [1] 2.9 [1] 6 8 9
R Miscellaneous Operators
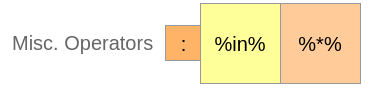
These operators does not fall into any of the categories mentioned above, but are significantly important during R programming for manipulating data.
Operator | Description | Usage |
: | Creates series of numbers from left operand to right operand | a:b |
%in% | Identifies if an element(a) belongs to a vector(b) | a %in% b |
%*% | Performs multiplication of a vector with its transpose | A %*% t(A) |
An example for each of the Miscellaneous operators is provided below.
r_op_misc.R
# R Operators - R Misc Operators a = 23:31 print ( a ) a = c(25, 27, 76) b = 27 print ( b %in% a ) M = matrix(c(1,2,3,4), 2, 2, TRUE) print ( M %*% t(M) )
Output
$ Rscript r_op_misc.R [1] 23 24 25 26 27 28 29 30 31 [1] TRUE [,1] [,2] [1,] 5 11 [2,] 11 25
Conclusion
In this R Tutorial, we have learnt about R Operators – R Arithmetic Operators, R Relational Operators, R Logical Operators, R Assignment Operators, R Miscellaneous Operators with example R commands and R script files.