Click a Link based on Partial Link Text
To click a link (anchor element) based on partial link text using Selenium in Java, find the element (web element) by the partial link text By.partialLinkText()
and then call click()
function on this web element.
The following is a simple code snippet where we find the link with the partial text "mail"
and then click on this link using Selenium.
</>
Copy
WebElement mailLink = driver.findElement(By.partialLinkText("mail"));
mailLink.click();
Example
In the following, we write Selenium Java program to visit google.com, and click the link which has the partial link text "mail"
. In google.com web page, we have "Gmail"
link with the partial text "mail"
.
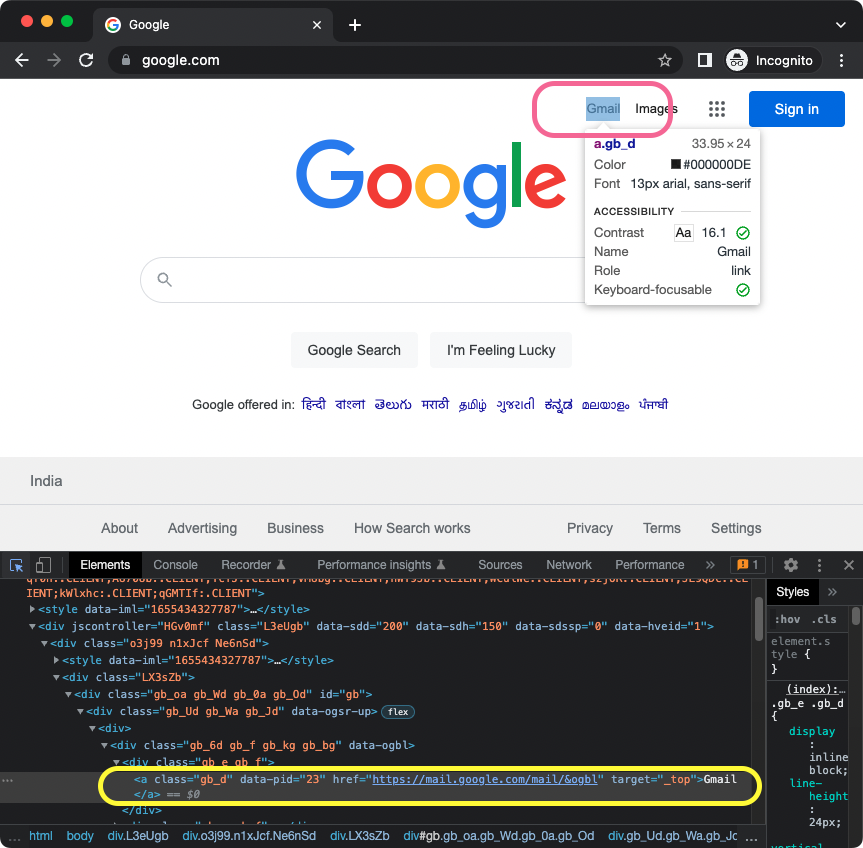
Java Program
</>
Copy
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://google.com/ncr");
WebElement mailLink = driver.findElement(By.partialLinkText("mail"));
mailLink.click();
driver.quit();
}
}
Screenshots
1. Initialize web driver and visit google.com.
</>
Copy
WebDriver driver = new ChromeDriver();
driver.get("https://google.com/ncr");
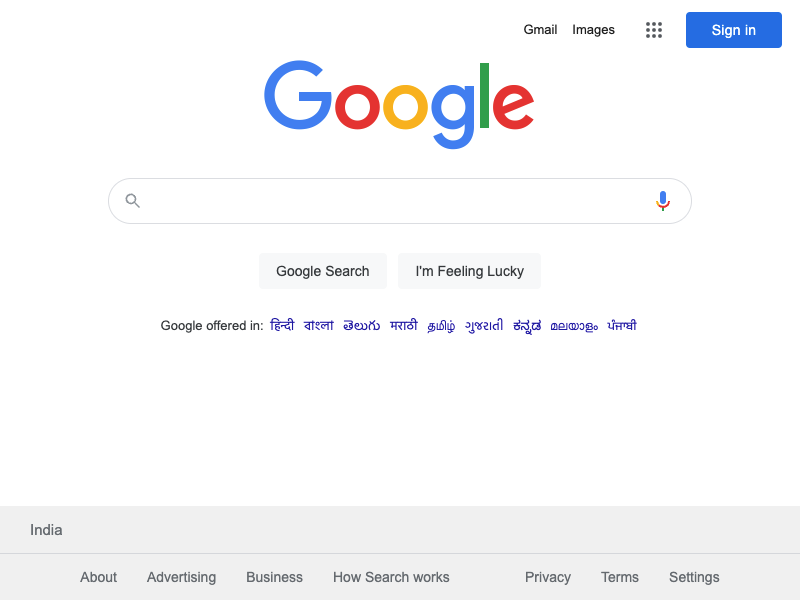
2. Find Web Element using the partial link text "mail"
, and click on the element.
</>
Copy
WebElement mailLink = driver.findElement(By.partialLinkText("mail"));
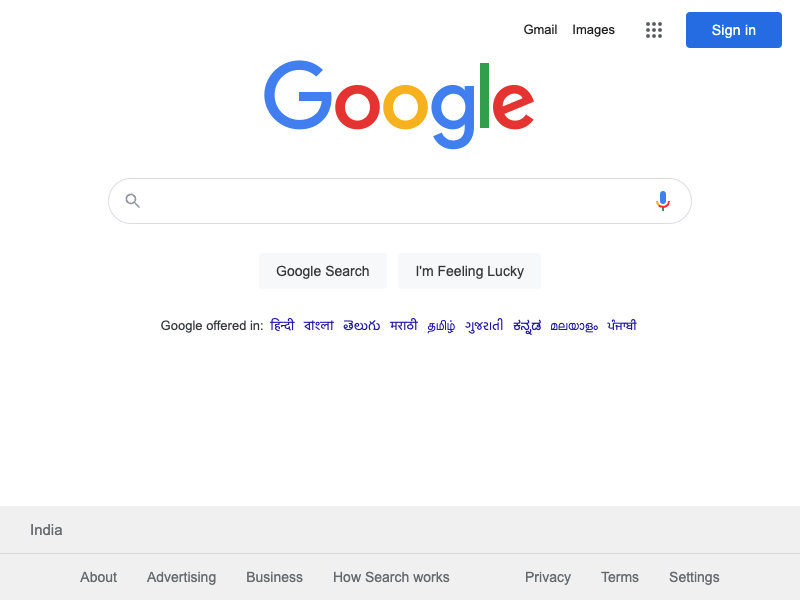
</>
Copy
mailLink.click();
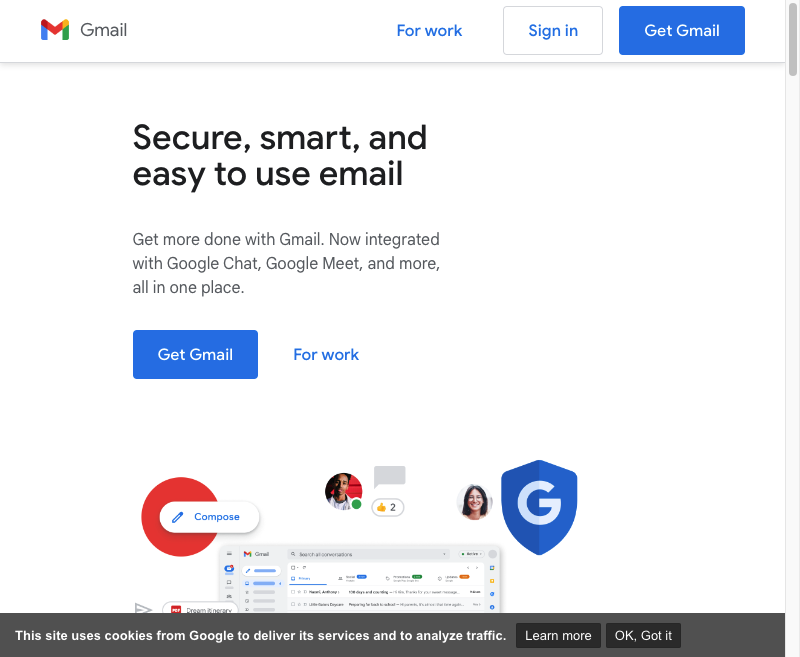