Find Link Element by href
To find a link (anchor element) based on the value in href attribute using Selenium in Java, find the element by xpath By.xpath()
and specify the XPath expression for anchor tag element with href attribute set to the required URL.
The following is a simple code snippet where we find the link element with href="https://www.tutorialkart.com/java/java-for-loop/"
.
String url = "https://www.tutorialkart.com/java/java-for-loop/"; WebElement link = driver.findElement(By.xpath("//a[@href=\"" + url + "\"]"));
Example
ADVERTISEMENT
In the following, we write Selenium Java program to visit https://www.tutorialkart.com/java/
, and find the link with URL https://www.tutorialkart.com/java/java-for-loop/
.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/java/"); String url = "https://www.tutorialkart.com/java/java-for-loop/"; WebElement link = driver.findElement(By.xpath("//a[@href=\"" + url + "\"]")); System.out.println(link.getText()); driver.quit(); } }
Screenshots
1. Initialize web driver and visit https://www.tutorialkart.com/java/
.
WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/java/");
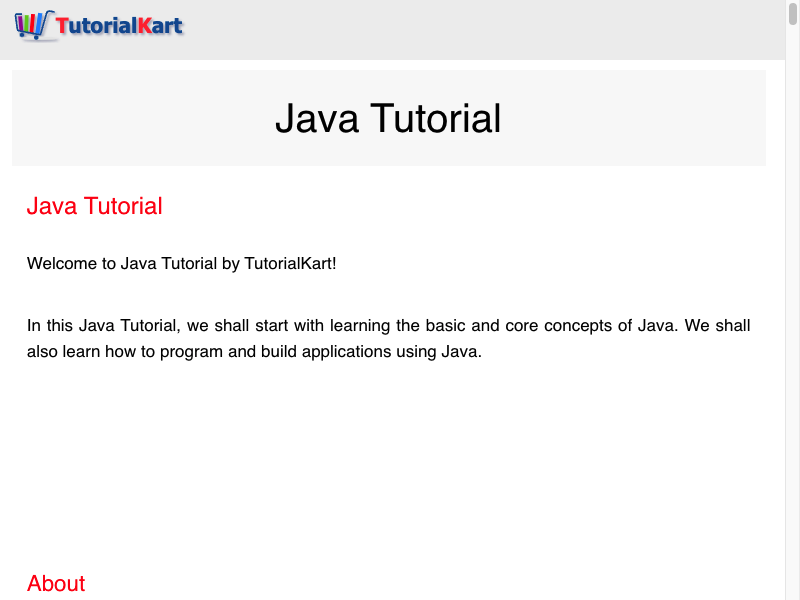
2. Find web element with href="https://www.tutorialkart.com/java/java-for-loop/"
.
WebElement link = driver.findElement(By.xpath("//a[@href=\"" + url + "\"]")); System.out.println(link.getText());
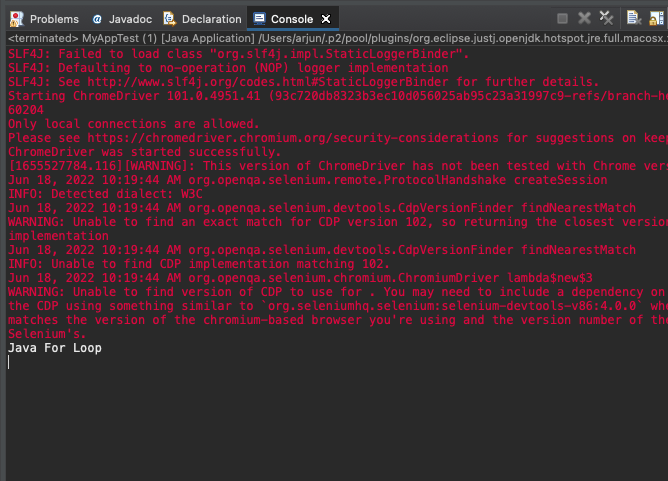