Click a Button
To click a button using Selenium in Java, find the button (web element) and then call click()
function on this button web element. The button can be found by name, id, class name, etc.
The following is a simple code snippet where we find an element with the name "btnK"
and then click on this button using Selenium.
WebElement searchButton = driver.findElement(By.name("btnK")); searchButton.click();
Example
ADVERTISEMENT
In the following program, we write Selenium Java code to visit google.com, enter a keyword in the search box, and then click on the Google Search
button. The focus would be on how to click a button using Selenium.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr"); WebElement searchBox = driver.findElement(By.name("q")); searchBox.sendKeys("Selenium"); WebElement searchButton = driver.findElement(By.name("btnK")); searchButton.click(); driver.quit(); } }
Screenshots
1. Initialize web driver and visit google.com.
WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr");
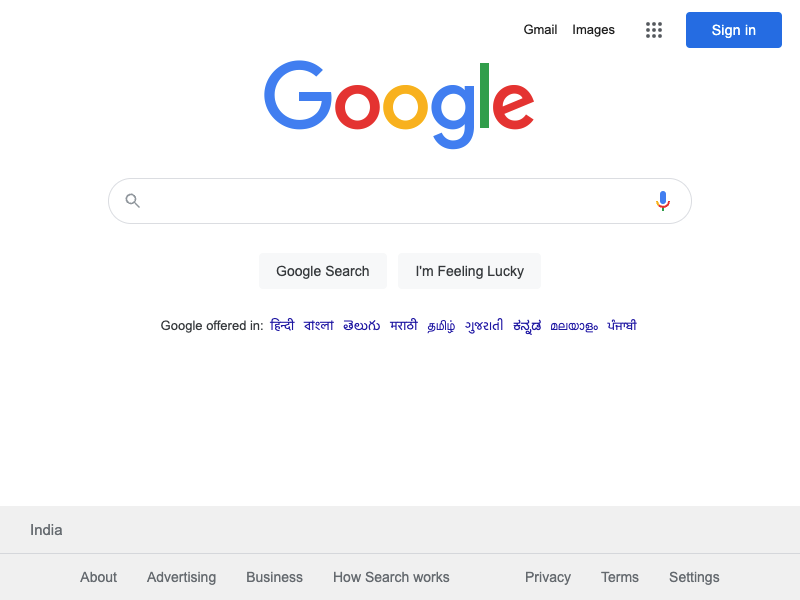
2. Enter string "Selenium"
in search box.
WebElement searchBox = driver.findElement(By.name("q")); searchBox.sendKeys("Selenium");
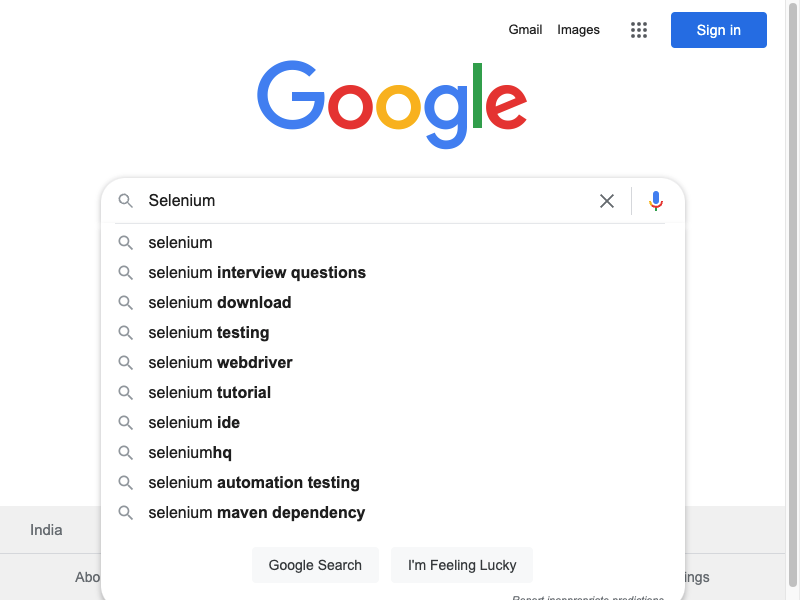
3. Click on the ‘Google Search’ button which is found by the name “btnK”.
WebElement searchButton = driver.findElement(By.name("btnK")); searchButton.click();
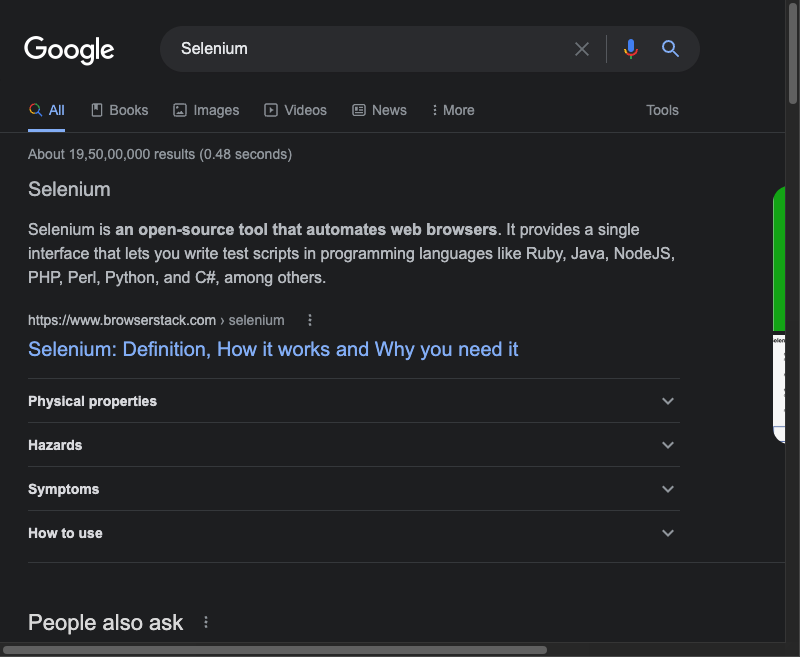