Get Browser Window Size
To get browser window size using Selenium in Java, we can use driver.manage().window().getSize()
where driver is the WebDriver object.
Now, let us breakdown the code snippet driver.manage().window().getSize()
.
driver.manage()
returns an Options Interface.driver.manage().window()
returns an Options Interface for the Browser Window.driver.manage().window().getSize()
returns a Dimension object repressing the dimensions of the current window.
Example
ADVERTISEMENT
In the following program, we write Selenium Java code to visit Google Page, and get the browser’s window dimensions (width, height). We can access the width and height values from the Dimension object using getWidth()
and getHeight()
methods respectively.
Java Program
import org.openqa.selenium.Dimension; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.google.com/ncr"); Dimension size = driver.manage().window().getSize(); System.out.println("Window Size / Dimensions : " + size); System.out.println("Width : " + size.getWidth()); System.out.println("Height : " + size.getHeight()); driver.quit(); } }
Output
Window Size / Dimensions : (1200, 1371) Width : 1200 Height : 1371
Screenshot
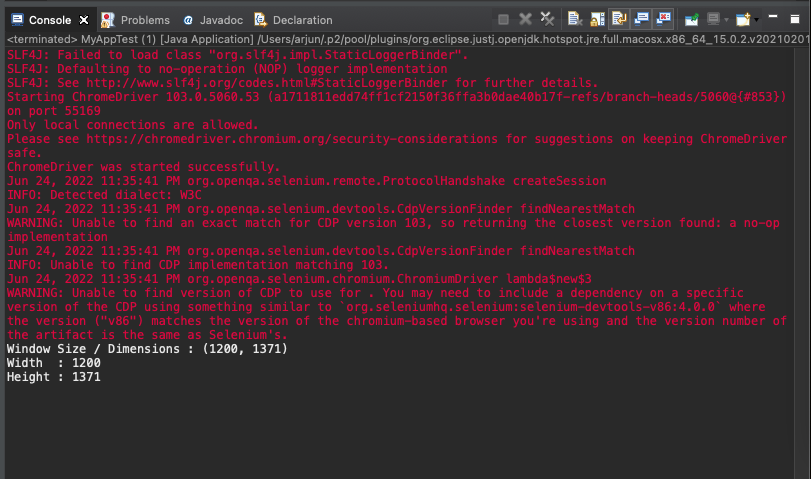