Get Title of Current Web Page
To get the title of the current web page programmatically using Selenium in Java, call WebDriver.getTitle()
function.
WebDriver.getTitle()
method returns a string representing the title of the current web page opened in the browser by Selenium.
The following is a simple code snippet to initialize a web driver and get the title of the current web page.
WebDriver driver = new ChromeDriver(); //some code String title = driver.getTitle();
ADVERTISEMENT
Example
In the following program, we write Java code to initialize a web driver, visit tutorialkart.com, and get the title of that web page.
Java Program
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/"); String title = driver.getTitle(); System.out.println(title); driver.quit(); } }
Output
Tutorial Kart - Best Online Learning Site for Free Tutorials, Online Training, Courses, Materials and Interview Questions - SAP Tutorial, Salesforce Tutorial, Informatica Tutorial, Kotlin Tutorial, Kotlin Android Tutorial, Spark Tutorial, Kafka Tutorial, Node JS Tutorial
Screenshots
1. Initialize web driver and navigate to tutorialkart.com.
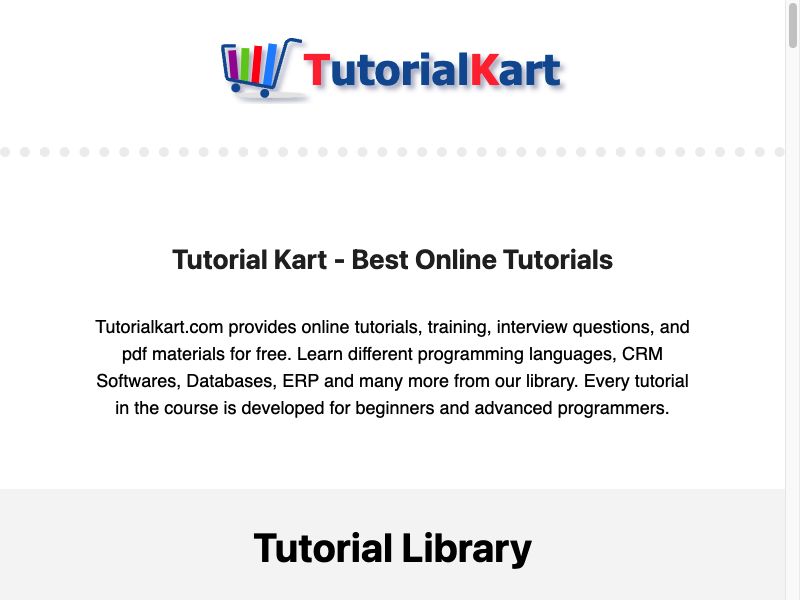
2. Print title of the page to console.
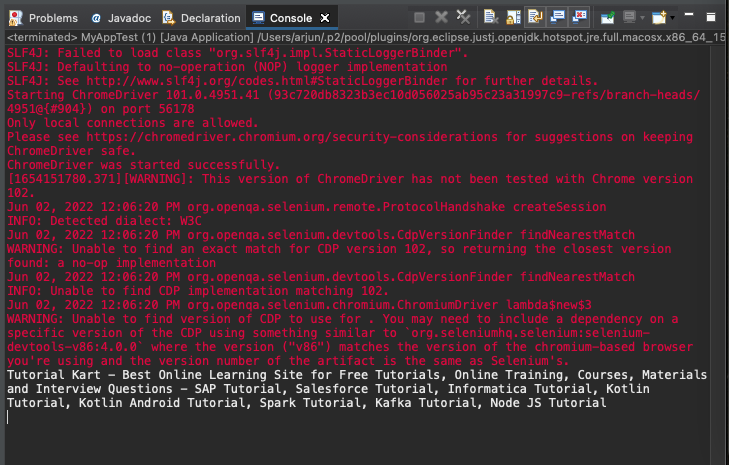