Get All Cookies
To get all the cookies for current browsing context using Selenium for Java, call manage().getCookies()
on the WebDriver object.
The following is a simple code snippet to get all the cookies
driver.manage().getCookies()
This expression returns a Set of Cookie objects.
Note: The server may set cookies during the page load. So, make sure that you fetch the cookies after the page load is complete. We shall cover an example to wait for a specific time to load the page, to get all the cookies.
Example
In the following program, we write Selenium Java code to visit Wikipedia Home Page, and get all the Cookies.
Java Program
import java.util.Set; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://en.wikipedia.org/wiki/Main_Page"); //get all cookies Set<Cookie> cookies = driver.manage().getCookies(); System.out.println(cookies); } finally { driver.quit(); } } }
Output
[GeoIP=IN:TG:Hyderabad:19.99:99.99:v4; path=/; domain=.wikipedia.org;secure;, WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 06:49:47 IST; path=/; domain=.wikipedia.org;secure;, WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 06:49:47 IST; path=/; domain=en.wikipedia.org;secure;]
Screenshot
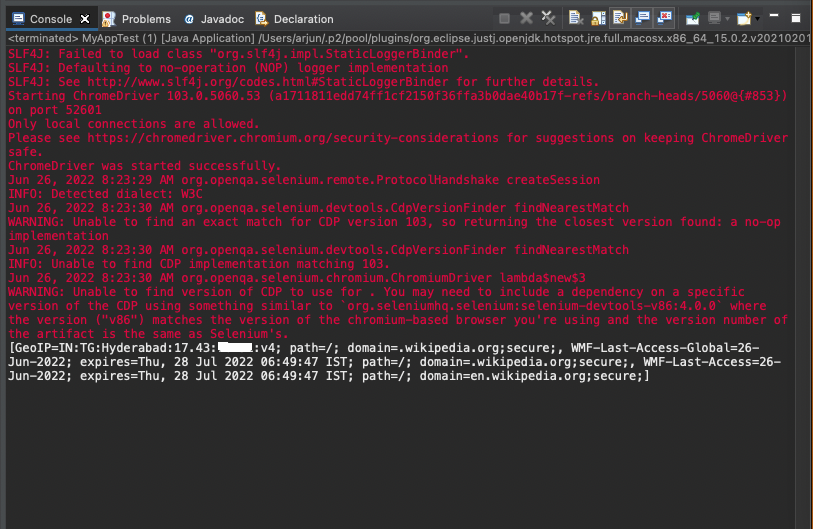
We can iterate over the Set of Cookie objects using a Java For Loop, as shown in the following.
Java Program
import java.util.Set; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://en.wikipedia.org/wiki/Main_Page"); Set<Cookie> cookies = driver.manage().getCookies(); for(Cookie cookie: cookies) { System.out.println(cookie + "\n"); } } finally { driver.quit(); } } }
Output
GeoIP=IN:TG:Hyderabad:17.43:78.51:v4; path=/; domain=.wikipedia.org;secure; WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 07:37:35 IST; path=/; domain=.wikipedia.org;secure; WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 07:37:35 IST; path=/; domain=en.wikipedia.org;secure;
Wait and Get All the Cookies
Now, we shall wait for five seconds before getting the cookies.
Java Program
import java.util.Set; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); try { driver.get("https://en.wikipedia.org/wiki/Main_Page"); wait(driver); Set<Cookie> cookies = driver.manage().getCookies(); for(Cookie cookie: cookies) { System.out.println(cookie + "\n"); } } finally { driver.quit(); } } public static void wait(WebDriver driver) { synchronized (driver) { try { driver.wait(5000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
Output
enwikimwuser-sessionId=9079965798bfe1cae009; path=/; domain=en.wikipedia.org GeoIP=IN:TG:Hyderabad:17.43:78.51:v4; path=/; domain=.wikipedia.org;secure; enwikiwmE-sessionTickLastTickTime=1656217083172; expires=Tue, 26 Jul 2022 09:48:03 IST; path=/; domain=en.wikipedia.org WMF-Last-Access-Global=26-Jun-2022; expires=Thu, 28 Jul 2022 08:14:18 IST; path=/; domain=.wikipedia.org;secure; enwikiwmE-sessionTickTickCount=1; expires=Tue, 26 Jul 2022 09:48:03 IST; path=/; domain=en.wikipedia.org WMF-Last-Access=26-Jun-2022; expires=Thu, 28 Jul 2022 08:14:18 IST; path=/; domain=en.wikipedia.org;secure;
Now, there are total six cookies, whereas in the previous program without waiting, there are only three cookies.