Get Next Sibling Web Element
To get the immediate next sibling element of given web element using Selenium for Java, find element on the given web element by XPath with the XPath expression as "following-sibling::*"
.
The following is a simple code snippet to get the next sibling element of the web element element
.
element.findElement(By.xpath("following-sibling::*"))
This expression returns the sibling WebElement following the element
.
ADVERTISEMENT
Example
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, and find the next sibling element of the link element <a href="/java/">Java</a>
. We shall print the outer HTML attribute of both the element and sibling element to standard output.
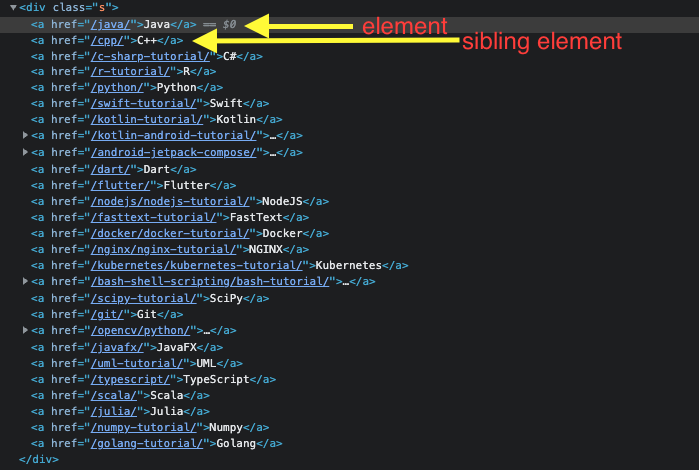
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialkart.com/"); //child element WebElement element = driver.findElement(By.xpath("//a[@href=\"/java/\"]")); System.out.println("Child\n" + element.getAttribute("outerHTML")); //get sibling element WebElement sibling = element.findElement(By.xpath("following-sibling::*")); System.out.println("\n\nSibling\n" + sibling.getAttribute("outerHTML")); driver.quit(); } }
Output
Child <a href="/java/">Java</a> Sibling <a href="/cpp/">C++</a>
Screenshot
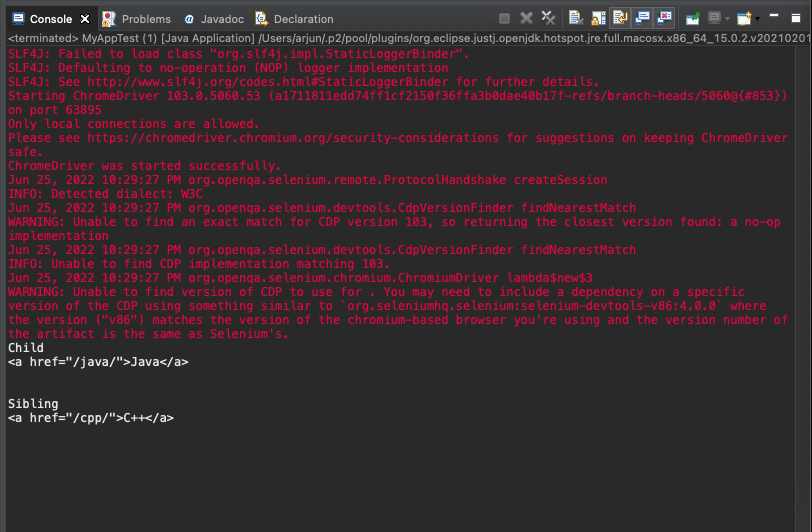