Get All Links in Web Page
To get all the links (of anchor elements) in a web page using Selenium in Java, find the web elements with the tag name "a"
using driver.findElements(By.tagName("a"))
. findElements() returns a List of web elements.
The following is a simple code snippet where we get all the link elements using Selenium.
List<WebElement> links = driver.findElements(By.tagName("a"));
And then, in a loop, we can access the actual link by getting the "href"
attribute value using getAttribute("href")
.
ADVERTISEMENT
Example
In the following, we write Selenium Java program to visit google.com, and extract all the links in this web page.
Java Program
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr"); List<WebElement> links = driver.findElements(By.tagName("a")); for(WebElement link: links) { System.out.println(link.getAttribute("href")); } driver.quit(); } }
Screenshots
1. Initialize web driver and visit google.com.
WebDriver driver = new ChromeDriver(); driver.get("https://google.com/ncr");
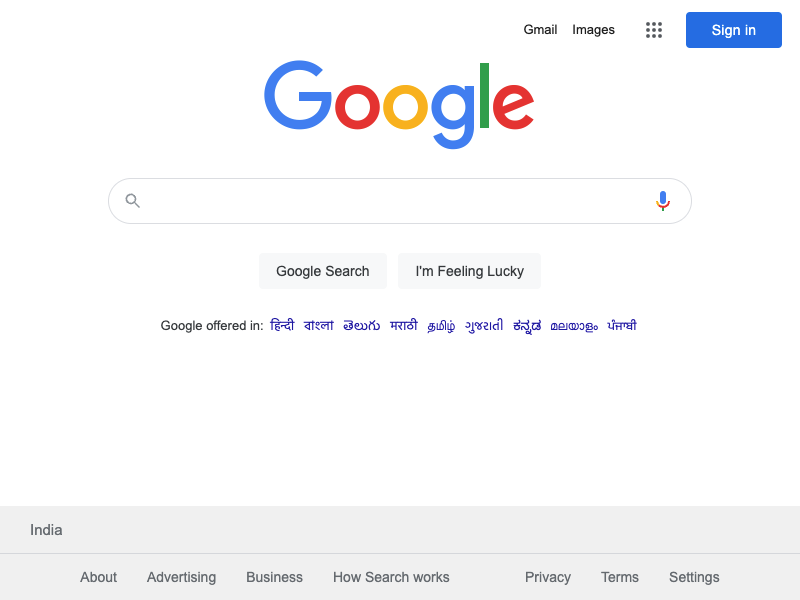
2. Find all Web Elements with the tag name "a"
.
List<WebElement> links = driver.findElements(By.tagName("a"));
Print the link’s href attribute to console, for all the link elements.
for(WebElement link: links) { System.out.println(link.getAttribute("href")); }
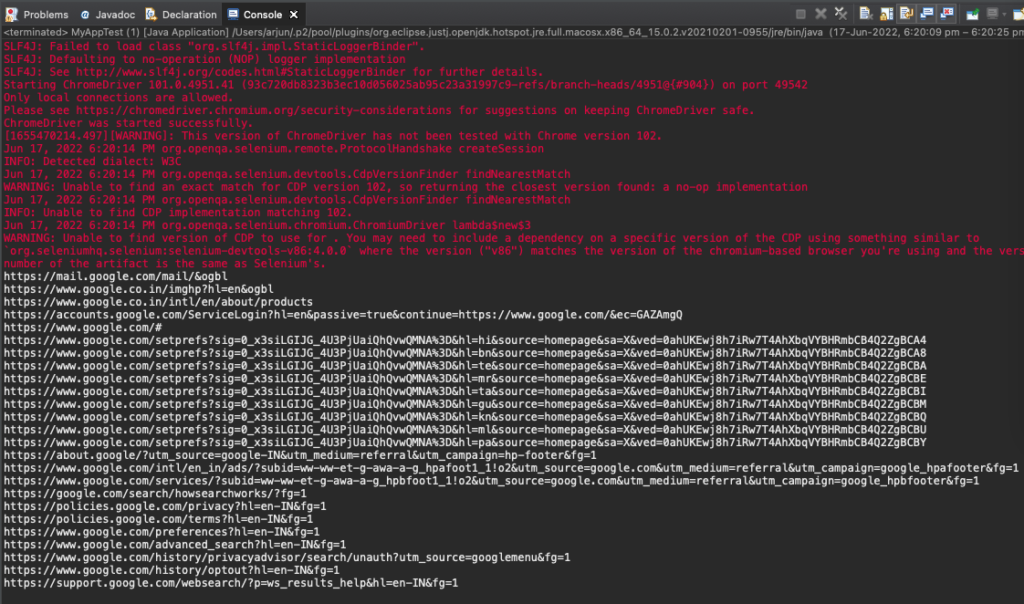