Typing Enter/Return Key in Selenium
To send a keyboard stroke of Enter/Return key programmatically using Selenium in Java, use WebDriver.sendKeys()
method and pass the value of Keys.RETURN
as argument.
WebDriver.sendKeys()
takes string or the keys to send to the element, as argument, and simulates typing these keys into the element.
The following is a simple code snippet where we find a web element in the page with the name q
and simulate typing the RETURN key into the web element.
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys(Keys.RETURN);
Example
In the following program, we write Java code to open Google home page, find the first web element with the name "q"
that is search box, enter a keyword "tutorialkart"
, and enter the RETURN key.
Java Program
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://google.com/ncr");
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("tutorialkart");
searchBox.sendKeys(Keys.RETURN);
driver.quit();
}
}
Screenshots
1. Initialize web driver and navigate to google.com.
WebDriver driver = new ChromeDriver();
driver.get("https://google.com/ncr");
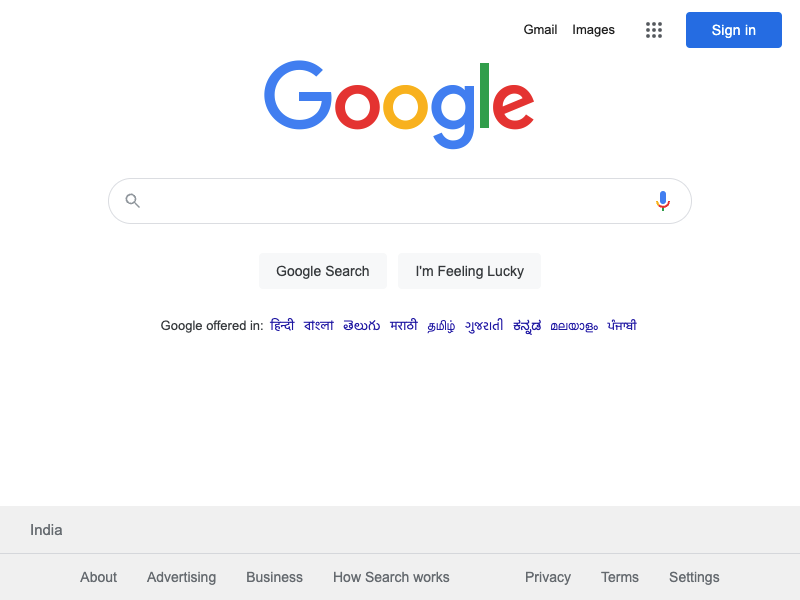
2. Enter search term “tutorialkart” in search box.
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("tutorialkart");
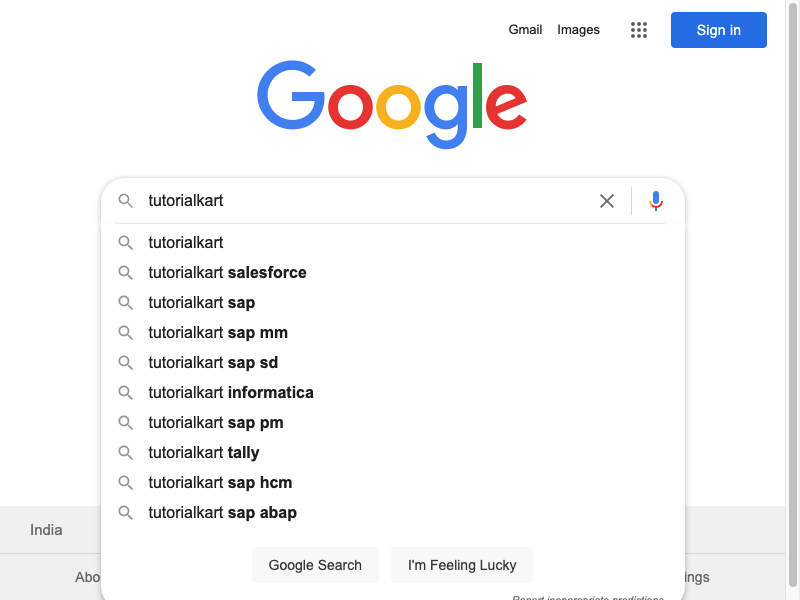
3. Enter RETURN key.
searchBox.sendKeys(Keys.RETURN);
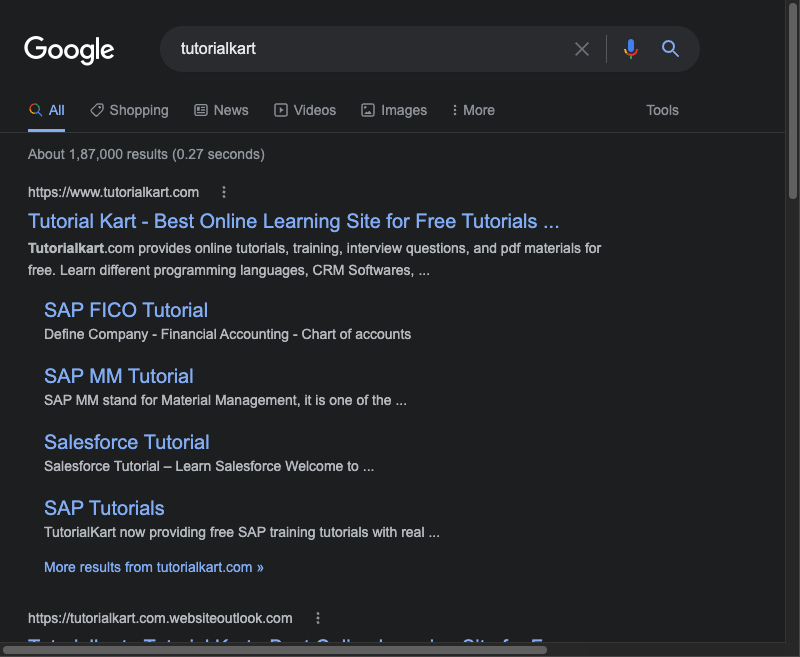