Find Element(s) by Tag Name
To find the element(s) by tag name using Selenium in Java, use WebDriver.findElement(By.tagName())
function or WebDriver.findElements(By.tagName())
.
The following is a simple code snippet to get the first h2
element
WebElement element = driver.findElement(By.tagName("h2"));
WebDriver.findElement(By.tagName("x"))
returns the first web element with the specified tag name "x"
.
The following is a simple code snippet to get all the h2
elements
List<WebElement> elements = driver.findElements(By.tagName("h2"));
WebDriver.findElements(By.tagName("x"))
returns the list of web elements with the specified tag name "x"
.
Example
Find the First Web Element with the Tag Name
In the following, we write Selenium Java program to visit WikiPedia Main Page, then find the first web element with the tag name "h2"
, meaning the first Heading 2 in the web page.
Java Program
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); WebElement element = driver.findElement(By.tagName("h2")); System.out.println(element.getText()); driver.quit(); } }
Screenshot
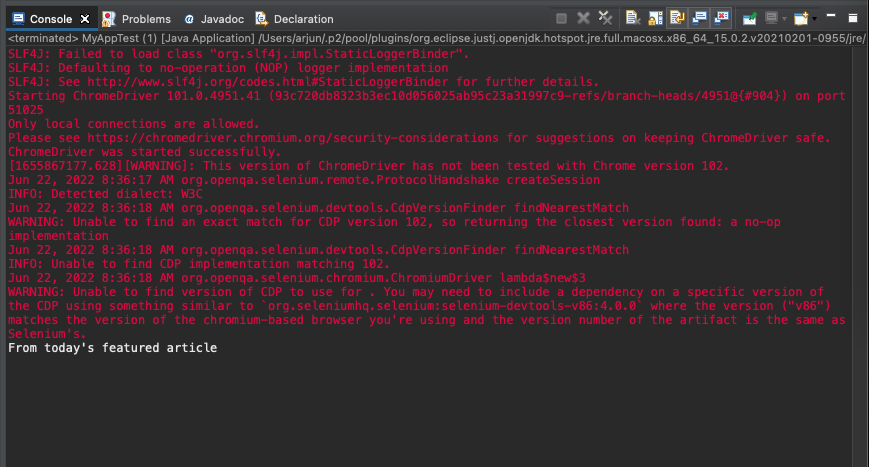
Find all Web Elements with the Tag Name
In the following, we write Selenium Java program to visit WikiPedia Main Page, then find all the web elements with the tag name "h2"
, meaning all the Heading 2.
Java Program
import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class MyAppTest { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://en.wikipedia.org/wiki/Main_Page"); List<WebElement> elements = driver.findElements(By.tagName("h2")); System.out.println("Number of elements with tag h2 : " + elements.size()); for (int i = 0; i < elements.size(); i++) { WebElement element = elements.get(i); System.out.println(i + " : " + element.getText()); } driver.quit(); } }
Screenshot
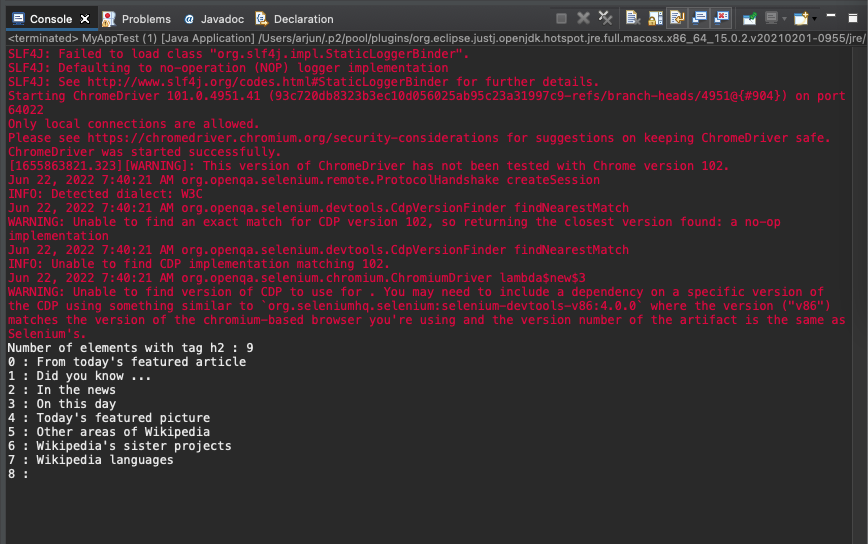