Get Parent Element
To get the parent element of given web element using Selenium for Java, find element on the given web element by XPath with the XPath expression as ".."
.
The following is a simple code snippet to get the parent element of the web element child
.
</>
Copy
child.findElement(By.xpath("parent::*"))
This expression returns the parent WebElement of child
.
Example
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, and find the parent element of the link element <a href="/java/">Java</a>
. We shall print the outer HTML attribute of both the child and parent to standard output.
Java Program
</>
Copy
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://www.tutorialkart.com/");
//child element
WebElement child = driver.findElement(By.xpath("//a[@href=\"/java/\"]"));
System.out.println("Child\n" + child.getAttribute("outerHTML"));
//get parent element
WebElement parent = child.findElement(By.xpath(".."));
System.out.println("\n\nParent\n" + parent.getAttribute("outerHTML"));
driver.quit();
}
}
Output
Child
<a href="/java/">Java</a>
Parent
<div class="s">
<a href="/java/">Java</a>
<a href="/cpp/">C++</a>
<a href="/c-sharp-tutorial/">C#</a>
<a href="/r-tutorial/">R</a>
<a href="/python/">Python</a>
<a href="/swift-tutorial/">Swift</a>
<a href="/kotlin-tutorial/">Kotlin</a>
<a href="/kotlin-android-tutorial/"><span>Kotlin Android</span></a>
<a href="/android-jetpack-compose/"><span>Android Jetpack Compose</span></a>
<a href="/dart/">Dart</a>
<a href="/flutter/">Flutter</a>
<a href="/nodejs/nodejs-tutorial/">NodeJS</a>
<a href="/fasttext-tutorial/">FastText</a>
<a href="/docker/docker-tutorial/">Docker</a>
<a href="/nginx/nginx-tutorial/">NGINX</a>
<a href="/kubernetes/kubernetes-tutorial/">Kubernetes</a>
<a href="/bash-shell-scripting/bash-tutorial/"><span>Bash Scripting</span></a>
<a href="/scipy-tutorial/">SciPy</a>
<a href="/git/">Git</a>
<a href="/opencv/python/"><span>OpenCV Python</span></a>
<a href="/javafx/">JavaFX</a>
<a href="/uml-tutorial/">UML</a>
<a href="/typescript/">TypeScript</a>
<a href="/scala/">Scala</a>
<a href="/julia/">Julia</a>
<a href="/numpy-tutorial/">Numpy</a>
<a href="/golang-tutorial/">Golang</a>
</div>
Screenshot
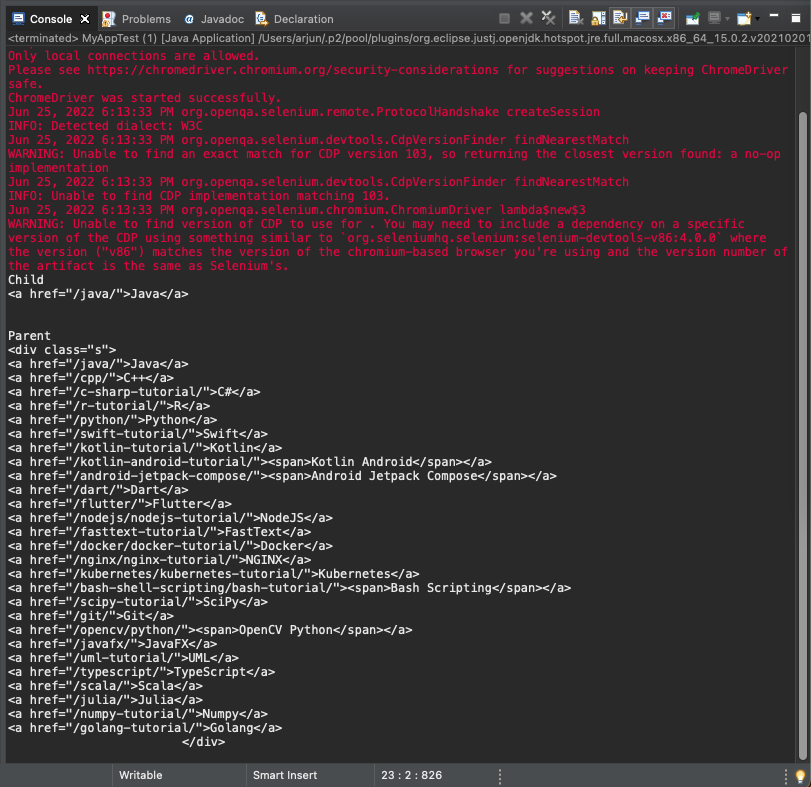