Set Specific Window Height
To set specific window height to the browser using Selenium for Java, call driver.manage().window().setSize()
method and pass the Dimension object as argument. The Dimension object can be initialized with required width and height. Height is set to the required value, and width is set to the existing width value.
The following is a simple code snippet to set the window size to a height of 800 and keeping the original width.
</>
Copy
int width = 800;
int height = driver.manage().window().getSize().height;
driver.manage().window().setSize(new Dimension(width, height));
Example
In the following program, we write Selenium Java code to set the window size to a width of 800.
Java Program
</>
Copy
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
//initialize web driver
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
try {
//get the URL
driver.get("https://www.tutorialkart.com/");
//set new dimension to browser window
int width = driver.manage().window().getSize().width;
int height = 800;
driver.manage().window().setSize(new Dimension(width, height));
} finally {
driver.quit();
}
}
}
Screenshots
1. Screenshot before resize.
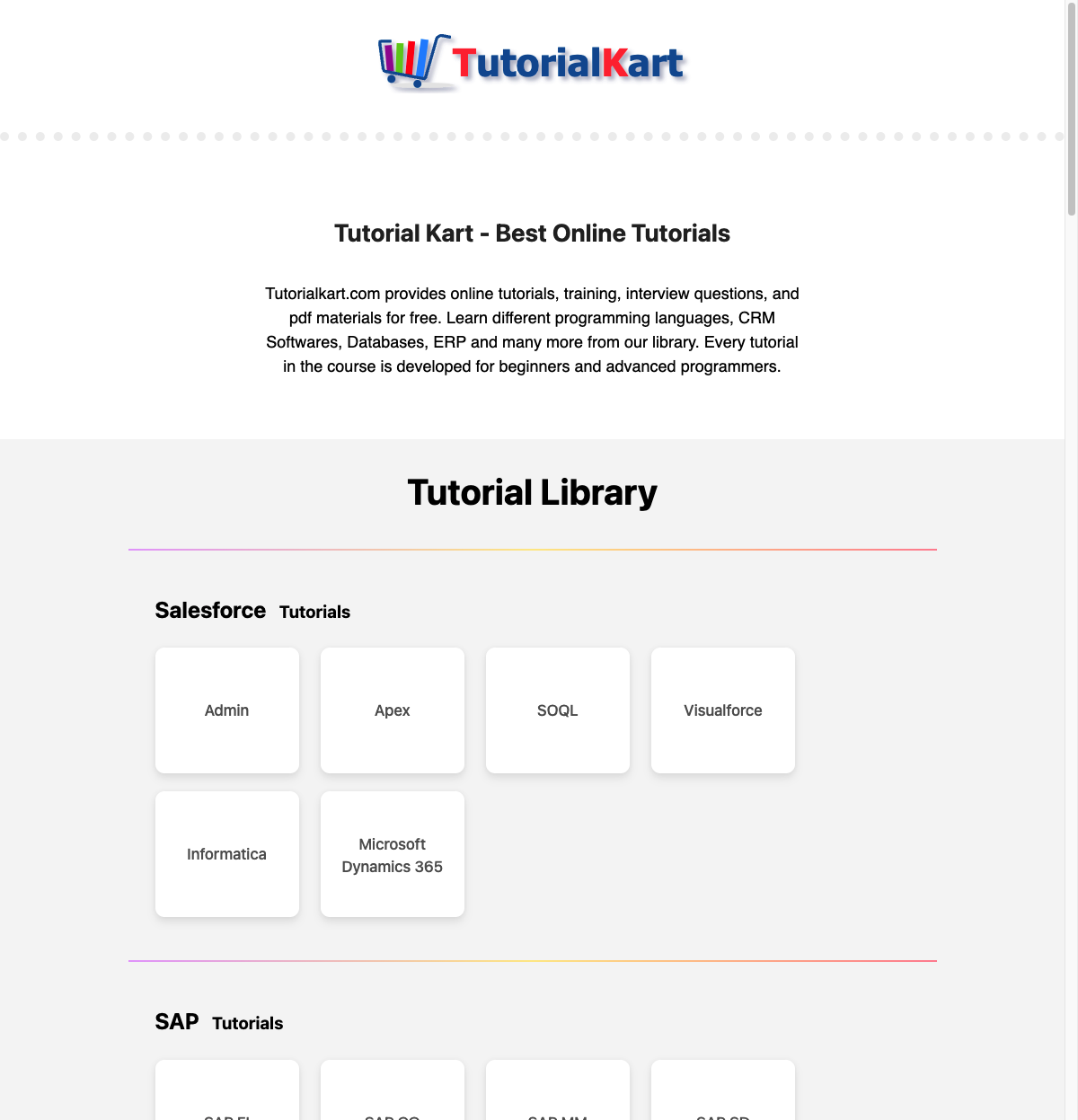
2. Screenshot after resize.
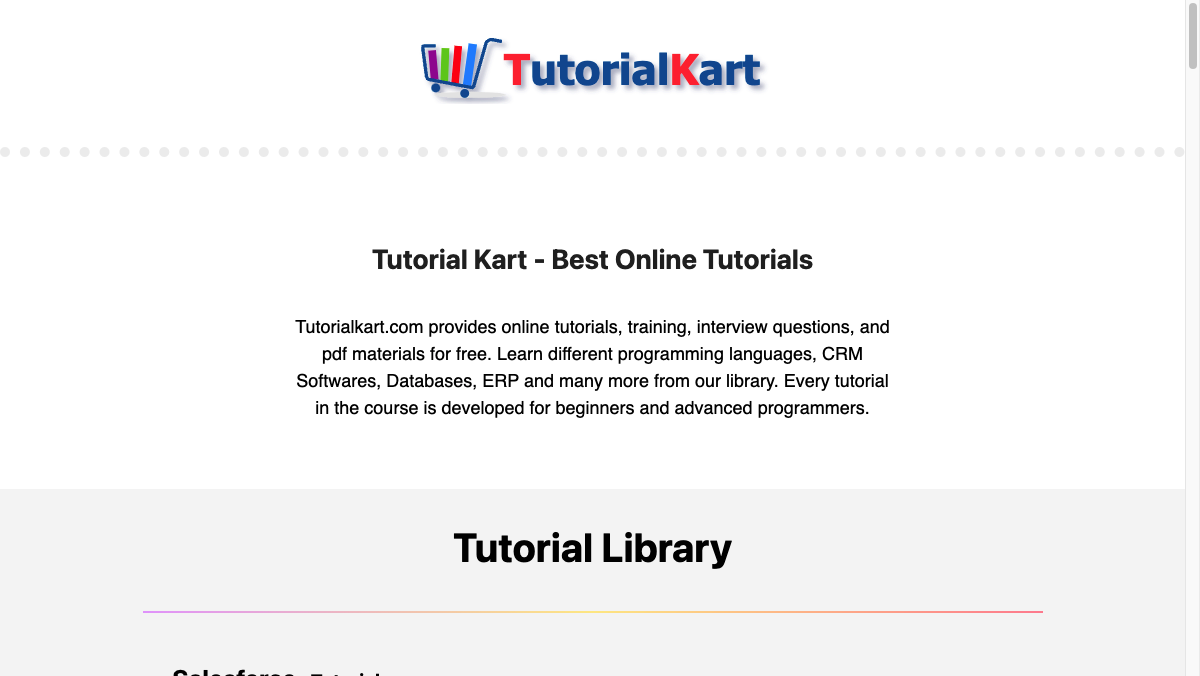