Matplotlib – Multiple Graphs on same Plot
To draw multiple graphs on same plot in Matplotlib, call plot() function on matplotlib.pyplot, and pass the x-y values of all the graphs one after another.
The syntax to call plot() function to draw multiple graphs on the same plot is
</>
Copy
plot([x1], y1, [fmt], [x2], y2, [fmt], ...)
where [x1], y1 will draw a graph, and [x2], y2 will draw another graph.
Example
In this example, we will draw three graphs, on the same plot. The first is a sine graph in red color, the second is a cosine graph in dashed blue color and the third graph is circular points in green color.
example.py
</>
Copy
import matplotlib.pyplot as plt
import numpy as np
#first graph
x1 = np.arange(0.0, 2.0, 0.01)
y1 = 1 + np.sin(2 * np.pi * x1)
#second graph
x2 = np.arange(0.0, 2.0, 0.01)
y2 = 1 + np.cos(np.pi * x2)
# third graph
x3 = [0.0, 0.1, 0.2, 0.6, 1.4, 1.5, 1.6, 1.9]
y3 = [0.5, 0.7, 0.8, 1.1, 1.4, 1.9, 0.6, 0.3]
plt.plot(x1, y1, 'r', x2, y2, '--b', x3, y3, 'go')
plt.show()
Output
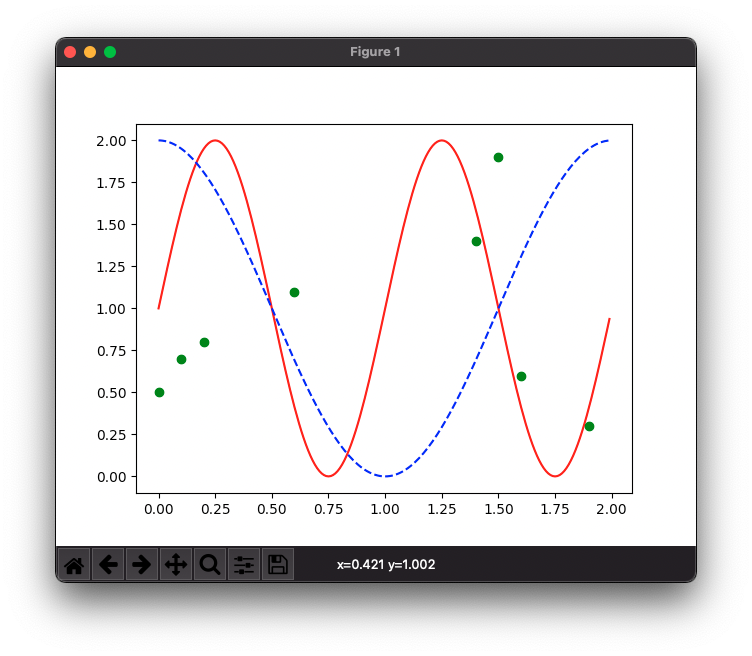
Conclusion
Concluding this Matplotlib Tutorial, we learned how to draw multiple graphs on a single plot in Matplotlib using plot() function.