Matplotlib PyPlot – Set Edge Color for Bar Plot
To set edge color for bars in a Bar Plot using Matplotlib PyPlot API, call matplotlib.pyplot.bar()
function, and pass required color value(s) to edgecolor
parameter of bar()
function.
The definition of matplotlib.pyplot.bar() function with edgecolor
parameter is
bar(x, height, edgecolor=None)
Of course, there are other named parameters, but for simplicity, only edgecolor
parameter is given along with required x
and height
.
where
Parameter | Description |
---|---|
edgecolor | The color(s) for bar edges. The parameter takes a color or list of colors. |
If a color value is given for edgecolor, then all the bars will get the same color for their edges.
Note: If we are providing a list of colors for edgecolor parameter, the length of this list of colors should match the length of x
and height
.
Example
In the following program, we will draw a bar plot with bars having red
as edge color.
example.py
import matplotlib.pyplot as plt
#data
x = [1, 2, 3, 4, 5]
h = [10, 8, 12, 4, 7]
#bar plot
plt.bar(x, height = h, fill = False, edgecolor = 'red')
plt.show()
Output
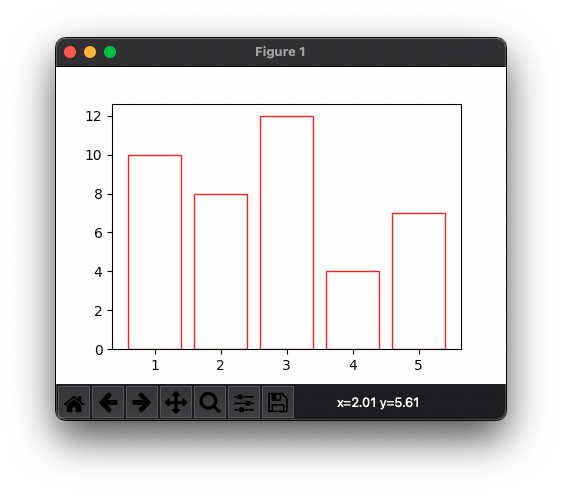
We can also give a hex color in RGB or RGBA format. In the following program, we will set the edge color of bar faces in the bar plot to the color represented by the hex color '#7eb54e'
.
example.py
import matplotlib.pyplot as plt
#data
x = [1, 2, 3, 4, 5]
h = [10, 8, 12, 4, 7]
#bar plot
plt.bar(x, height = h, fill = False, edgecolor = '#7eb54e')
plt.show()
Output
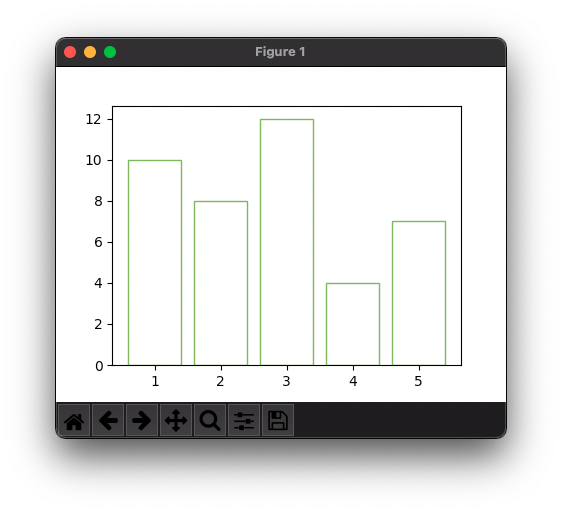
Now, let us apply different colors for bar edges of the bar plot, by passing a list of colors for edgecolor
parameter.
example.py
import matplotlib.pyplot as plt
#data
x = [1, 2, 3, 4, 5]
h = [10, 8, 12, 4, 7]
ec = ['red', 'gray', 'black', 'blue', 'orange']
#bar plot
plt.bar(x, height = h, fill = False, edgecolor = ec)
plt.show()
Output
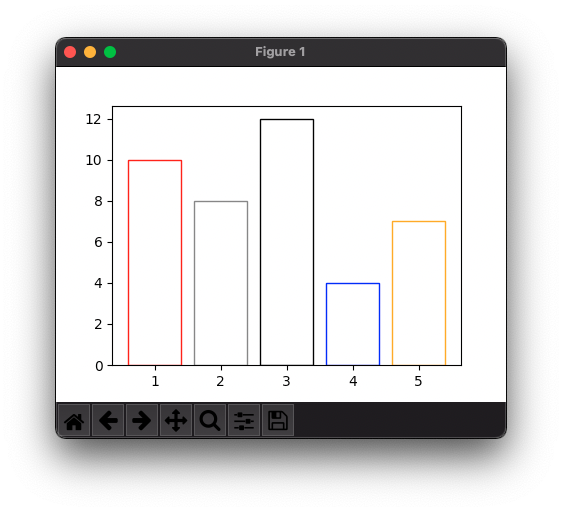
Conclusion
In this Matplotlib Tutorial, we learned how to set edge color(s) for bars in bar plot using Matplotlib PyPlot API.