Matplotlib Example
In this tutorial, we will write a basic Python program to display a plot using Matplotlib library.
In the following example, we will draw a simple line plot using X-axis and Y-axis data.
example.py
import matplotlib.pyplot as plt
# data for plotting
x = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
y = [5, 7, 8, 1, 4, 9, 6, 3, 5, 2, 1, 8]
plt.plot(x, y)
plt.xlabel('x-axis label')
plt.ylabel('y-axis label')
plt.title('Matplotlib Example')
plt.savefig("output.jpg")
Output
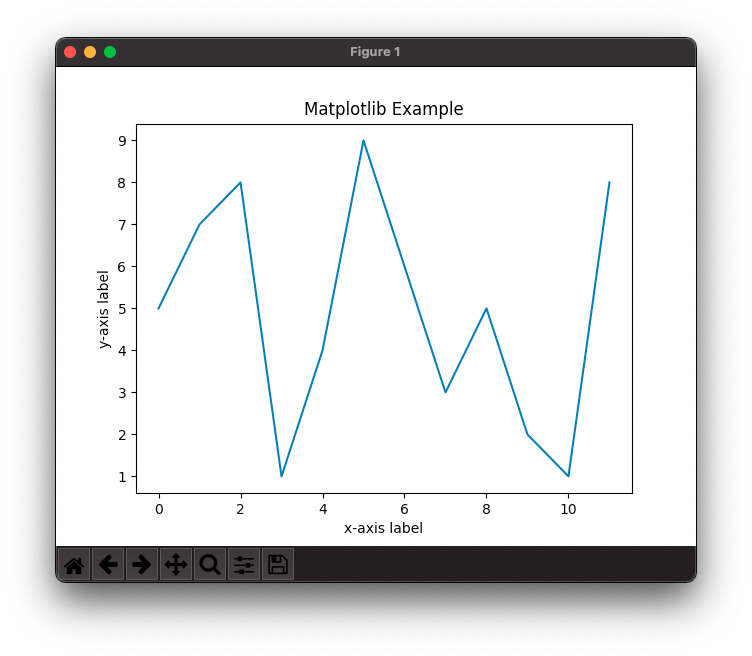
Let us understand each of the statements in the above program.
Import
Import matplotlib.pyplot.
import matplotlib.pyplot as plt
This is a state based interface to matplotlib. We can use this to draw plots.
Data
Get the data that we would like to plot.
x = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
y = [5, 7, 8, 1, 4, 9, 6, 3, 5, 2, 1, 8]
This is a state based interface to matplotlib. We can use this to draw plots.
Plot
Pass the x-axis and y-axis data to plot() function.
plt.plot(x, y)
Plot Title, X Label, Y Label
We may set the X axis label, Y axis label and Title to the plot by calling xlabel(), ylabel() and title() functions on pyplot, respectively.
plt.xlabel('x-axis label')
plt.ylabel('y-axis label')
plt.title('Matplotlib Example')
Show Plot
Finally, we can display the plot to user, in a window, using show() function.
plt.show()
Conclusion
Concluding this Matplotlib Tutorial, we learned how to write a draw a simple plot using Matplotlib library in Python.