Matplotlib – Set Line Width for Step Plot
To set specific line width for Step Plot in Matplotlib, call matplotlib.pyplot.step()
function, and pass required line width as float value for linewidth
parameter of step()
function.
The definition of matplotlib.pyplot.step() function with linewidth
parameter is
</>
Copy
step(x, y, linewidth=None)
Of course, there are other named parameters, but for simplicity, only linewidth
parameter is given along with required x
and y
. The default value is None
.
where
Parameter | Description |
---|---|
linewidth | The width of line in Step Plot. The parameter takes a float value. If 0, don’t draw steps. |
Example
In the following program, we will draw a Step Plot with some sample data, and 3.0
as line width.
example.py
</>
Copy
import matplotlib.pyplot as plt
# make data
x = [0, 1, 2, 3, 4, 5, 6, 7, 8]
y = [3, 4, 8, 2, 6, 5, 9, 4, 2]
#draw step plot
plt.step(x, y, linewidth=3.0)
#show plot as image
plt.show()
Output
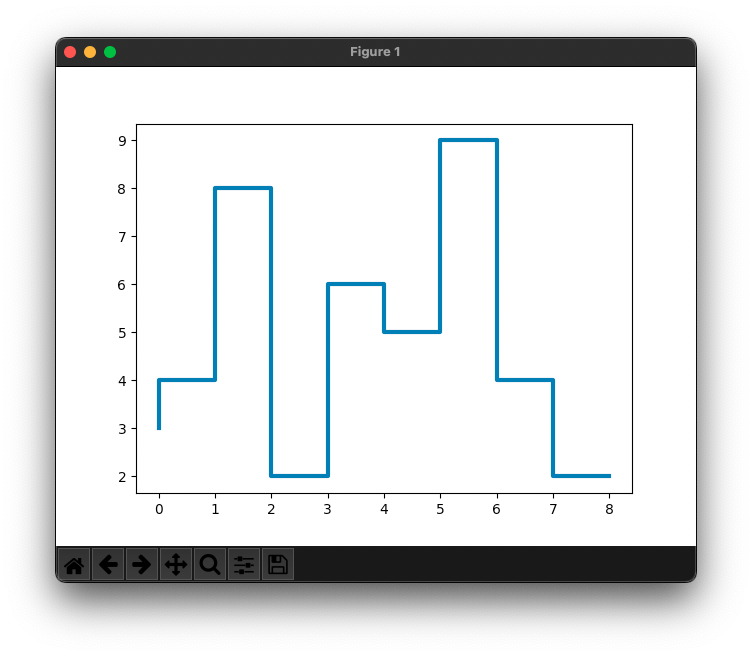
Now, let us take another value of 10.0 for line width and draw the Step Plot.
example.py
</>
Copy
import matplotlib.pyplot as plt
# make data
x = [0, 1, 2, 3, 4, 5, 6, 7, 8]
y = [3, 4, 8, 2, 6, 5, 9, 4, 2]
#draw step plot
plt.step(x, y, linewidth=3.0)
#show plot as image
plt.show()
Output
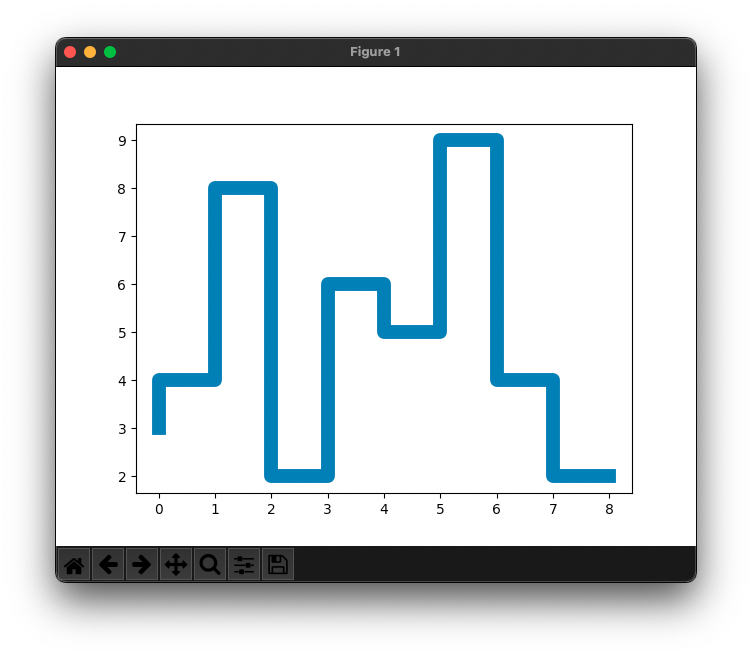
Conclusion
In this Matplotlib Tutorial, we learned how to set line width for Step Plot using Matplotlib PyPlot API.