Get CSS Value of Web Element
To get the value of specific CSS property for a specific web element using Selenium for Java, find the required web element, and call getCssValue()
method on the Web Element object with the css property name passed as String argument.
getCssValue(property)
method returns value of the CSS property for the Web Element.
The following is a simple code snippet to get the CSS property color for the Web Element element
.
</>
Copy
element.getCssValue("color");
Example
In the following program, we write Selenium Java code to visit Tutorialkart Home Page, get the Java tutorial link, and get its CSS color value.
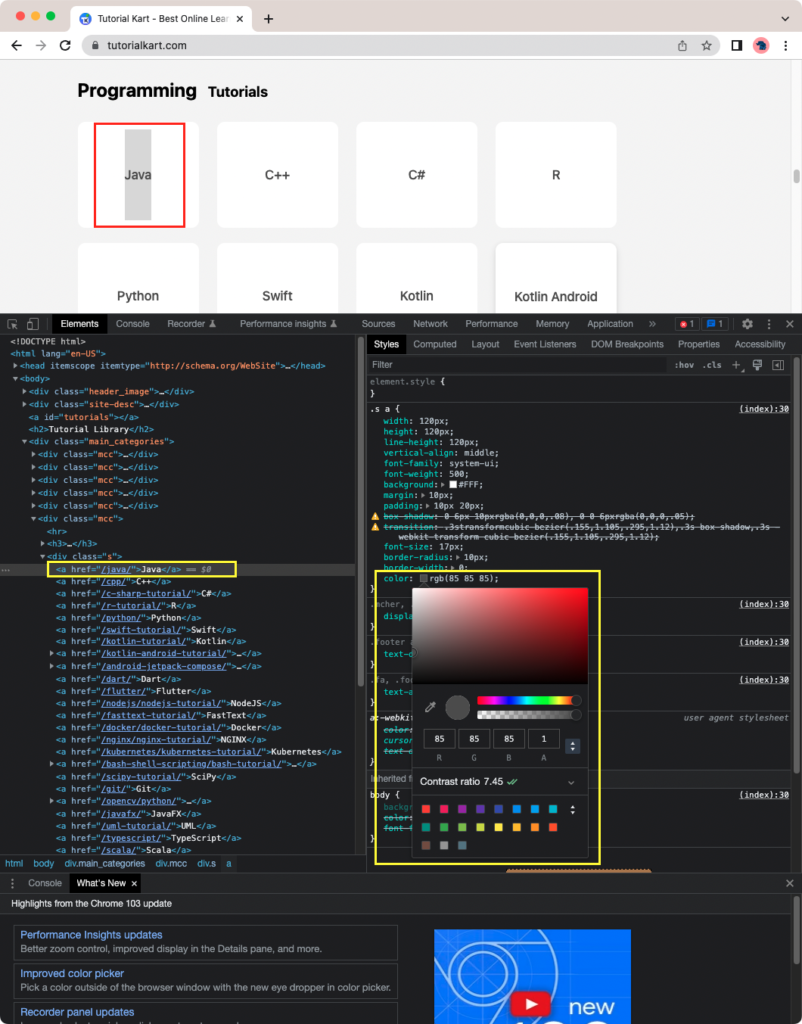
Java Program
</>
Copy
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
public class MyAppTest {
public static void main(String[] args) {
//initialize web driver
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
try {
//visit Tutorialkart Home Page
driver.get("https://www.tutorialkart.com/");
//click on Java link
WebElement tutorialLink = driver.findElement(By.xpath("//a[@href=\"/java/\"]"));
//get CSS color value
String color = tutorialLink.getCssValue("color");
System.out.println(color);
} finally {
driver.quit();
}
}
}
Output
rgba(85, 85, 85, 1)